


How to generate thumbnails after PHP saves remote images to local?
When developing a website or application, you often encounter situations where you need to save remote images to the local server, and also need to generate thumbnails to improve page loading speed and save bandwidth. This article will introduce how to use PHP to save remote images to local and use the GD library to generate thumbnails.
- Download remote images to the local server
In PHP, you can use the file_get_contents() function to read the contents of the remote image, and then use the file_put_contents() function to put the contents Save to local server.
<?php // 远程图片URL $remoteImageUrl = "http://example.com/image.jpg"; // 保存到本地的路径 $localImagePath = "/path/to/local/image.jpg"; // 读取远程图片内容 $imageContent = file_get_contents($remoteImageUrl); // 保存到本地 file_put_contents($localImagePath, $imageContent); ?>
In the above code, $remoteImageUrl is the URL of the remote image, and $localImagePath is the path saved locally. Read the contents of the remote image through the file_get_contents() function, and save the contents to the local server using the file_put_contents() function.
- Generate thumbnails
The most common way to generate thumbnails in PHP is to use the GD library. The GD library is a PHP extension library for creating and processing images that can be used in most PHP installations.
First, you need to use the imagecreatefromXXX() function to create an image resource, then use the imagecopyresampled() function to scale the original image to the specified size, and use the imageXXX() function to save the scaled image to the specified file. .
<?php // 原始图片路径 $originalImagePath = "/path/to/local/image.jpg"; // 缩略图路径 $thumbnailImagePath = "/path/to/local/thumbnail.jpg"; // 缩略图尺寸 $thumbnailSize = 200; // 创建原始图片资源 $originalImage = imagecreatefromjpeg($originalImagePath); // 获取原始图片尺寸 $originalWidth = imagesx($originalImage); $originalHeight = imagesy($originalImage); // 计算缩放后的尺寸 if ($originalWidth > $originalHeight) { $thumbnailWidth = $thumbnailSize; $thumbnailHeight = intval($originalHeight / $originalWidth * $thumbnailSize); } else { $thumbnailHeight = $thumbnailSize; $thumbnailWidth = intval($originalWidth / $originalHeight * $thumbnailSize); } // 创建缩略图资源 $thumbnailImage = imagecreatetruecolor($thumbnailWidth, $thumbnailHeight); // 缩放原始图片到缩略图 imagecopyresampled($thumbnailImage, $originalImage, 0, 0, 0, 0, $thumbnailWidth, $thumbnailHeight, $originalWidth, $originalHeight); // 保存缩略图 imagejpeg($thumbnailImage, $thumbnailImagePath); // 释放资源 imagedestroy($originalImage); imagedestroy($thumbnailImage); ?>
In the above code, $originalImagePath is the path of the original image, $thumbnailImagePath is the path of the thumbnail, and $thumbnailSize is the size of the thumbnail. First, use the imagecreatefromjpeg() function to create an original image resource. Then, obtain the size of the original image through the imagesx() and imagesy() functions, and calculate the scaled size based on the thumbnail size. Next, use the imagecreatetruecolor() function to create a thumbnail resource, and then use the imagecopyresampled() function to scale the original image to the thumbnail dimensions. Finally, use the imagejpeg() function to save the thumbnail to the specified file path, and use the imagedestroy() function to release the resources.
Through the above steps, we can download remote images to the local and generate thumbnails to provide to users. In actual development, you can adjust the code according to your own needs, and add error handling and security verification. Hope this article helps you!
The above is the detailed content of How to generate thumbnails after PHP saves remote images to local?. For more information, please follow other related articles on the PHP Chinese website!
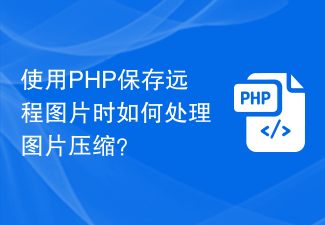
使用PHP保存远程图片时如何处理图片压缩?在实际开发中,我们经常需要从网络上获取图片并保存到本地服务器。然而,有些远程图片可能太大,这就需要我们对它们进行压缩以减少存储空间和提高加载速度。PHP提供了一些功能强大的扩展来处理图片压缩,其中最常用的是GD库和Imagick库。GD库是一个流行的图像处理库,它提供了许多功能用于创建、编辑和保存图像。下面是一个使用
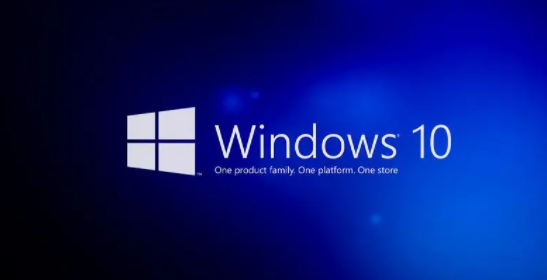
许多用户都不怎么使用win10自带的一个快捷截图,这个截图之后就有用户找不到对应的截图内容保存在哪里,其实这个截图以后不会保存的,是在你的剪切板里面,也就是复制里面,你可以自由地把截图放在任何文件夹。win10截图Win+Shift+S保存在哪答:在你的剪贴板里面。你可以把你的截图文件放到任何的文件夹里面。当你截图之后,你可以把你的文件粘贴到文件夹里面或者是直接点击保存为图片,那样就可以把截图的内容给保存下来了。win10电脑截图快捷键1、“PrtScrSysRq”或者“PrtSc”2、“Win
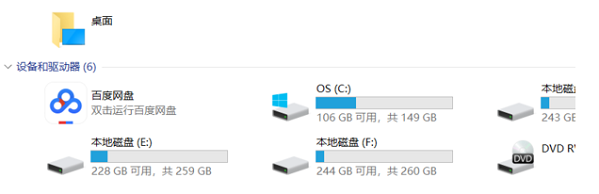
很多用户使用惠普打印机扫描文件过后,不知道扫描的文件保存到哪里去了,想要找一下在哪里,只要在我的电脑中搜索如期就可以进行搜索了。惠普打印机扫描的文件保存在哪儿:1、首先打开我的电脑。2、然后输入日期进行搜索。3、接着就可以找到扫描的文件了。4、打印机驱动安装之后会有一个打印机多功能机辅助软件,把它打开。5、最后点击扫描文件夹图标中就可以找到文件了。
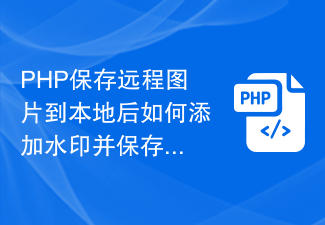
PHP保存远程图片到本地后如何添加水印并保存?在PHP开发中,经常会遇到需要将远程图片保存到本地的需求。而有时候,我们可能还需要在保存后的图片上添加水印以保护版权或增加额外信息。本文将介绍如何使用PHP保存远程图片到本地,并在保存后的图片上添加水印。一、保存远程图片到本地首先,我们需要使用PHP的文件操作函数将远程图片保存到本地。下面是一个简单的示例代码:&
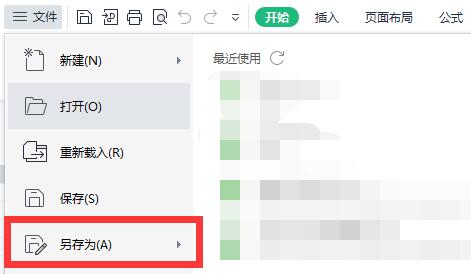
如果我们想要将制作好的Excel表格快速保存到桌面上,但是不知道win11excel怎么保存到桌面,其实使用另存为功能就可以了,还可以直接发送快捷方式。win11excel怎么保存到桌面:一、Excel文件1、首先点开左上角“文件”并选择“另存为”2、接着选择左边的“桌面”3、然后点击右下角“保存”就可以保存Excel到桌面了。二、Excel软件1、如果是想要将Excel软件放到桌面。2、可以在文件夹里找到Excel,右键点击并选择“显示更多选项”3、然后选择“发送到桌面快捷方式”就可以了。
