


How to use Workerman to realize the multi-person collaborative editing function of PHP and Unity3D
How to use Workerman to realize the multi-person collaborative editing function of PHP and Unity3D
Introduction:
In today's Internet era, multi-person collaborative editing has become a very important and common functional requirement. Whether it is document editing in team collaboration or scene editing in multiplayer online games, it is necessary to enable multiple people to edit the same file or scene at the same time. This article will introduce how to use the Workerman framework to implement the multi-person collaborative editing function of PHP and Unity3D, and provide code examples.
1. What is Workerman framework?
Workerman is a high-performance PHP socket server framework that can support tens of thousands or even hundreds of thousands of concurrent connections. It is suitable for implementing various network applications such as WebSocket, TCP, and UDP, including multiplayer online games, real-time chat, online document editing, etc.
2. Implementation Principle of Multi-person Collaborative Editing Function
Before realizing the multi-person collaborative editing function, we need to have a certain understanding of its implementation principle. Simply put, whenever an editing action occurs, the Unity3D client will send the action to the server through the WebSocket protocol. After the server receives the action, it will broadcast it to other clients, thereby achieving the effect of multi-person collaborative editing.
3. Server-side implementation
The following is a code example for implementing the server-side using the Workerman framework:
// 引入Workerman的Autoloader require_once 'vendor/autoload.php'; use WorkermanWorker; use WorkermanWebServer; $web = new WebServer('http://0.0.0.0:8080'); $web->count = 1; $ws_worker = new Worker('websocket://0.0.0.0:8000'); $ws_worker->count = 4; $ws_worker->onWorkerStart = function ($worker) { echo "Worker starting... "; }; $ws_worker->onConnect = function ($connection) { echo "New connection established. "; }; $ws_worker->onMessage = function ($connection, $data) use ($ws_worker) { // 处理接收到的编辑动作 // 广播给其他连接 foreach ($ws_worker->connections as $clientConnection) { $clientConnection->send($data); } }; $ws_worker->onClose = function ($connection) { echo "Connection closed. "; }; Worker::runAll();
The above code creates a Web server and a WebSocket server. The Web server listens on port 8080 for receiving HTTP requests from Unity3D, and the WebSocket server listens on port 8000 for receiving WebSocket connections and messages from Unity3D.
4. Unity3D client implementation
The following is a code example of using Unity3D to implement the client (only the core code is shown):
using UnityEngine; using WebSocketSharp; public class SyncEditor : MonoBehaviour { private WebSocket ws; void Start() { // 连接WebSocket服务器 ws = new WebSocket("ws://localhost:8000"); ws.Connect(); ws.OnMessage += OnMessage; } void OnMessage(object sender, MessageEventArgs e) { // 处理接收到的编辑动作 } void OnDestroy() { // 断开WebSocket连接 ws.Close(); } }
The above code creates a WebSocket in the Start() method Connect and process the received editing action in the OnMessage() method.
5. Summary
By using the Workerman framework, we can easily implement the multi-person collaborative editing function of PHP and Unity3D. After the server receives the editing action sent by the Unity3D client, it will broadcast it to other clients, thereby achieving the effect of multi-person collaborative editing. The above is a simple implementation example, and readers can adjust and expand it according to actual needs.
Reference link:
- Workerman framework official documentation: http://www.workerman.net/
- WebSocketSharp official documentation: https://github.com /sta/websocket-sharp
The above is the detailed content of How to use Workerman to realize the multi-person collaborative editing function of PHP and Unity3D. For more information, please follow other related articles on the PHP Chinese website!
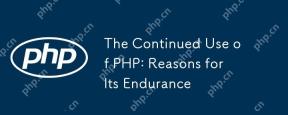
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
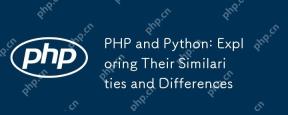
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
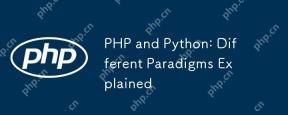
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
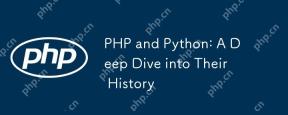
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
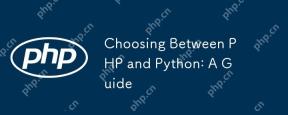
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
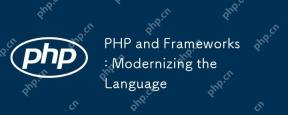
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
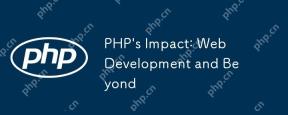
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
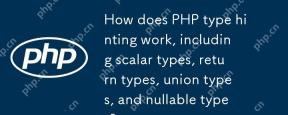
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
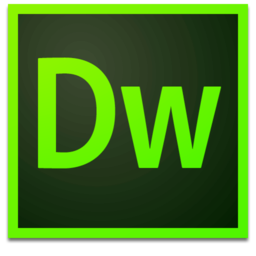
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.