


Detailed explanation of Golang language features: interface-based programming style
Detailed explanation of Golang language features: Interface-based programming style
Introduction:
As a programming language that emphasizes simplicity and efficiency, Golang adopts many unique features in its design, one of which is Interface-based programming style. Interface is a key concept in the Golang language. It is not only used to achieve polymorphism, but also helps developers achieve goals such as loose coupling and high cohesion of code. This article will introduce in detail how to use interfaces in Golang language and explain its features through code examples.
- Definition of interface
In Golang, an interface is a collection of methods that defines a set of behaviors. As long as a type implements all methods in the interface, it is the implementation class of the interface. The definition of the interface uses thetype
keyword, for example:
type Writer interface { Write(data []byte) (int, error) }
The above code defines a Writer
interface, which contains a Write
Method, this method receives a byte array as a parameter and returns the number of bytes written and possible errors.
- Implementation of interface
In order to implement an interface, you only need to implement the specified method in the type definition. For example, if we want to implement theWriter
interface defined above, we can do this:
type FileWriter struct { // 文件路径等相关字段 } func (f *FileWriter) Write(data []byte) (int, error) { // 实现具体的写入逻辑 }
In the above code, we define a FileWriter
structure, And implements the Write
method. In this way, the FileWriter
type becomes the implementation class of the Writer
interface.
- Polymorphism of interfaces
An important feature of interfaces in Golang is polymorphism. This means that a variable of an interface type can accept values of different types, as long as those values implement the interface. For example, we can use the aboveWriter
interface like this:
func WriteData(w Writer, data []byte) { w.Write(data) } func main() { fileWriter := &FileWriter{} networkWriter := &NetworkWriter{} data := []byte("Hello, World!") WriteData(fileWriter, data) WriteData(networkWriter, data) }
In the above code, we define a WriteData
function, which receives a Parameters of type Writer
and call its Write
method. In the main
function, we created a FileWriter
object and a NetworkWriter
object, and called the WriteData
function respectively. Since the FileWriter
and NetworkWriter
types both implement the Writer
interface, they can be used as actual parameters of the WriteData
function.
In this way, we can easily implement different types of write operations without modifying the WriteData
function. This polymorphism achieved through interfaces makes our code more flexible and extensible.
- Nesting of interfaces
In Golang, an interface can be nested within another interface to form a larger interface. The nested interface will inherit its methods, and you can also add your own methods. Here is an example:
type Reader interface { Read() ([]byte, error) } type Closer interface { Close() error } type ReadCloser interface { Reader Closer }
In the above code, we define three interfaces: Reader
, Closer
and ReadCloser
. Among them, the ReadCloser
interface nests the Reader
and Closer
interfaces, and it inherits the methods in the two interfaces.
Through the nesting of interfaces, we can organize multiple related methods into one interface, thereby improving the readability and maintainability of the code.
Conclusion:
Through the introduction of this article, we have a detailed understanding of the interface-based programming style in the Golang language. The definition and implementation of interfaces and the polymorphism of interfaces are important features in Golang. Through the effective use of interfaces, high cohesion and low coupling of code can be achieved, making the code easier to understand, expand, and maintain.
Through continuous research and practice, we can give full play to the role of interfaces in Golang programming, thereby writing more concise, flexible and efficient code. It is recommended that readers not only learn the basic use of interfaces, but also deeply understand the principles and design ideas of interfaces to better apply them to actual projects. I wish everyone will go further and further on the road of Golang programming!
The above is the detailed content of Detailed explanation of Golang language features: interface-based programming style. For more information, please follow other related articles on the PHP Chinese website!
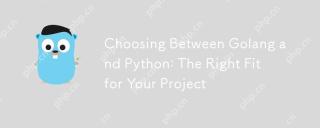
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
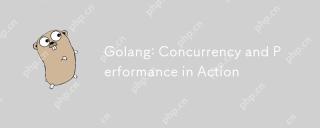
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
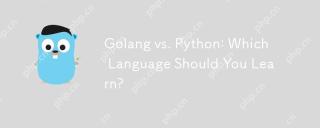
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
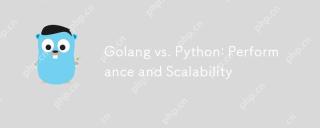
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
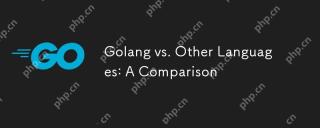
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
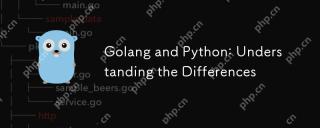
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
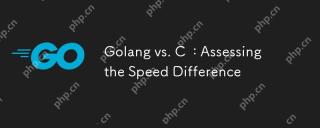
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
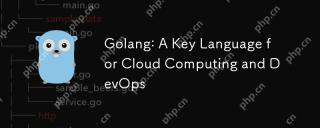
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
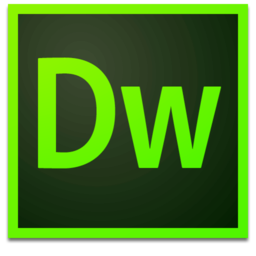
Dreamweaver Mac version
Visual web development tools
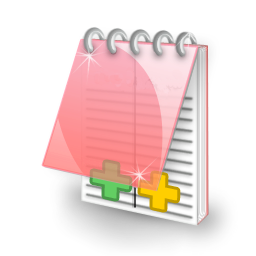
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.