How to use Vue and Axios to request and update page-level data
Introduction:
In today's front-end development, data request and update are very common and important operations. As a popular front-end framework, Vue.js provides a data-driven way of thinking. At the same time, combined with Axios, an excellent HTTP library, it can easily implement page-level data requests and updates. This article will introduce in detail how to use Vue and Axios to request and update page-level data in the Vue project, and provide some related code examples.
-
Installing and introducing Vue and Axios
First, make sure that Vue and Axios have been installed in your project. If it is not installed, you can install it with the following command.npm install vue axios
Next, where Vue and Axios need to be used, introduce them through the following code.
import Vue from 'vue' import axios from 'axios' Vue.prototype.$axios = axios
-
Send a request to obtain data
Generally speaking, we will send a request in the life cycle hook function of the Vue component to obtain data and display it. The following is an example that demonstrates how to send a GET request to obtain data in a Vue component.export default { data() { return { users: [] } }, mounted() { this.$axios.get('/api/users') .then(response => { this.users = response.data }) .catch(error => { console.error(error) }) } }
In the above code, the mounted life cycle hook function will be automatically called after the component is mounted, and then send a GET request to the /api/users interface, and assign the returned data to the data of the component. users attribute.
-
Update data
Once the data is obtained, we can operate and display it in the Vue component. Below is an example showing how to update data in a Vue component.export default { data() { return { users: [] } }, methods: { updateUser(id, newName) { this.$axios.put(`/api/users/${id}`, { name: newName }) .then(response => { // 更新成功,更新对应用户的name const updatedUser = response.data const index = this.users.findIndex(user => user.id === updatedUser.id) if (index !== -1) { this.$set(this.users, index, updatedUser) } }) .catch(error => { console.error(error) }) } } }
In the above code, the updateUser method will receive a user id and new user name as parameters, and send a PUT request to the corresponding interface. When the request is successful, we can update the user's name attribute in the response callback function and ensure responsive updates through Vue's $set method.
-
Supplementary: Sending POST requests and deleting data
In addition to GET and PUT requests, we can also send POST requests to create new data, and send DELETE requests to delete data. Below are two examples demonstrating how to send POST and DELETE requests.export default { methods: { createUser(name) { this.$axios.post('/api/users', { name: name }) .then(response => { // 创建成功,将新用户添加到users数组中 const newUser = response.data this.users.push(newUser) }) .catch(error => { console.error(error) }) }, deleteUser(id) { this.$axios.delete(`/api/users/${id}`) .then(() => { // 删除成功,从users数组中移除对应用户 const index = this.users.findIndex(user => user.id === id) if (index !== -1) { this.users.splice(index, 1) } }) .catch(error => { console.error(error) }) } } }
In the above code, the createUser method will receive a username as a parameter and send a POST request to the /api/users interface to create a new user. When the request is successful, we can add the new user to the users array in the response callback function. The deleteUser method receives a user id as a parameter and sends a DELETE request to the corresponding interface to delete the corresponding user. When the request is successful, we can remove the corresponding user from the users array in the response callback function.
Summary:
Through the combination of Vue and Axios, we can easily implement page-level data requests and updates in the Vue project. Through the above code examples, you can better understand how to use Vue and Axios to send GET, POST, PUT and DELETE requests, and operate and update the returned data. I hope this article can help you understand and use Vue and Axios to handle data requests in your project.
The above is the detailed content of How to use Vue and Axios to request and update page-level data. For more information, please follow other related articles on the PHP Chinese website!
