Golang space replacement steps: Import the "`strings" package in the Go program, define a string variable "str", and then use the "strings.ReplaceAll()" function to replace all spaces in the string Replaced with the "#" character, and finally, we print out the original string and the replaced string. In addition to this method, you can also use the "strings.Replace()" and "strings.ReplaceN()" functions to replace spaces.
#The operating environment of this article: Windows 10 system, go1.20 version, dell g3 computer.
In the field of programming, space replacement is a commonly used function, especially when processing text data. In the programming language Golang, we can implement the space replacement function through some methods. This article will introduce how to use Golang to replace spaces and provide a sample code.
First, we need to import the `strings` package in the Go program. This package provides some string operation functions, including replacement operations. We can use the `strings.Replace()` function to implement space replacement.
The following is a sample code that demonstrates how to use Golang to perform space replacement:
package main import ( "fmt" "strings" ) func main() { str := "Hello World! This is a test string." // 将所有空格替换为任意字符,比如"#" replacedStr := strings.ReplaceAll(str, " ", "#") fmt.Println("原始字符串:", str) fmt.Println("替换后的字符串:", replacedStr) }
In the above example, we define a string variable `str`, which contains a text. Then, we use the `strings.ReplaceAll()` function to replace all spaces in the string with the "#" character. Finally, we print out the original string and the replaced string.
The output result is as follows:
原始字符串: Hello World! This is a test string. 替换后的字符串: Hello#World!#This#is#a#test#string.
As you can see, all spaces have been successfully replaced with "#".
In addition to the `strings.ReplaceAll()` function, we can also use other functions to implement space replacement. Here are some commonly used functions:
- `strings.Replace()`: Replaces the specified number of string instances.
- `strings.ReplaceAll()`: Replace all matching string instances.
- `strings.ReplaceN()`: Replaces the specified number of string instances, and specifies the number of replacements.
The sample code is as follows:
package main import ( "fmt" "strings" ) func main() { str := "Hello World! This is a test string." // 将前两个空格替换为"#" replacedStr1 := strings.Replace(str, " ", "#", 2) // 将所有空格替换为"#" replacedStr2 := strings.ReplaceAll(str, " ", "#") // 将所有空格替换为"#", 最多替换3次 replacedStr3 := strings.ReplaceN(str, " ", "#", 3) fmt.Println("原始字符串:", str) fmt.Println("替换后的字符串1:", replacedStr1) fmt.Println("替换后的字符串2:", replacedStr2) fmt.Println("替换后的字符串3:", replacedStr3) }
The output result is as follows:
原始字符串: Hello World! This is a test string. 替换后的字符串1: Hello#World!#This is a test string. 替换后的字符串2: Hello#World!#This#is#a#test#string. 替换后的字符串3: Hello#World!#This#is a test string.
By using these string replacement functions, we can easily implement the space replacement function in Golang. Whether it is to replace all spaces or limit the number of replacements, it can be achieved through these functions.
The above is the detailed content of How to replace spaces in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use
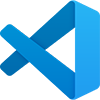
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
