


How to use PHP to call Kuaishou API interface to obtain and process user information
How to use PHP to call the Kuaishou API interface to obtain and process user information
As a popular short video social platform in China, Kuaishou has a huge user base and rich content resources. When developing related to Kuaishou, many developers will use Kuaishou's API interface to obtain and process user information. This article will introduce how to use PHP to call the Kuaishou API interface to quickly and accurately obtain user information and process it.
1. Apply for a Kuaishou developer account
Before using the Kuaishou API interface, you need to apply for a Kuaishou developer account and create an application to obtain the information required to access the API, including App ID and App Secret.
2. Obtain Access Token
In order to access Kuaishou’s API interface, you need to obtain the Access Token first. Access Token is a token used to verify the developer's identity and is generally valid for 30 days. There are two ways to obtain Access Token: one is to obtain it through user name and password, and the other is to obtain it through App ID and App Secret.
The following is a sample code to obtain Access Token through App ID and App Secret:
<?php $appId = "your_app_id"; $appSecret = "your_app_secret"; $tokenUrl = "https://open.kuaishou.com/oauth2/access_token"; $data = [ "app_id" => $appId, "app_secret" => $appSecret, "grant_type" => "client_credentials" ]; $options = [ CURLOPT_URL => $tokenUrl, CURLOPT_POST => true, CURLOPT_POSTFIELDS => http_build_query($data), CURLOPT_RETURNTRANSFER => true, ]; $curl = curl_init(); curl_setopt_array($curl, $options); $response = curl_exec($curl); curl_close($curl); $result = json_decode($response, true); $accessToken = $result['access_token']; ?>
3. Obtain user information
After obtaining Access Token, you can use it to obtain user information . By calling Kuaishou's user information interface, you can obtain the user's basic information, watch list, fan list, etc. The URL of the interface is as follows:
https://open.kuaishou.com/openapi/userinfo?access_token={access_token}&open_id={open_id}
Among them, {access_token} is the obtained Access Token, and {open_id} is the user's unique identifier.
The following is a sample code for obtaining user information:
<?php $openId = "user_open_id"; $userInfoUrl = "https://open.kuaishou.com/openapi/userinfo?access_token={$accessToken}&open_id={$openId}"; $options = [ CURLOPT_URL => $userInfoUrl, CURLOPT_RETURNTRANSFER => true, ]; $curl = curl_init(); curl_setopt_array($curl, $options); $response = curl_exec($curl); curl_close($curl); $userInfo = json_decode($response, true); ?>
4. Processing user information
After obtaining user information, it can be processed according to needs. For example, the user's nickname, avatar, number of fans and other information can be obtained and displayed or stored. The following is a simple example showing how to process user information:
<?php $nickname = $userInfo['user_nickname']; $avatar = $userInfo['user_avatar']; $followers = $userInfo['user_followers']; // 在这里可以进行进一步的处理,例如展示用户信息、保存用户信息到数据库等 ?>
Through the above steps, you can call Kuaishou's API interface in PHP to obtain user information and process it. In actual applications, different API interfaces can be called according to specific needs to achieve richer functions.
Summary
This article introduces how to use PHP to call the Kuaishou API interface to obtain and process user information. By applying for a Kuaishou developer account, obtaining Access Token, calling the user information interface and processing user information, developers can flexibly apply the API interface provided by Kuaishou in their own applications to achieve more interesting functions. I hope this article will be helpful to developers who are developing Kuaishou!
The above is the detailed content of How to use PHP to call Kuaishou API interface to obtain and process user information. For more information, please follow other related articles on the PHP Chinese website!
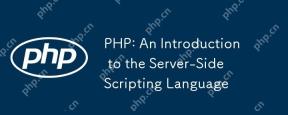
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
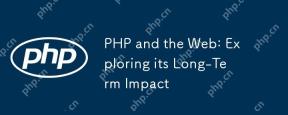
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
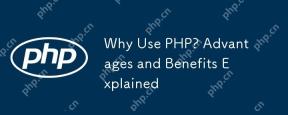
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
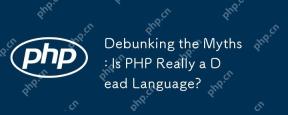
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
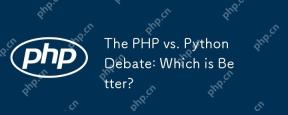
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
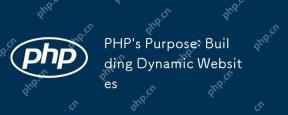
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
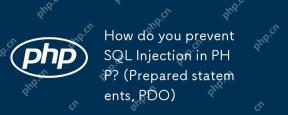
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!