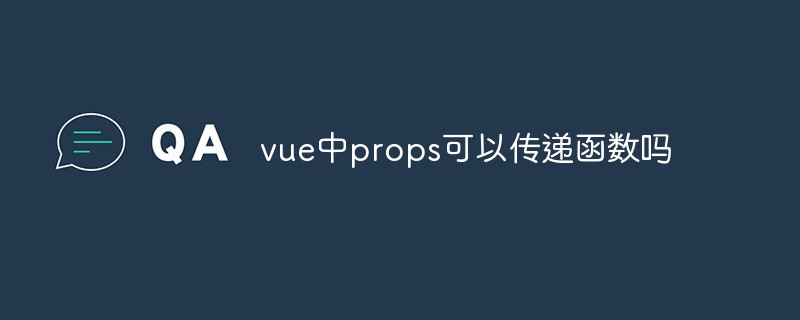
vue中props可以传递函数;vue中可以将字符串、数组、数字和对象作为props传递,props主要用于组件的传值,目的为了接收外面传过来的数据,语法为“export default {methods: {myFunction() {// ...}}};”。
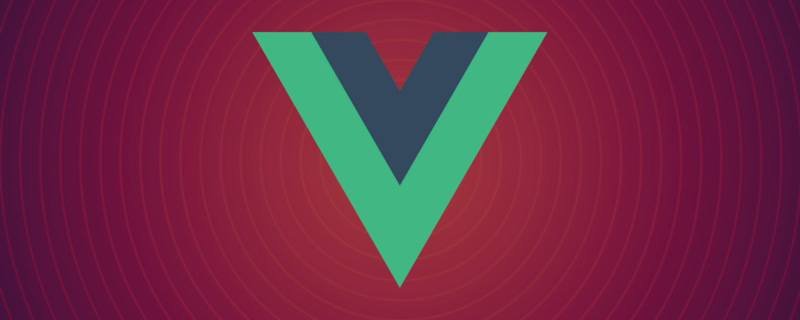
本篇文章带大家聊聊vue指令中的修饰符,对比一下vue中的指令修饰符和dom事件中的event对象,介绍一下常用的事件修饰符,希望对大家有所帮助!
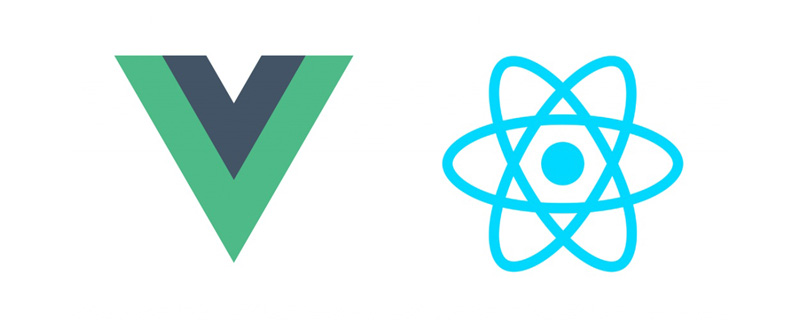
如何覆盖组件库样式?下面本篇文章给大家介绍一下React和Vue项目中优雅地覆盖组件库样式的方法,希望对大家有所帮助!
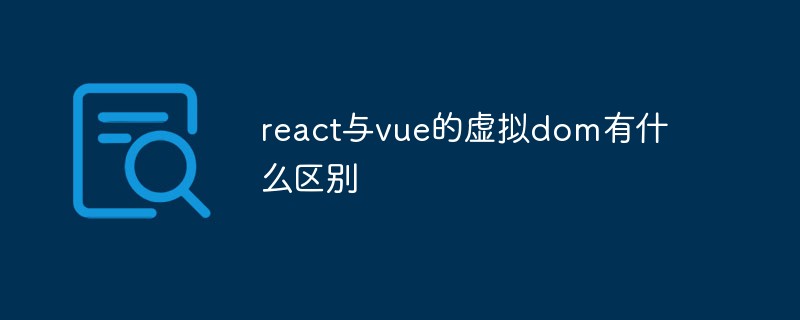
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
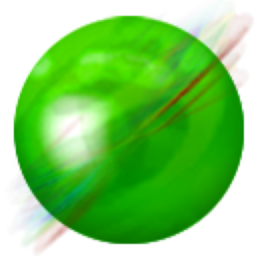
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
