How to implement advanced search functionality using PHP and Algolia
How to use PHP and Algolia to implement advanced search functionality
Introduction:
In web application development, search functionality is a very important part. As the amount of data increases and user needs continue to change, traditional search methods may not be able to meet user expectations. So, in this article, we will learn how to implement advanced search functionality using PHP and Algolia search engine.
Algolia Introduction:
Algolia is a powerful real-time search service provider. It provides a user-friendly API and rich functionality that allows you to easily integrate search engines into your applications. Algolia provides full-text search, fuzzy search, filtering, sorting and highlighting functions.
Step 1: Install Algolia’s PHP SDK
First, we need to install Algolia’s PHP SDK. You can use Composer to install the SDK, just create a composer.json file in the project root directory and add the following content:
{ "require": { "algolia/algoliasearch-client-php": "^2.0" } }
Then, run the following command to install the SDK:
composer install
Step 2: Create an Algolia account and create an index
Before using Algolia, you need to create an Algolia account. Once logged in, you can create an index to store search data. In the Algolia console, click the "Indices" tab, then click the "Add Index" button and follow the instructions to create an index.
Step 3: Add data to the index
Next, we need to add the data to the Algolia index. First, you need to set Algolia's configuration information in the PHP code:
require 'vendor/autoload.php'; $client = AlgoliaAlgoliaSearchSearchClient::create( 'YOUR_APP_ID', 'YOUR_API_KEY' ); $index = $client->initIndex('YOUR_INDEX_NAME');
Replace "YOUR_APP_ID" and "YOUR_API_KEY" with the relevant information for your Algolia account, and replace "YOUR_INDEX_NAME" with the information you created in step 2 The name of the index created in .
You can then add the data to the index using the following code:
$index->saveObject([ 'objectID' => '1', 'name' => 'John Doe', 'email' => 'john@example.com', 'age' => 30, 'city' => 'New York' ]); $index->saveObject([ 'objectID' => '2', 'name' => 'Jane Smith', 'email' => 'jane@example.com', 'age' => 25, 'city' => 'San Francisco' ]);
Please note that each saved object requires a unique "objectID" attribute.
Step 4: Implement Basic Search
Once the data is added to the index, we can start implementing basic search functionality. Here is a simple search function example:
function search($query) { require 'vendor/autoload.php'; $client = AlgoliaAlgoliaSearchSearchClient::create( 'YOUR_APP_ID', 'YOUR_API_KEY' ); $index = $client->initIndex('YOUR_INDEX_NAME'); $searchResults = $index->search($query); return $searchResults; }
Replace "YOUR_APP_ID" and "YOUR_API_KEY" with the Algolia account information obtained in step 3, and replace "YOUR_INDEX_NAME" with your index name.
Step 5: Implement advanced search
Algolia also provides some advanced search functions, such as filtering, sorting, and highlighting. Here is an example of an advanced search function:
function advancedSearch($query, $filters, $sortField, $sortOrder) { require 'vendor/autoload.php'; $client = AlgoliaAlgoliaSearchSearchClient::create( 'YOUR_APP_ID', 'YOUR_API_KEY' ); $index = $client->initIndex('YOUR_INDEX_NAME'); $searchParams = [ 'query' => $query, 'filters' => $filters, 'sort' => $sortField . ':' . $sortOrder ]; $searchResults = $index->search($searchParams); return $searchResults; }
Replace "YOUR_APP_ID" and "YOUR_API_KEY" with the Algolia account information obtained in step 3, and replace "YOUR_INDEX_NAME" with your index name.
The "$filters" parameter in the above function is a string used to filter search results. For example, "age > 25 AND city = 'New York'".
Conclusion:
In this article, we learned how to use PHP and the Algolia search engine to implement advanced search capabilities. By using the power of Algolia, we can easily build efficient, flexible and advanced search capabilities. Whether it's a small website or a large application, Algolia is a search solution worth considering.
The above is just a basic example. Algolia also provides many other features, such as customized search interface, search suggestions and search analysis. I hope this article has been helpful to you in implementing advanced search capabilities using PHP and Algolia.
The above is the detailed content of How to implement advanced search functionality using PHP and Algolia. For more information, please follow other related articles on the PHP Chinese website!

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
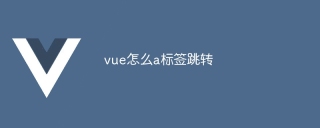
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
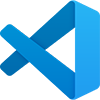
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
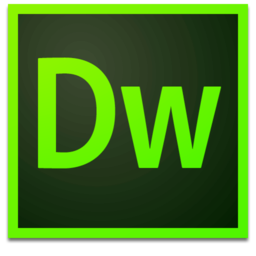
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor