How to use context to implement request interceptor in Go
Introduction:
In the actual development process, we often need to intercept and process requests. For the Go language, you can use the context package to implement request interception and middleware processing. This article will introduce how to use the context package to implement a request interceptor and provide corresponding code examples.
1. What is context package?
The context package is a package in the Go language used to pass the context of the request. After a request is initiated, the context package can pass some request-related information, such as the deadline of the request, the intermediate results of the request, etc. At the same time, the context package can also implement functions such as request cancellation and timeout.
2. Use the context package to implement the request interceptor
In the Go language, you can use the context package to implement the request interceptor. Interceptors can be used to implement some common functions, such as permission verification, request logging, etc. Below we will use an example to demonstrate how to use the context package to implement a request interceptor.
First, we create an intercepted function handleRequest, which is used to process the user's request:
func handleRequest(ctx context.Context) { // 处理请求 fmt.Println("处理请求") }
Next, we create an interceptor function interceptor, which is used to intercept the request , and do some additional processing before request processing:
func interceptor(ctx context.Context) context.Context { // 在请求处理之前执行拦截器的逻辑 fmt.Println("执行拦截器逻辑") // 返回新的上下文 newContext := context.WithValue(ctx, "token", "123456") return newContext }
In the above code, we first print the message that executes the interceptor logic, and pass a value named "token" through the WithValue method of the context package is added to the context and the new context is returned as the return value.
Next, we can call the interceptor function to intercept the request of the handleRequest function:
