


How to implement high-concurrency real-time video broadcast with PHP and swoole?
How do PHP and swoole implement high-concurrency real-time video live broadcast?
Introduction:
With the development of the Internet, live video has become an important part of people's daily lives. For enthusiasts, building a high-concurrency real-time video live broadcast platform is a very challenging task. This article will introduce how to use PHP and swoole extensions to achieve high-concurrency real-time video broadcast, and provide corresponding code examples.
1. Understanding PHP and swoole extension
1.1 PHP
PHP is a scripting language widely used in developing web applications. It has great compatibility and scalability, making it popular in the field of web development.
1.2 swoole extension
swoole extension is a coroutine network communication engine based on PHP. It provides a series of low-level APIs that allow PHP to handle concurrent requests like other high-performance languages. By leveraging the swoole extension, PHP can achieve higher concurrency performance.
2. Real-time video live broadcast
2.1 Basic idea
The basic idea of real-time video live broadcast is to transmit the video data stream to the client through the network and display it in real time. In this process, it is necessary to build a server to receive and distribute video streams, and write corresponding client code to realize video playback and display.
2.2 Build the server
First, you need to build a server to receive and process the video stream. By using swoole extension, asynchronous and non-blocking network communication can be easily achieved.
The following is a simple server example code:
// 实例化一个swoole WebSocket服务器对象 $server = new swoole_websocket_server("0.0.0.0", 9501); // 处理连接事件 $server->on('open', function (swoole_websocket_server $server, $request) { echo "new connection open: {$request->fd} "; }); // 处理消息事件 $server->on('message', function (swoole_websocket_server $server, $frame) { echo "received message: {$frame->data} "; // 将接收到的消息广播给所有连接的客户端 foreach ($server->connections as $fd) { $server->push($fd, $frame->data); } }); // 启动服务器 $server->start();
In the above code, the server listens to the open
and message
events of WebSocket. When a new client successfully connects, the open
event will be triggered; when a client sends a message, the message
event will be triggered. The server broadcasts received messages to all clients.
2.3 Writing the client
For real-time video broadcast, the function of the client is to receive the video stream from the server and play it in real time.
The following is a simple client code example:
<!DOCTYPE html> <html> <head> <title>视频直播</title> </head> <body> <video id="my-video" width="800" height="600" controls autoplay></video> <script> // 创建 WebSocket 连接 var socket = new WebSocket("ws://127.0.0.1:9501"); // 处理接收到的消息 socket.onmessage = function (event) { var video = document.getElementById("my-video"); var blob = event.data; var videoURL = URL.createObjectURL(blob); video.src = videoURL; }; // 处理关闭事件 socket.onclose = function (event) { console.log("WebSocket closed"); }; </script> </body> </html>
In the above code, a WebSocket connection is first created and connected to the server. After that, through the onmessage
event handling function, the video stream from the server is received and played to the client's video tag in real time.
3. Summary
Through PHP and swoole extensions, we can easily achieve high-concurrency real-time video live broadcast. Build a server to receive and distribute video streams, and write corresponding client code to realize video playback and display. The asynchronous and non-blocking features of the swoole extension enable PHP to handle high concurrency. I believe that through the introduction and sample code of this article, you already have a certain understanding of how to implement high-concurrency real-time video broadcast. Hope it helps.
The above is the detailed content of How to implement high-concurrency real-time video broadcast with PHP and swoole?. For more information, please follow other related articles on the PHP Chinese website!
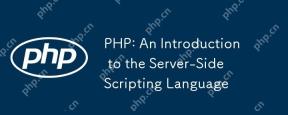
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
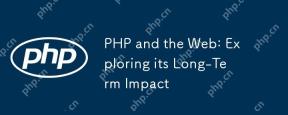
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
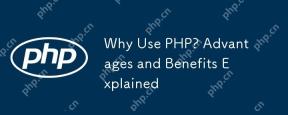
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
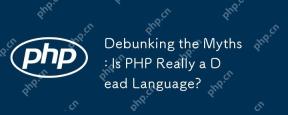
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
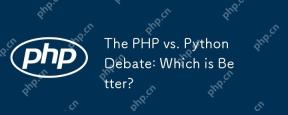
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
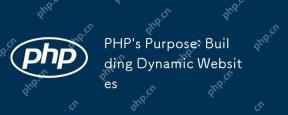
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
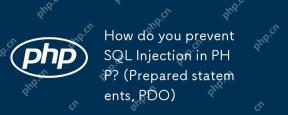
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
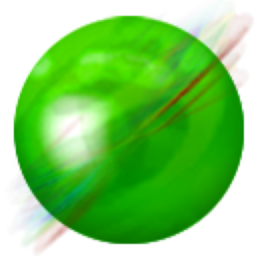
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor