


Tips for pairing Vue with Excel: How to implement dynamic filtering and sorting of data
Introduction:
In modern data processing, Excel is one of the most widely used tools. However, sometimes we need to integrate data from Excel into our applications and be able to dynamically filter and sort the data. This article will introduce techniques to use the Vue framework to achieve this requirement and provide code examples.
1. Import the Excel file
First, we need to import the Excel file and parse the data in it. This can be done through some libraries, such as xlsx
or xlsjs
. In Vue, you can introduce the Excel file in the mounted
life cycle hook and process the data in it. The following is a sample code:
<template> <div> <input type="file" ref="fileInput" @change="handleFileChange" /> </div> </template> <script> import XLSX from 'xlsx'; export default { methods: { handleFileChange(event) { const file = event.target.files[0]; const reader = new FileReader(); reader.onload = (event) => { const data = new Uint8Array(event.target.result); const workbook = XLSX.read(data, { type: 'array' }); const worksheet = workbook.Sheets[workbook.SheetNames[0]]; const jsonData = XLSX.utils.sheet_to_json(worksheet, {header: 1}); // 在这里处理Excel数据 // 将jsonData存储到Vue数据中,用于后续操作 }; reader.readAsArrayBuffer(file); } } } </script>
In the above code, we first introduced the xlsx
library, and then passed FileReader
in the handleFileChange
method The object reads the Excel file and parses it into JSON formatted data using the xlsx
library. Finally, we can save the parsed data in the data of the Vue instance for subsequent operations.
2. Dynamically filter data
Next, we can use Vue’s calculated properties and filters to implement the function of dynamically filtering data. The following is a sample code:
<template> <div> <input type="text" v-model="searchKeyword" placeholder="输入关键字过滤表格" /> <table> <thead> <tr> <th v-for="header in headers">{{ header }}</th> </tr> </thead> <tbody> <tr v-for="(row, index) in filteredData" :key="index"> <td v-for="cell in row">{{ cell }}</td> </tr> </tbody> </table> </div> </template> <script> export default { data() { return { data: [], // Excel数据 searchKeyword: '' // 过滤关键字 }; }, computed: { headers() { if (this.data.length > 0) { return this.data[0]; } return []; }, filteredData() { if (this.data.length > 0) { return this.data.filter(row => { return row.some(cell => cell.includes(this.searchKeyword)); }); } return []; } } } </script>
In the above code, we add an input box to the template for entering filter keywords. In the calculated attribute headers
, we return the first row of Excel data, that is, the header information. In the calculated property filteredData
, we use the filter
method to filter out the rows containing the filter keyword.
3. Dynamic sorting of data
In addition to filtering data, we may also need the function of sorting data. In Vue, you can use the sort
method of the array to implement sorting. The following is a sample code:
<template> <div> <table> <thead> <tr> <th v-for="header in headers"> {{ header }} <button @click="handleSort(header)">排序</button> </th> </tr> </thead> <tbody> <tr v-for="(row, index) in filteredData" :key="index"> <td v-for="cell in row">{{ cell }}</td> </tr> </tbody> </table> </div> </template> <script> export default { data() { return { data: [], // Excel数据 searchKeyword: '', // 过滤关键字 sortKey: '', // 排序关键字 sortOrder: '' // 排序顺序,'asc'为升序,'desc'为降序 }; }, computed: { headers() { if (this.data.length > 0) { return this.data[0]; } return []; }, filteredData() { if (this.data.length > 0) { return this.data.filter(row => { return row.some(cell => cell.includes(this.searchKeyword)); }).sort((a, b) => { if (this.sortKey !== '' && this.sortOrder !== '') { const indexA = this.headers.indexOf(this.sortKey); const indexB = this.headers.indexOf(this.sortKey); if (this.sortOrder === 'asc') { return a[indexA] > b[indexB] ? 1 : -1; } else if (this.sortOrder === 'desc') { return a[indexA] < b[indexB] ? 1 : -1; } } }); } return []; } }, methods: { handleSort(key) { if (this.sortKey === key) { this.sortOrder = this.sortOrder === 'asc' ? 'desc' : 'asc'; } else { this.sortKey = key; this.sortOrder = 'asc'; } } } } </script>
In the above code, we have added a button in each column of the table header to trigger the sorting method. In the handleSort
method, we determine whether the currently sorted column is consistent with the previous sorting column. If it is consistent, switch the sorting order; if it is inconsistent, set a new sorting column and set the sorting order to ascending order. . In the computed property filteredData
, we sort the data based on the sorting column and the sorting order.
Conclusion:
Through the above code examples, we can see how to use Vue to dynamically filter and sort Excel data. With Vue's computed properties and filters, we can easily implement these features and make our applications more flexible and efficient. Hope this article helps you!
