How to implement object-oriented data structures in Go language
How to implement object-oriented data structures in Go language
Go language is a statically typed, compiled programming language. Compared with other programming languages, such as Java and C, Go language There are some differences in syntax and features. The Go language does not provide the concept of classes, but implements object-oriented features through structures and methods. In this article, we will explore how to implement object-oriented data structures in Go language.
First, let us understand the structure in Go language. A structure is a data type that packages fields of different types together. In Go language, we can use structures to represent and manipulate complex data structures. The following is an example of a simple structure:
type Rectangle struct { width float64 height float64 }
In the above code, we define a structure named Rectangle, which has two fields: width and height. Next, we can use the structure to create a rectangular object. For example:
rect := Rectangle{width: 10, height: 5}
With the structure, we can start to implement object-oriented features. First, we can manipulate the structure by defining methods. A method is a function associated with a structure. Methods are typically used to implement structure behavior and attribute access control. The following is an example of a structure and methods:
type Rectangle struct { width float64 height float64 } func (r Rectangle) Area() float64 { return r.width * r.height }
In the above code, we define a method named Area, which is used to calculate the area of a rectangle. Notice that there is a receiver in front of the method, which specifies the structure type to which the method belongs. In the Area method, we can access the fields of the structure through the receiver r.
Next, let’s take a look at how to implement encapsulation in the Go language. Encapsulation is an important concept in object-oriented programming that restricts direct access to data inside an object. In Go language, we can achieve encapsulation through the case of fields. Fields starting with a lowercase letter will be considered private and can only be accessed within the same package. Fields starting with a capital letter can be accessed in other packages. For example:
type Rectangle struct { width float64 height float64 }
In the above code, the width and height fields are public and can be accessed in other packages. If we change them to start with a lowercase letter, they will become private and can only be accessed within the current package.
Finally, let’s take a look at how to implement inheritance in the Go language. Inheritance is another important concept in object-oriented programming, which allows one object to inherit the properties and methods of another object. In Go language, we can use composition to implement inheritance. Here is an example:
type Shape interface { Area() float64 } type Rectangle struct { Shape width float64 height float64 } func (r Rectangle) Area() float64 { return r.width * r.height }
In the above code, we define an interface called Shape, which has an Area method. Next, we defined a structure named Rectangle, which has the Shape interface embedded. Through the embedded interface, the Rectangle structure can implement all methods of the Shape interface. In this way, we can assign the Rectangle type object to the Shape type variable and call the methods of the Shape interface.
Through the above code examples, we can see how to implement object-oriented data structures in Go language. Although the Go language does not provide the concept of classes, the combination of structures and methods allows us to implement object-oriented programming style. Mastering these concepts, we can use Go language more flexibly to design and implement complex data structures.
The above is the detailed content of How to implement object-oriented data structures in Go language. For more information, please follow other related articles on the PHP Chinese website!
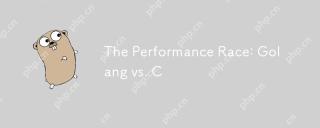
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
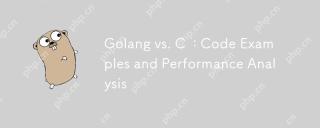
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
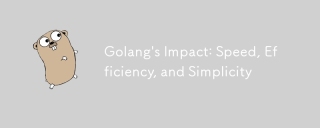
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
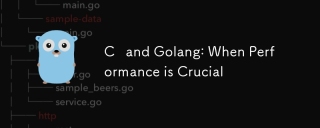
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
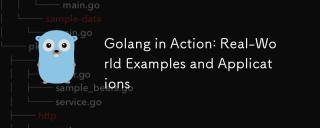
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
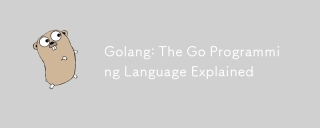
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
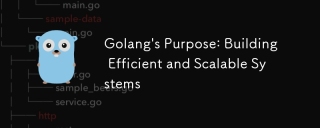
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
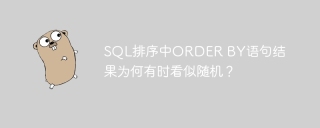
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
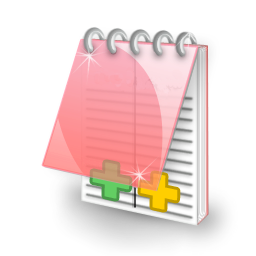
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
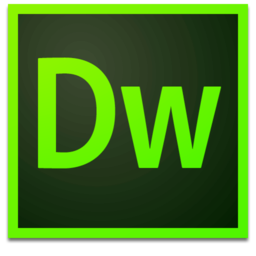
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor