How to develop a real-time drawing sharing application using Vue and Canvas
How to use Vue and Canvas to develop a real-time drawing sharing application
Introduction:
In the era of the Internet, real-time collaboration has become an indispensable part of our life and work. Developing real-time drawing sharing applications is a very common requirement. This article will introduce how to use Vue and Canvas to develop a real-time drawing sharing application, and give corresponding code examples.
1. Preparation
Before starting development, we need to ensure that the Vue and Canvas development environments have been installed on the computer. If it is not installed, you can use the following commands to install it:
# 安装Vue npm install -g @vue/cli # 创建一个新的Vue项目 vue create draw-app # 安装Canvas npm install canvas
2. Draw the basic drawing board interface
Next we will use Vue’s template syntax to draw the basic drawing board interface. In the App.vue file, add the following code:
<template> <div class="app"> <canvas ref="canvas" @mousedown="startDrawing" @mousemove="draw" @mouseup="stopDrawing"></canvas> </div> </template> <script> export default { data() { return { isDrawing: false, context: null, lastX: 0, lastY: 0, }; }, mounted() { this.context = this.$refs.canvas.getContext('2d'); this.$refs.canvas.width = window.innerWidth; this.$refs.canvas.height = window.innerHeight; }, methods: { startDrawing(event) { this.isDrawing = true; [this.lastX, this.lastY] = [event.pageX, event.pageY]; }, draw(event) { if (!this.isDrawing) return; const { context, lastX, lastY } = this; context.beginPath(); context.moveTo(lastX, lastY); context.lineTo(event.pageX, event.pageY); context.stroke(); [this.lastX, this.lastY] = [event.pageX, event.pageY]; }, stopDrawing() { this.isDrawing = false; }, }, }; </script> <style> .app { background-color: #eee; } </style>
In the above code, we bind the mousedown, mousemove and mouseup events to implement the real-time drawing function. Among them, the mousedown event indicates that drawing starts when the mouse is pressed, the mousemove event indicates that the path is drawn when the mouse moves, and the mouseup event indicates that drawing stops when the mouse is raised.
3. Real-time sharing function
To realize the real-time sharing function, we can use WebSocket for real-time messaging. In this article, we will use the socket.io library to simplify the use of WebSockets.
First, we need to install the socket.io library in the project:
npm install socket.io
Then, in the main.js file, add the following code:
import Vue from 'vue'; import App from './App.vue'; import io from 'socket.io-client'; const socket = io('http://localhost:3000'); Vue.prototype.$socket = socket; new Vue({ render: h => h(App), }).$mount('#app');
In the above code , we will create a socket instance and set it as a prototype property of Vue so that it can be used throughout the project.
Next, in the methods attribute of the App.vue file, add the following methods:
methods: { // 省略之前的代码... startDrawing(event) { this.isDrawing = true; [this.lastX, this.lastY] = [event.pageX, event.pageY]; this.$socket.emit('startDrawing', { x: event.pageX, y: event.pageY }); }, draw(event) { if (!this.isDrawing) return; const { context, lastX, lastY } = this; context.beginPath(); context.moveTo(lastX, lastY); context.lineTo(event.pageX, event.pageY); context.stroke(); [this.lastX, this.lastY] = [event.pageX, event.pageY]; this.$socket.emit('draw', { x: event.pageX, y: event.pageY }); }, stopDrawing() { this.isDrawing = false; this.$socket.emit('stopDrawing'); }, },
In the above code, we added three socket.emit() method calls, respectively in Send corresponding messages to the WebSocket server when starting drawing, drawing paths, and stopping drawing.
Finally, we need to implement the WebSocket server on the server side. Here we use Node.js to build the server. Create a new server.js file in the root directory of the project and add the following code:
const server = require('http').createServer(); const io = require('socket.io')(server, { cors: { origin: '*', }, }); io.on('connection', socket => { console.log('New client connected'); socket.on('startDrawing', (data) => { socket.broadcast.emit('startDrawing', data); }); socket.on('draw', (data) => { socket.broadcast.emit('draw', data); }); socket.on('stopDrawing', () => { socket.broadcast.emit('stopDrawing'); }); socket.on('disconnect', () => { console.log('Client disconnected'); }); }); server.listen(3000, () => { console.log('Server listening on port 3000'); });
In the above code, we created an HTTP server and upgraded it to a WebSocket server using the socket.io library. Then, we added listeners for startDrawing, draw, and stopDrawing in the connection event to receive messages sent from the client and broadcast them to other connected clients.
4. Run the application
Now that we have completed the development of the application, we can start the application through the following command:
npm run serve
According to the command line prompts, we can use http:/ /localhost:8080 to access the application. Now, we can open the app in multiple browser windows, use the mouse to draw on the artboard, and share it with other users in real time.
Conclusion:
This article introduces how to use Vue and Canvas to develop a real-time drawing sharing application, and combine it with the socket.io library to implement real-time messaging functions. Through the introduction of this article, readers can master the basic steps of using Vue and Canvas to develop real-time drawing sharing applications, and how to use WebSocket to implement real-time messaging. I hope this article is helpful to readers, thank you for reading.
The above is the detailed content of How to develop a real-time drawing sharing application using Vue and Canvas. For more information, please follow other related articles on the PHP Chinese website!

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
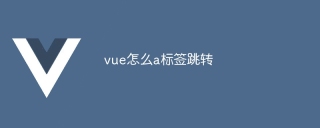
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
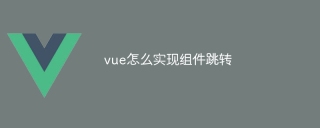
There are the following methods to implement component jump in Vue: use router-link and <router-view> components to perform hyperlink jump, and specify the :to attribute as the target path. Use the <router-view> component directly to display the currently routed rendered components. Use the router.push() and router.replace() methods for programmatic navigation. The former saves history and the latter replaces the current route without leaving records.
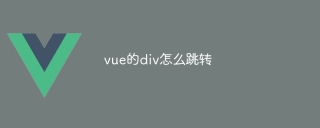
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.
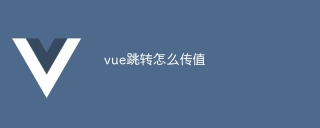
There are two main ways to pass data in Vue: props: one-way data binding, passing data from the parent component to the child component. Events: Pass data between components using events and custom events.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
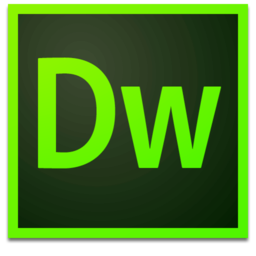
Dreamweaver Mac version
Visual web development tools
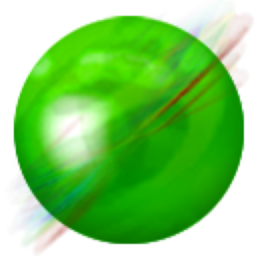
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use