


Request flow control configuration and practice of http.Transport in Go language
Request flow control configuration and practice of http.Transport in Go language
Introduction
In the current Internet environment, high concurrent requests are very common. In order to ensure system stability and good performance, we need to perform appropriate flow control on requests. In the Go language, http.Transport is a commonly used HTTP client library, which we can configure to achieve request flow control. This article will introduce how to configure http.Transport in Go language to implement request flow control, and combine it with code examples to help readers better understand and apply it.
- Understanding http.Transport
Before starting to configure http.Transport, we first need to understand its basic functions and principles. http.Transport is a client library for the HTTP transport layer in the Go language. It can send HTTP requests and process responses. By default, http.Transport does not have any flow control mechanism, that is, it sends all requests immediately. This may cause the server to be overloaded or even cause the service to crash under high concurrency conditions. Therefore, we need to configure http.Transport to implement request flow control. - Configuring http.Transport
The http.Transport structure in the Go language has several parameters related to request flow control. We can achieve flow control by setting these parameters.
a. MaxIdleConnsPerHost parameter
The MaxIdleConnsPerHost parameter indicates the maximum number of idle connections for each host. In the process of HTTP requests, in order to improve performance, connection pool technology is often used, that is, multiple requests share one connection. By setting the MaxIdleConnsPerHost parameter, we can limit the number of connections per host and thereby control the concurrency of requests.
b. MaxConnsPerHost parameter
The MaxConnsPerHost parameter indicates the maximum number of connections per host. Similar to MaxIdleConnsPerHost, by setting the MaxConnsPerHost parameter, we can limit the number of connections per host to control the amount of concurrent requests. The difference is that the MaxConnsPerHost parameter includes the number of active and idle connections, while the MaxIdleConnsPerHost parameter only includes the number of idle connections.
c. MaxIdleTime parameter
The MaxIdleTime parameter indicates the maximum idle time of each connection. By setting the MaxIdleTime parameter, we can control the connection to be closed after being idle for a period of time, thereby releasing resources.
- Practice example
The following is a simple code example that demonstrates how to configure http.Transport to implement request flow control.
package main import ( "fmt" "net/http" "time" ) func main() { // 创建一个HTTP客户端 client := &http.Client{ Transport: &http.Transport{ MaxIdleConnsPerHost: 10, // 每个主机的最大空闲连接数 MaxConnsPerHost: 20, // 每个主机的最大连接数 IdleConnTimeout: 30 * time.Second, // 连接的最大空闲时间 }, } // 发送100个请求 for i := 0; i < 100; i++ { go func(i int) { // 创建一个HTTP请求 req, err := http.NewRequest("GET", "https://example.com", nil) if err != nil { fmt.Println("创建请求失败:", err) return } // 发送请求并获取响应 resp, err := client.Do(req) if err != nil { fmt.Println("发送请求失败:", err) return } // 处理响应 // ... resp.Body.Close() fmt.Println("第", i+1, "个请求完成") }(i) } // 等待所有请求完成 time.Sleep(5 * time.Second) }
In the above code example, we create an http.Client object and configure http.Transport by setting the Transport field. We set the maximum number of idle connections per host to 10, the maximum number of connections to 20, and the maximum idle time of a connection to 30 seconds. Then, we send 100 requests through a loop and use goroutines to achieve concurrency. Finally, we wait for all requests to complete through the Sleep function.
Conclusion
By configuring http.Transport, we can control the request flow, thereby ensuring system stability and good performance. In practical applications, we can adjust parameter settings according to the specific needs of the system. Through flexible configuration, we can optimize the system's resource utilization and improve the system's concurrent processing capabilities.
The above is an introduction to the configuration and practice of request flow control of http.Transport in Go language. I hope this article can help readers better understand and apply this feature.
Reference link:
- Go HTTP client: https://golang.org/pkg/net/http/
- Go HTTP package examples: https:/ /golang.org/pkg/net/http/#pkg-examples
The above is the detailed content of Request flow control configuration and practice of http.Transport in Go language. For more information, please follow other related articles on the PHP Chinese website!
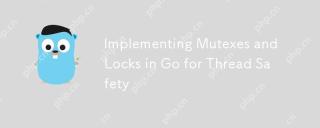
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
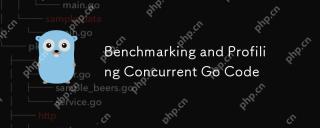
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
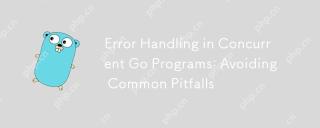
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
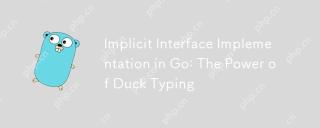
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
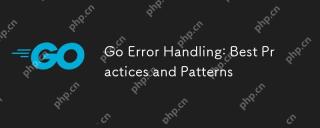
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
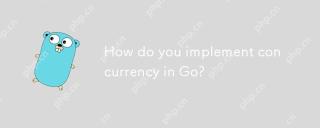
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
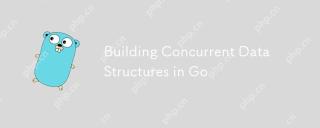
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
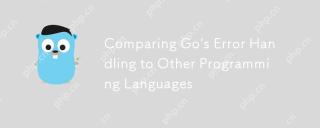
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
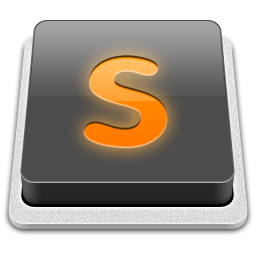
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
