How to use Vue to implement component communication?
How to use Vue to implement component communication?
Vue is a popular JavaScript framework for building user interfaces. In Vue, components are the basic units for building web applications. And communication between components is critical to building complex applications. This article will introduce how to use Vue to implement communication between components and provide some code examples.
1. Parent component communicates with child components
Communication between parent component and child component is the most common scenario. Vue provides props attributes to implement this communication. In the parent component, data can be passed to the child component through props, and the child component can directly use the data in the props.
The following is a simple example of a parent component communicating to a child component:
Parent component
<template> <div> <h1 id="父组件">父组件</h1> <ChildComponent :message="message"></ChildComponent> </div> </template> <script> import ChildComponent from './ChildComponent.vue'; export default { components: { ChildComponent }, data() { return { message: 'Hello World!' }; } }; </script>
Child component
<template> <div> <h2 id="子组件">子组件</h2> <p>{{ message }}</p> </div> </template> <script> export default { props: { message: { type: String, required: true } } }; </script>
In the above example, the parent A data message
is defined in the component, and the data is passed to the sub-component through the props
attribute. Use props
in the child component to receive the data passed by the parent component and render it in the template.
2. Communication from child components to parent components
Communication from child components to parent components is relatively complicated. Vue provides the $emit
method to implement communication between child components and parent components.
The following is a simple example of a child component communicating to a parent component:
Parent component
<template> <div> <h1 id="父组件">父组件</h1> <ChildComponent @message="handleMessage"></ChildComponent> </div> </template> <script> import ChildComponent from './ChildComponent.vue'; export default { components: { ChildComponent }, methods: { handleMessage(message) { console.log(message); } } }; </script>
Child component
<template> <div> <h2 id="子组件">子组件</h2> <button @click="sendMessage">发送消息</button> </div> </template> <script> export default { methods: { sendMessage() { this.$emit('message', 'Hello World!'); } } }; </script>
In the above example, the child The message
event is triggered in the component by using the $emit
method, and the data Hello World!
is passed to the parent component. The parent component uses @message
to listen to the message
event and handles the event in the handleMessage
method.
3. Non-parent-child component communication
If you need to communicate between non-parent-child components, you can use the event bus mechanism provided by Vue. You can create an event bus instance and then communicate between components through this instance.
The following is an example of using event bus to implement non-parent-child component communication:
Event Bus
// eventBus.js import Vue from 'vue'; const EventBus = new Vue(); export default EventBus;
Component A
<template> <div> <h2 id="组件A">组件A</h2> <button @click="sendMessage">发送消息</button> </div> </template> <script> import EventBus from './eventBus.js'; export default { methods: { sendMessage() { EventBus.$emit('message', 'Hello World!'); } } }; </script>
Component B
<template> <div> <h2 id="组件B">组件B</h2> <p>{{ message }}</p> </div> </template> <script> import EventBus from './eventBus.js'; export default { data() { return { message: '' }; }, created() { EventBus.$on('message', (message) => { this.message = message; }); } }; </script>
In the above example, an event bus instance EventBus
is created, and then the message
event is triggered in component A and the data Hello World!
is passed to the event bus Example. Component B listens to the message
event when it is created and updates the data after receiving the event.
Summary:
The above is a brief introduction on how to use Vue to implement component communication. In Vue, parent components can communicate with child components through props attributes, child components can communicate with parent components through the $emit method, and non-parent and child components can communicate through the event bus mechanism. I hope this article will help you understand component communication in Vue.
The above is the detailed content of How to use Vue to implement component communication?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is closely integrated with the front-end technology stack to improve development efficiency and user experience. 1) Construction tools: Integrate with Webpack and Rollup to achieve modular development. 2) State management: Integrate with Vuex to manage complex application status. 3) Routing: Integrate with VueRouter to realize single-page application routing. 4) CSS preprocessor: supports Sass and Less to improve style development efficiency.

Netflix chose React to build its user interface because React's component design and virtual DOM mechanism can efficiently handle complex interfaces and frequent updates. 1) Component-based design allows Netflix to break down the interface into manageable widgets, improving development efficiency and code maintainability. 2) The virtual DOM mechanism ensures the smoothness and high performance of the Netflix user interface by minimizing DOM operations.

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
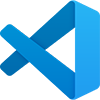
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
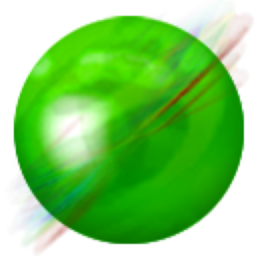
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
