Security control skills for PHP and Oracle database
Security control skills for PHP and Oracle database
Introduction:
In Web development, security is an important consideration. Database security controls are particularly important when it comes to handling sensitive data. As a commonly used server-side scripting language, PHP is widely used in combination with Oracle database. This article will introduce some security control techniques for PHP and Oracle databases, and provide corresponding code examples.
- Use prepared statements
Prepared statements are an important technology that can prevent SQL injection attacks. The PDO (PHP Data Objects) extension in PHP provides support for prepared statements. Here is an example of using prepared statements:
$pdo = new PDO('oci:dbname=Oracle;charset=UTF8', 'username', 'password'); $query = "SELECT * FROM users WHERE username = :username"; $stmt = $pdo->prepare($query); $stmt->bindParam(':username', $username); $username = 'admin'; $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC);
In the above example, we have used :username
as a placeholder and passed bindParam
Bind the value of variable $username
. Doing this ensures that the entered value is not parsed directly into SQL code, thus preventing SQL injection attacks.
- Password Encrypted Storage
During the user registration and login process, the secure storage of passwords is very important. Storing clear text passwords is very dangerous. Once the database is leaked, hackers can easily obtain the user's password. To increase data security, we should store passwords encrypted using a password hash function. Here is an example using PHP's built-in password hashing functionspassword_hash()
andpassword_verify()
:
$password = 'password123'; $hashedPassword = password_hash($password, PASSWORD_DEFAULT); // 将$hashedPassword存入数据库 // 登录时校验密码 $enteredPassword = $_POST['password']; if (password_verify($enteredPassword, $hashedPassword)) { // 密码正确,登录成功 } else { // 密码错误,登录失败 }
In the above example, we Use the password_hash()
function to hash the password, and then store the hashed value in the database. During login verification, use the password_verify()
function to verify whether the password is correct.
- Restrict database user permissions
When using Oracle database, we should assign minimum permissions to database users to prevent unauthorized operations. User permissions can be controlled through the GRANT and REVOKE commands. The following is an example of authorizing a user through the GRANT command:
$pdo = new PDO('oci:dbname=Oracle;charset=UTF8', 'username', 'password'); $query = "GRANT SELECT, INSERT, UPDATE, DELETE ON tableName TO username"; $stmt = $pdo->prepare($query); $stmt->execute();
In the above example, we grant the user SELECT, INSERT, UPDATE, and DELETE permissions through the GRANT command, and specify specific Table name and user name.
- Prevent the leakage of sensitive information
During the code development process, in order to protect the security of sensitive information, you should avoid hardcoding sensitive information directly in the code. Sensitive information includes database connection strings, API keys, etc. This sensitive information can be stored in configuration files and set appropriate permissions to protect the configuration files. Here is an example of storing sensitive information in a configuration file:
// config.php define('DB_HOST', 'localhost'); define('DB_USER', 'username'); define('DB_PASS', 'password'); define('DB_NAME', 'database'); // 使用配置文件中的敏感信息 $pdo = new PDO('oci:dbname='.DB_NAME.';host='.DB_HOST.';charset=UTF8', DB_USER, DB_PASS);
In the above example, sensitive information of the database is stored in the configuration file config.php
, And the constant is defined through the define()
function. When using a database connection, use these constants directly to obtain sensitive information instead of directly writing sensitive information in the code.
Conclusion:
We have introduced several important techniques for security control of PHP and Oracle databases, including using prepared statements, password encrypted storage, restricting database user permissions and preventing sensitive information leakage. These techniques can effectively improve database security and prevent potential security threats. In practical applications, we should follow these best practices and constantly update and strengthen security controls.
Reference materials:
- PHP official documentation: https://www.php.net/
- Oracle official documentation: https://www.oracle. com/
The above is the detailed content of Security control skills for PHP and Oracle database. For more information, please follow other related articles on the PHP Chinese website!
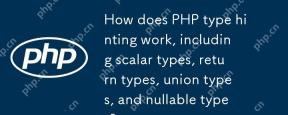
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
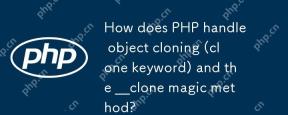
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
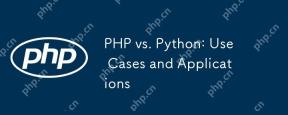
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
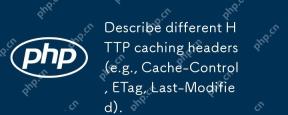
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
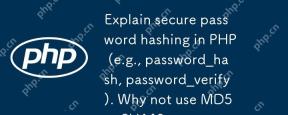
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
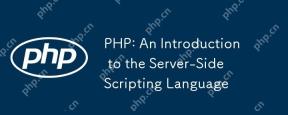
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
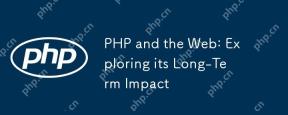
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
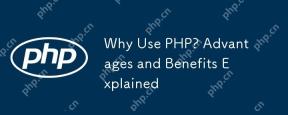
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
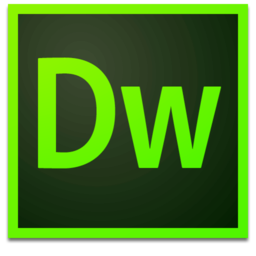
Dreamweaver Mac version
Visual web development tools
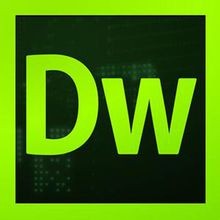
Dreamweaver CS6
Visual web development tools