


Advanced Guide to Concurrent Programming in Golang: Master the Advanced Usage of Goroutines
Advanced Guide to Concurrent Programming in Golang: Master the Advanced Usage of Goroutines
With the continuous development of computer technology, the popularity of multi-core processors and the rise of cloud computing, concurrent programming has become more and more important. As a language for developing high-concurrency programs, Golang's concurrency model uses Goroutines and Channels as its core, making concurrent programming simple and efficient.
This article will introduce the advanced usage of Goroutines to help developers better utilize the concurrency features of Golang and improve program performance and reliability. We'll explain each concept and technique through code examples.
- Startup and synchronization of Goroutines
Goroutine is the smallest unit that represents concurrent tasks in Golang. A Goroutine is a lightweight thread. To start a Goroutine, just prepend the keyword "go" to the function name. For example:
func main() { go printHello() time.Sleep(time.Second) } func printHello() { fmt.Println("Hello, World!") }
In the above code, the printHello()
function is started as a Goroutine, which will output "Hello, World!" asynchronously. In order to let the main function wait for the Goroutine to end, we use time.Sleep(time.Second)
.
- Communication between Goroutines through Channel
In Golang, communication between Goroutines is usually implemented using Channel. Channel is a type-safe concurrent data structure used to pass data between Goroutines.
func main() { ch := make(chan int) go produce(ch) go consume(ch) time.Sleep(time.Second) } func produce(ch chan<- int) { for i := 0; i < 10; i++ { ch <- i } close(ch) } func consume(ch <-chan int) { for num := range ch { fmt.Println("Received:", num) } }
In the above code, we define a Channel containing 10 integers. produce()
The function sends 0 to 9 to the Channel in sequence, consume()
The function receives the integer from the Channel and prints it. It should be noted that in the produce()
function we use close(ch)
to close the Channel to notify the consume()
function to stop receiving data.
- Scheduling and synchronization of Goroutines
In concurrent programming, we sometimes need to control the scheduling and synchronization of Goroutines to avoid problems such as race conditions and deadlocks. Golang provides some tools to implement these functions, such as WaitGroup, Mutex, and Cond.
func main() { var wg sync.WaitGroup wg.Add(2) go doWork(&wg) go doWork(&wg) wg.Wait() fmt.Println("All Goroutines completed.") } func doWork(wg *sync.WaitGroup) { defer wg.Done() fmt.Println("Doing work...") time.Sleep(time.Second) }
In the above code, we use sync.WaitGroup
to wait for the two Goroutines to complete their work. At the beginning and end of each Goroutine, we call wg.Add(1)
and defer wg.Done()
respectively to increase and decrease the count of WaitGroup. In the main function, we use wg.Wait()
to wait for all Goroutines to complete.
Summary:
This article introduces the advanced usage of Goroutines in Golang concurrent programming, including starting and synchronizing Goroutines, communicating between Goroutines through Channel, and scheduling and synchronizing Goroutines. By mastering these advanced usages, developers can better utilize Golang's concurrency features and improve program performance and reliability.
In practical applications, we can also use other concurrency primitives and tools provided by Golang to implement more complex functions, such as using atomic operations to implement atomic updates to shared resources and using Select statements to implement multiplexing. Use wait. Through continuous learning and practice, the technical reserves and experience of concurrent programming will gradually be enriched and able to deal with more practical scenarios and complex problems.
The above is the detailed content of Advanced Guide to Concurrent Programming in Golang: Master the Advanced Usage of Goroutines. For more information, please follow other related articles on the PHP Chinese website!
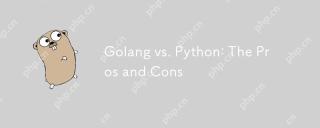
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
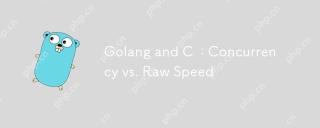
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
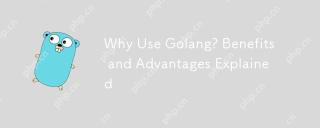
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
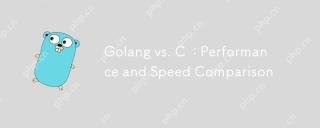
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
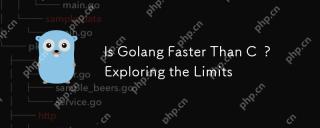
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
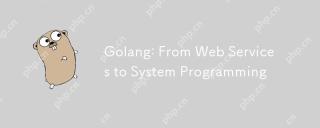
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
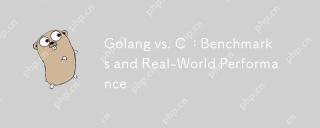
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
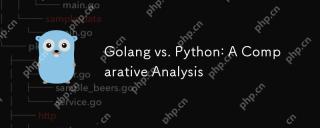
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.