The above is the detailed content of Tips for pairing Vue with Excel: How to implement dynamic filtering and sorting of data. For more information, please follow other related articles on the PHP Chinese website!
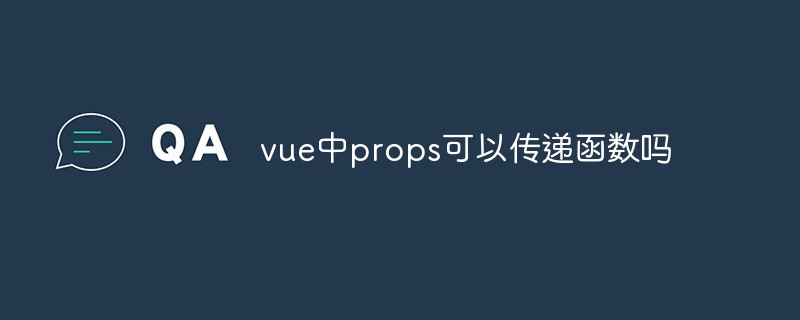
vue中props可以传递函数;vue中可以将字符串、数组、数字和对象作为props传递,props主要用于组件的传值,目的为了接收外面传过来的数据,语法为“export default {methods: {myFunction() {// ...}}};”。
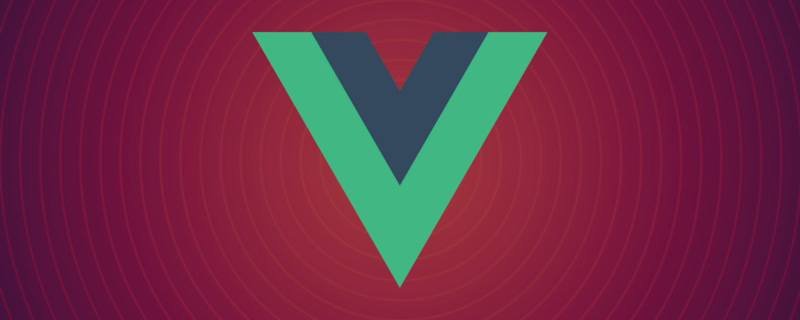
本篇文章带大家聊聊vue指令中的修饰符,对比一下vue中的指令修饰符和dom事件中的event对象,介绍一下常用的事件修饰符,希望对大家有所帮助!
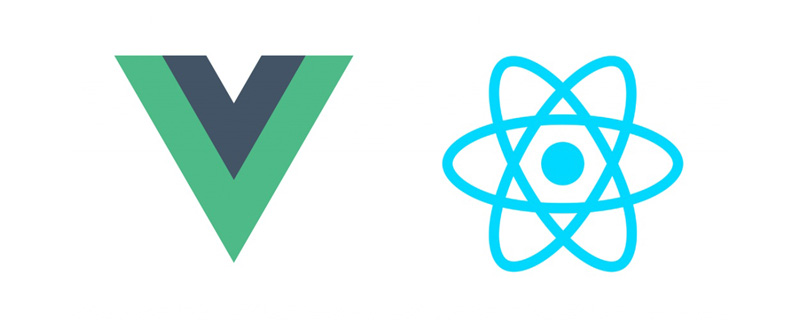
如何覆盖组件库样式?下面本篇文章给大家介绍一下React和Vue项目中优雅地覆盖组件库样式的方法,希望对大家有所帮助!
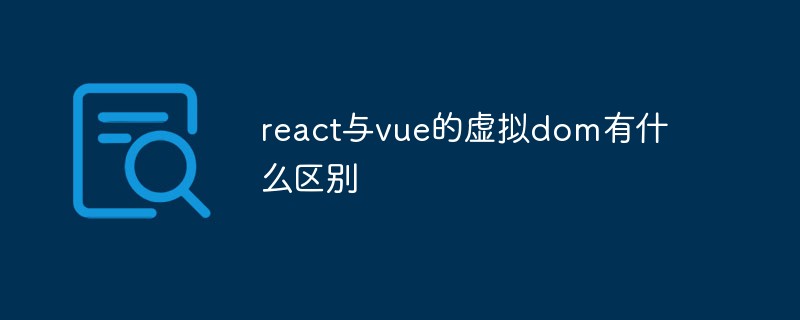
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
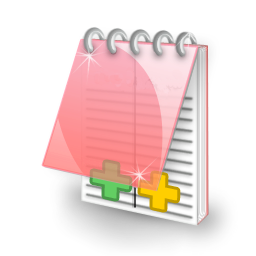
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
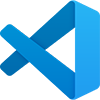
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
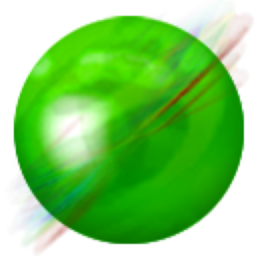
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
