


How to use PHP and swoole for high-performance message push service?
How to use PHP and swoole for high-performance message push service?
With the rapid development of the Internet, the need for real-time communication is becoming more and more urgent. Especially in some real-time interaction scenarios, such as chat rooms, instant messaging, online games and other applications, message push services have become an indispensable part. As one of the most widely used languages in Internet development, PHP also needs to find a high-performance message push solution. In this regard, swoole is an excellent choice.
swoole is a third-party extension based on PHP that can turn PHP into a Node.js-like environment. It provides asynchronous IO, coroutine, high-performance network communication and other functions, and can be used to build high-performance message push services. Below we will introduce in detail how to use PHP and swoole to implement a high-performance message push service.
First, we need to install the swoole extension. It can be installed through the following command:
pecl install swoole
After the installation is complete, add the following configuration in the php.ini file:
extension=swoole.so
Next, we create an index.php file to write our Message push service code:
<?php // 创建WebSocket服务器 $server = new swoole_websocket_server("0.0.0.0", 9501); // 监听WebSocket连接打开事件 $server->on('open', function (swoole_websocket_server $server, $request) { echo "New WebSocket connection: fd={$request->fd} "; }); // 监听WebSocket消息事件 $server->on('message', function (swoole_websocket_server $server, $frame) { foreach ($server->connections as $fd) { // 向所有连接中的客户端推送消息 $server->push($fd, $frame->data); } }); // 监听WebSocket连接关闭事件 $server->on('close', function ($server, $fd) { echo "WebSocket connection close: fd={$fd} "; }); // 启动WebSocket服务器 $server->start(); ?>
The above code is a simple WebSocket server example. It creates a WebSocket server listening on the local port 9501 through the swoole_websocket_server class. Then three events are monitored through the on method: open, message and close. The open event is triggered when the WebSocket connection is established, the message event is triggered when a client message is received, and the close event is triggered when the WebSocket connection is closed. In the message event, we traverse all connections through foreach and push the received message to all clients.
After saving and running the above code, our WebSocket server has been started. Next, we can use the WebSocket client to connect and send messages.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>WebSocket Client</title> </head> <body> <script> var ws = new WebSocket("ws://localhost:9501"); // 监听WebSocket连接打开事件 ws.onopen = function (event) { console.log("WebSocket connection opened."); }; // 监听WebSocket消息事件 ws.onmessage = function (event) { console.log("Received message: " + event.data); }; // 监听WebSocket连接关闭事件 ws.onclose = function (event) { console.log("WebSocket connection closed."); }; // 发送消息 function sendMessage() { var message = document.getElementById('message').value; ws.send(message); } </script> <input type="text" id="message"> <button onclick="sendMessage()">发送消息</button> </body> </html>
The above code is a simple WebSocket client example. It creates a WebSocket connection through JavaScript's WebSocket object, and then listens to the events of connection opening, message reception, and connection closing through onopen, onmessage, and onclose respectively. In the sendMessage function, we get the message text in the input box and then send the message through the ws.send method.
After opening the above client page in the browser, you can see an input box and a send button on the page. When we enter a message in the input box and click the send button, the message will be sent to the server and pushed to all clients at the same time. After the client receives the message, it will print the message in the console.
Through the above examples, we can see that a simple message push service can be easily implemented using PHP and swoole. Swoole's high performance and asynchronous IO features allow it to handle a large number of concurrent connections and message push, making it very suitable for message push services in real-time communication scenarios.
To sum up, using PHP and swoole to perform a high-performance message push service only requires a few lines of code and is very easy to get started. Compared with the traditional synchronous blocking IO method, swoole's asynchronous IO method can enable the server to process more requests at the same time, greatly improving service performance and realizing real-time communication requirements. Therefore, PHP and swoole are an excellent choice for developers who need to build high-performance message push services.
Reference documentation:
- swoole official documentation: https://www.swoole.co.uk/
- PHP official documentation: https://www. php.net/
The above is the detailed content of How to use PHP and swoole for high-performance message push service?. For more information, please follow other related articles on the PHP Chinese website!
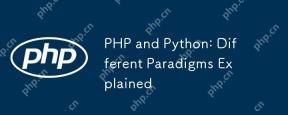
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
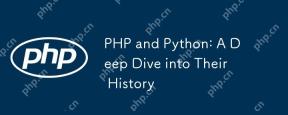
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
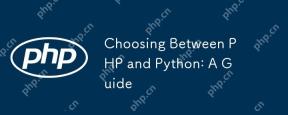
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
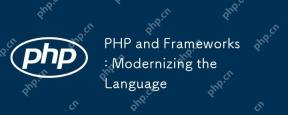
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
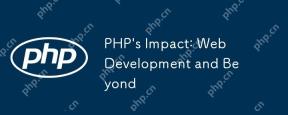
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
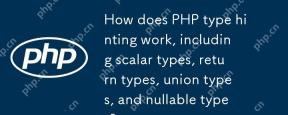
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
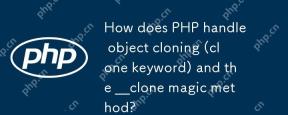
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
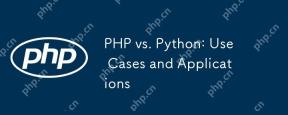
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
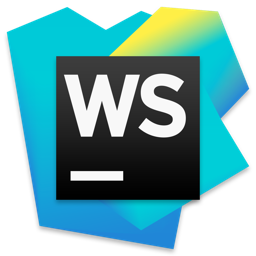
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor