How to use Vue and Excel to quickly generate interactive data reports
Introduction:
In the field of modern business and data analysis, data reporting is a very important tool. They help us better understand and analyze data, discover trends and patterns in data, and support business decisions. With the help of these two powerful tools, Vue and Excel, we can easily create interactive data reports, taking data visualization and presentation to a whole new level.
This article will introduce how to use Vue and Excel to quickly generate interactive data reports, and provide code examples for reference.
1. Preparation
First of all, we need to prepare the required tools and resources. Here is a list of necessary tools and resources:
- Vue Framework: Vue is a popular JavaScript framework for building user interfaces. You can download and install Vue from the official website (https://vuejs.org/).
- Excel file: As our data source, we need to prepare an Excel file and ensure that the data in the file is in the correct format.
2. Install necessary dependencies
Before using Vue and Excel, we need to install some necessary dependency libraries. Use the following command to install these dependent libraries:
npm install --save xlsx npm install --save file-saver
3. Read Excel data
In the code of the Vue project, we first need to read and parse the data in the Excel file. We can use the two libraries xlsx and file-saver to achieve this function. The following is a code example for reading Excel data:
import { read, utils } from 'xlsx'; import { saveAs } from 'file-saver'; // 读取Excel文件 const workbook = read(file, { type: 'binary' }); // 获取工作表 const sheetName = workbook.SheetNames[0]; const worksheet = workbook.Sheets[sheetName]; // 解析数据 const jsonData = utils.sheet_to_json(worksheet, { header: 1 });
4. Use Vue components to display data
In the Vue project, we can use Vue components to display data. We can first define a data reporting component and use charts, tables and other components to display data. The following is a simple Vue component example:
<template> <div> <!-- 使用图表展示数据 --> <chart :data="chartData"></chart> <!-- 使用表格展示数据 --> <table :data="tableData"></table> </div> </template> <script> export default { data() { return { chartData: [], // 图表数据 tableData: [] // 表格数据 }; } }; </script>
5. Data processing and visualization
In the Vue component, we can process and visualize data. We can use various chart libraries, table libraries, etc. to display data, such as Echarts, Highcharts, Element-ui, etc. The following is an example of using Echarts to display data:
import echarts from 'echarts'; export default { mounted() { // 初始化Echarts实例 const chart = echarts.init(this.$el); // 填入数据 chart.setOption({ xAxis: { type: 'category', data: this.chartData.labels }, yAxis: { type: 'value' }, series: [{ data: this.chartData.values, type: 'bar' }] }); } };
6. Export report
Finally, in the Vue project, we need to provide the function of exporting data reports. We can use the file-saver library to achieve this function. The following is an example of exporting a data report:
import { write } from 'xlsx'; export default { methods: { exportReport() { // 构造Excel数据 const worksheet = utils.json_to_sheet(this.tableData); const workbook = utils.book_new(); utils.book_append_sheet(workbook, worksheet, 'Report'); // 保存Excel文件 const excelData = write(workbook, { type: 'binary' }); const blob = new Blob([s2ab(excelData)], { type: 'application/octet-stream' }); saveAs(blob, 'Report.xlsx'); } } }; // 字符串转ArrayBuffer function s2ab(s) { const buf = new ArrayBuffer(s.length); const view = new Uint8Array(buf); for (let i = 0; i < s.length; i++) { view[i] = s.charCodeAt(i) & 0xFF; } return buf; }
7. Summary
By utilizing Vue and Excel, we can easily generate interactive data reports. In this article, we introduce the entire process from reading Excel data to displaying and exporting data reports, and provide code samples for readers' reference.
However, the above only introduces a basic example. In actual operation, more functional expansion and optimization can be carried out according to specific needs. I hope this article can provide readers with some help and guidance in using Vue and Excel to generate interactive data reports.
The above is the detailed content of How to quickly generate interactive data reports using Vue and Excel. For more information, please follow other related articles on the PHP Chinese website!
