Build a secure authentication system using Golang and Vault
Build a secure authentication system using Golang and Vault
In today's Internet era, protecting the security of user data has become a very important task. Authentication, as the first line of defense to protect user data, is an essential module for any application. To ensure the security of authentication, we can use Golang and Vault to build a secure authentication system.
Vault is an open source tool developed by HashiCorp for secure management and protection of sensitive data, such as API keys, passwords, certificates, etc. It has key management, authentication, access control and other functions, which can help us build a powerful and secure authentication system.
First, we need to install and configure Vault. You can download it from the HashiCorp official website and install and configure it according to the official documentation. Once installed, we can interact with Vault via the HTTP REST API or Golang SDK.
Next, we will write a simple authentication system using Golang. First, we need to introduce Vault’s Golang SDK. It can be installed using the following command:
go get github.com/hashicorp/vault/api
Then, we need to import Vault’s Golang SDK:
import ( "github.com/hashicorp/vault/api" )
Next, we need to set up the connection to Vault. First, we need to obtain the Vault token. You can create a token on Vault's web interface and save it in a configuration file and then read it in your code. Please note that here we only hardcode the token directly into the code for demonstration purposes. In practice, please follow best practices.
config := &api.Config{ Address: "http://localhost:8200", } client, err := api.NewClient(config) if err != nil { log.Fatal(err) } client.SetToken("your_vault_token")
Now that we have successfully connected to Vault, we will create a simple authentication function. We can use Vault's K/V storage engine to store key-value pairs of usernames and passwords. First, we need to create a secret storage path for the K/V storage engine.
path := "/secret/userinfo" data := map[string]interface{}{ "username": "admin", "password": "password123", } _, err := client.Logical().Write(path, data) if err != nil { log.Fatal(err) }
Now that we have stored the username and password in Vault, we will write a verification function to verify that the username and password entered by the user are correct.
func authenticate(username, password string) bool { path := "/secret/userinfo" secret, err := client.Logical().ReadWithData(path, map[string]interface{}{"key": "value"}) if err != nil { log.Fatal(err) } storedUsername := secret.Data["username"].(string) storedPassword := secret.Data["password"].(string) if username == storedUsername && password == storedPassword { return true } return false }
In the above code, we first use Vault’s API to read the stored username and password. We then compare the username and password entered by the user with the stored values. Returns true if there is a match, false otherwise.
Now we can use the above authentication function to verify the user's identity.
username := "admin" password := "password123" result := authenticate(username, password) if result { fmt.Println("Authentication succeeded") } else { fmt.Println("Authentication failed") }
In the above code, we pass the username and password to the authenticate function for authentication. Based on the returned results, we output the corresponding information.
By using Golang and Vault, we can build a secure and strong authentication system. The powerful functions of Vault can help us protect the security of user data, while the simplicity and efficiency of Golang make the development process smoother.
Full code example:
package main import ( "fmt" "log" "github.com/hashicorp/vault/api" ) func main() { config := &api.Config{ Address: "http://localhost:8200", } client, err := api.NewClient(config) if err != nil { log.Fatal(err) } client.SetToken("your_vault_token") path := "/secret/userinfo" data := map[string]interface{}{ "username": "admin", "password": "password123", } _, err = client.Logical().Write(path, data) if err != nil { log.Fatal(err) } username := "admin" password := "password123" result := authenticate(client, username, password) if result { fmt.Println("Authentication succeeded") } else { fmt.Println("Authentication failed") } } func authenticate(client *api.Client, username, password string) bool { path := "/secret/userinfo" secret, err := client.Logical().ReadWithData(path, map[string]interface{}{}) if err != nil { log.Fatal(err) } storedUsername := secret.Data["username"].(string) storedPassword := secret.Data["password"].(string) if username == storedUsername && password == storedPassword { return true } return false }
In this article, we built a simple and secure authentication system using Golang and Vault. By using Vault’s API, we can easily store sensitive data in Vault and use Golang to authenticate users. This way, we ensure the security of our users' data and provide a strong and secure authentication process.
The above is the detailed content of Build a secure authentication system using Golang and Vault. For more information, please follow other related articles on the PHP Chinese website!
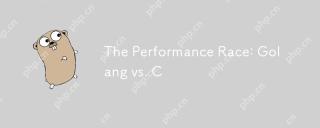
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
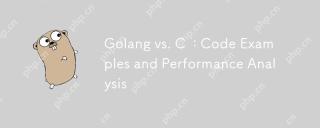
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
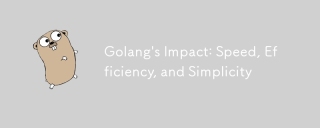
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
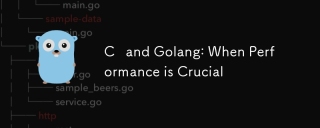
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
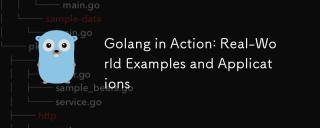
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
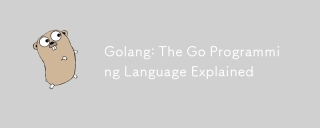
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
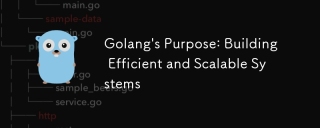
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
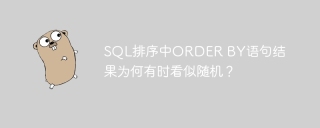
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.