func main() { // 创建一个空白的上下文 ctx := context.Background() // 调用interceptor函数来拦截handleRequest函数的请求 newCtx := interceptor(ctx) // 调用handleRequest函数来处理请求 handleRequest(newCtx) }
In the above code, we first create a blank context object ctx, and use the interceptor function to intercept the request. The context object intercepts and generates a new context object newCtx. Then, we pass newCtx as a parameter to the handleRequest function to handle the request.
Finally, we can run the above code to see the effect. It can be found that before calling the handleRequest function, we will first print the message that executes the interceptor logic.
3. Conclusion
By using the context package, it becomes very simple to implement a request interceptor in the Go language. We can define an interceptor function and perform some additional processing on the request. In this way, we can implement some common functions, such as permission verification, request logging, etc.
The above is the entire content of this article. I hope it will be helpful for everyone to understand how to use the context package to implement a request interceptor. Thanks for reading!
Reference:
- Go Documentation: https://golang.org/pkg/context/
The above is the detailed content of How to use context to implement request interceptor in Go. For more information, please follow other related articles on the PHP Chinese website!
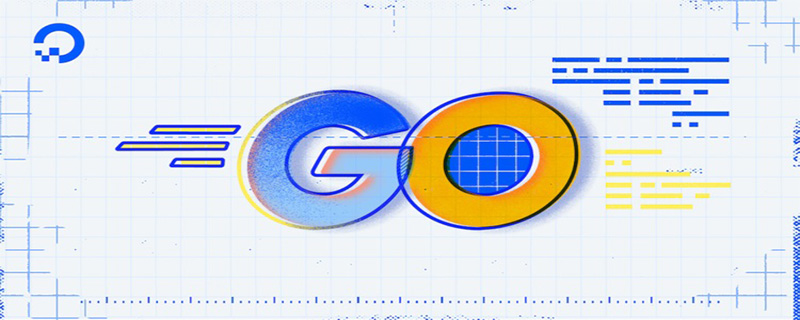
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
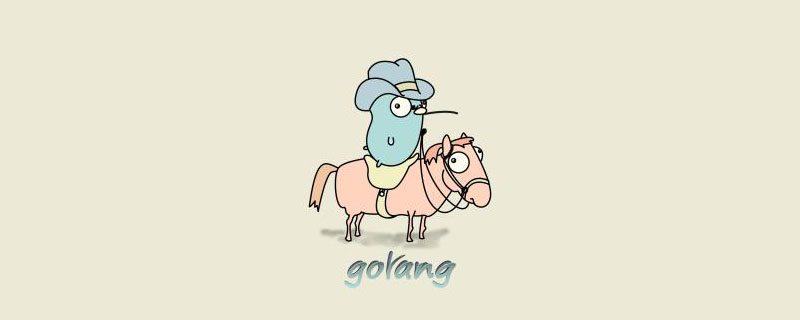
闭包(closure)是一个函数以及其捆绑的周边环境状态(lexical environment,词法环境)的引用的组合。 换而言之,闭包让开发者可以从内部函数访问外部函数的作用域。 闭包会随着函数的创建而被同时创建。
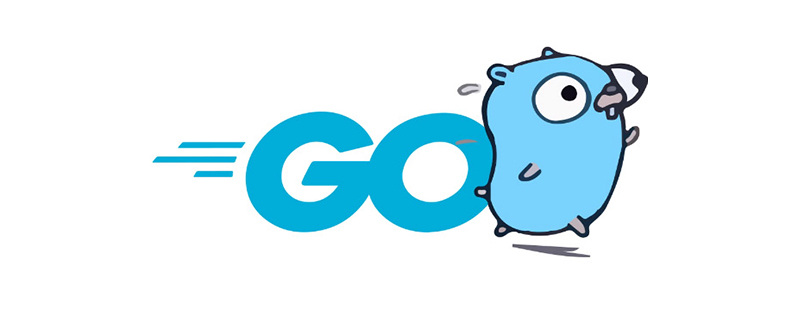
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
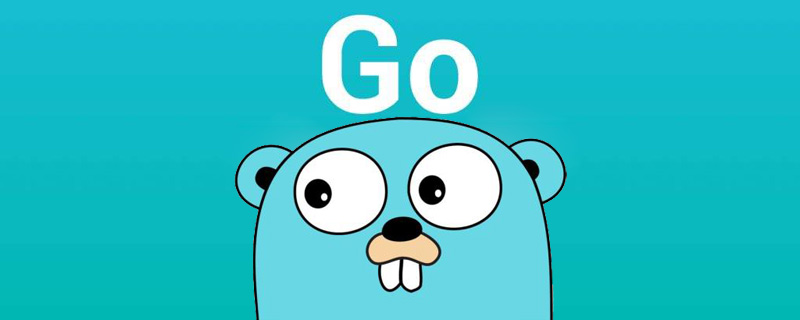
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
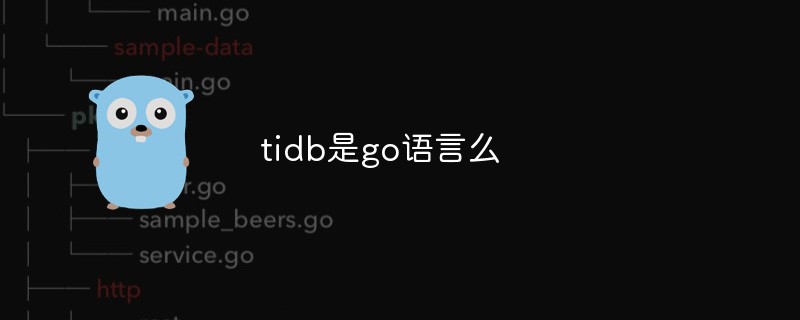
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
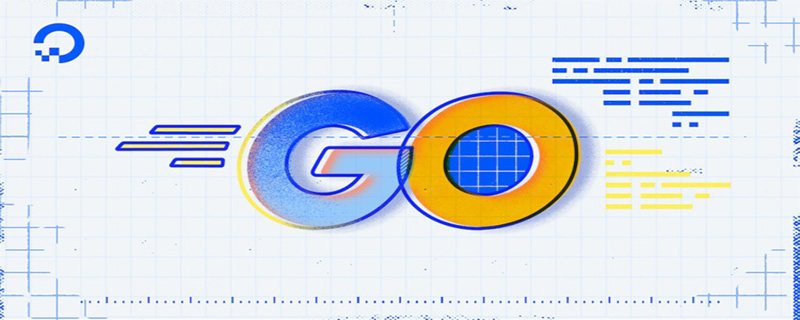
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
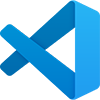
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