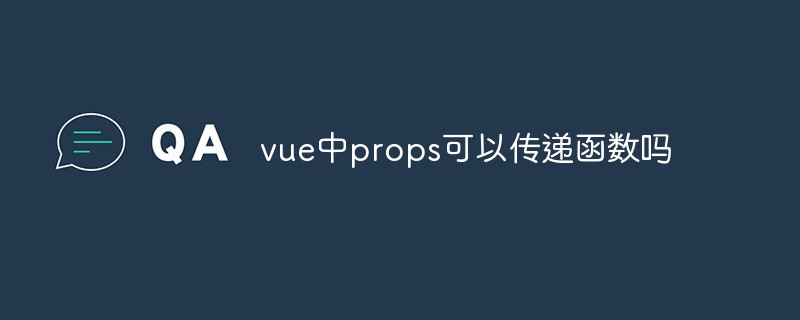
vue中props可以传递函数;vue中可以将字符串、数组、数字和对象作为props传递,props主要用于组件的传值,目的为了接收外面传过来的数据,语法为“export default {methods: {myFunction() {// ...}}};”。
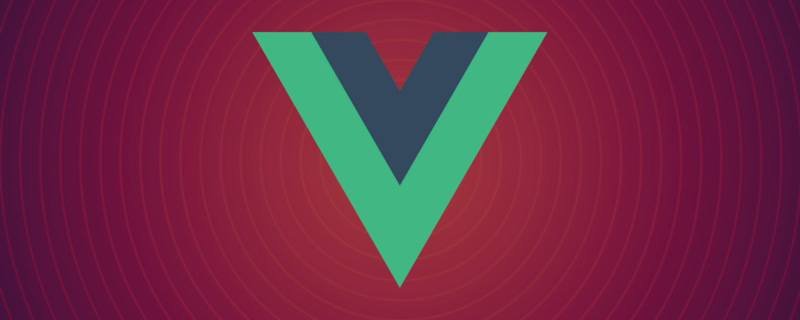
本篇文章带大家聊聊vue指令中的修饰符,对比一下vue中的指令修饰符和dom事件中的event对象,介绍一下常用的事件修饰符,希望对大家有所帮助!
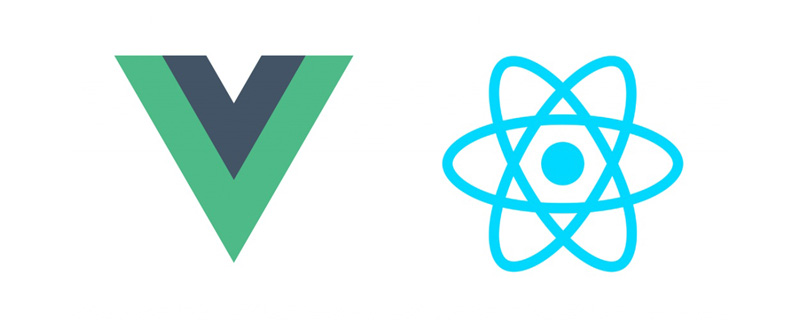
如何覆盖组件库样式?下面本篇文章给大家介绍一下React和Vue项目中优雅地覆盖组件库样式的方法,希望对大家有所帮助!
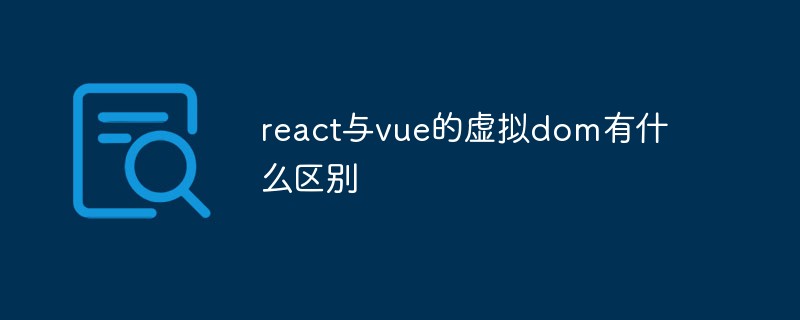
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
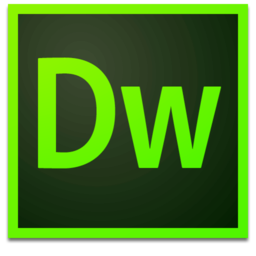
Dreamweaver Mac version
Visual web development tools
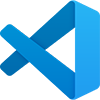
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