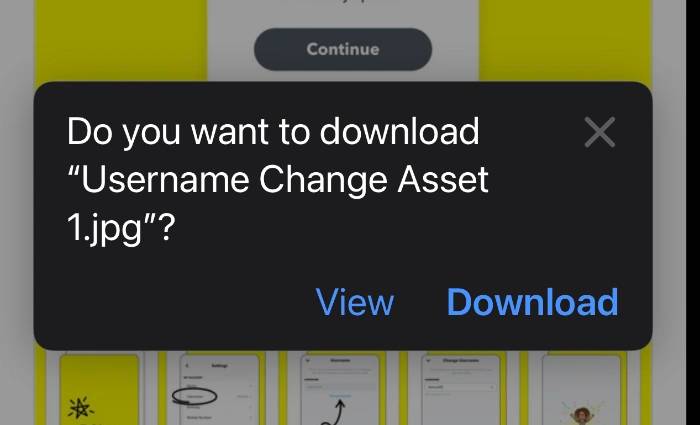
如何将文件从网站下载到我的设备?您可以轻松地将文件从网站直接下载到您的iPhone或iPad。这可以通过转到文件所在的网站,然后单击该文件并选择下载来完成。其中一些文件可能直接在您的Web浏览器中打开,这完全取决于文件类型。当您单击该文件时,您将获得查看或下载文件的选项。从菜单中选择下载,然后文件将下载到您的设备。然后,您可以使用设备上的“文件”应用程序在iPhone或iPad上轻松找到该文件。要查找文件,请选择并打开“文件”应用程序,然后将显示存储在iPhone或iPad上的所有文件。当您在i
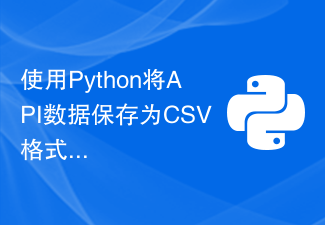
在数据驱动的应用程序和分析领域,API(应用程序编程接口)在从各种来源检索数据方面发挥着至关重要的作用。使用API数据时,通常需要以易于访问和操作的格式存储数据。其中一种格式是CSV(逗号分隔值),它允许有效地组织和存储表格数据。本文将探讨使用强大的编程语言Python将API数据保存为CSV格式的过程。通过遵循本指南中概述的步骤,我们将学习如何从API检索数据、提取相关信息并将其存储在CSV文件中以供进一步分析和处理。让我们深入了解使用Python进行API数据处理的世界,并释放CSV格式的潜
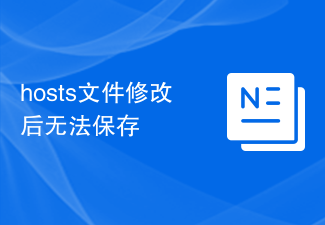
hosts文件是一种用来映射IP地址和主机域名的文本文件,用于解决域名解析问题。在某些情况下,我们可能需要对hosts文件进行修改,添加或删除一些条目。然而,有时候我们可能会遇到hosts文件修改后无法保存的问题。本文将探讨这个问题可能出现的原因,并提供一些解决方法。首先,hosts文件位于操作系统的系统目录中,如Windows操作系统下的路径是C:Wind


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
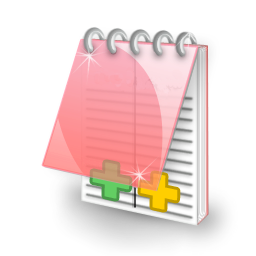
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
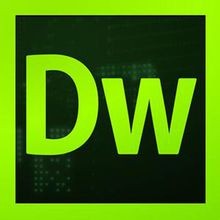
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
