PHP and GD library tutorial: How to add a transparent effect to pictures
Introduction: In web design and image processing, the transparency effect is one of the commonly used effects. In PHP, we can use the GD library to add transparency to images. This article will introduce how to use PHP and GD library to add transparency effects to images, with code examples.
1. Introduction to GD library
GD library is a library used to process images. It provides a series of functions and tools that can realize image generation, modification, cropping, scaling and other operations. In PHP, the GD library is enabled by default, so we can directly use the functions of the GD library to process images.
2. Add a transparent effect to the picture
To add a transparent effect to the picture, we need to create a transparent canvas first, then copy the original image to the canvas and set the transparency. Next, we can perform some processing on the canvas, such as adding text, drawing graphics, etc.
The following is a sample code that demonstrates how to add a transparent effect to an image:
<?php // 创建画布 $width = 500; // 画布宽度 $height = 500; // 画布高度 $canvas = imagecreatetruecolor($width, $height); // 创建透明颜色 $transparent = imagecolorallocatealpha($canvas, 0, 0, 0, 127); // 填充透明颜色 imagefill($canvas, 0, 0, $transparent); // 加载图片 $imageFile = 'image.jpg'; // 图片文件路径 $image = imagecreatefromjpeg($imageFile); // 将图片复制到画布上 imagecopy($canvas, $image, 0, 0, 0, 0, $width, $height); // 设置透明度 imagealphablending($canvas, false); imagesavealpha($canvas, true); // 添加文字 $fontFile = 'arial.ttf'; // 字体文件路径 $textColor = imagecolorallocate($canvas, 255, 255, 255); // 文字颜色 $text = 'Hello World'; // 文字内容 imagettftext($canvas, 20, 0, 150, 250, $textColor, $fontFile, $text); // 输出图像 header('Content-Type: image/png'); imagepng($canvas); // 释放内存 imagedestroy($canvas); imagedestroy($image); ?>
In the above code, we first create a 500x500 transparent canvas, and then load an image named image. jpg image and copy the image to the canvas. Then, we set the transparency by calling the functions imagealphablending() and imagesavealpha(). Finally, we add a text to the canvas using the function imagettftext().
3. Summary
This article introduces how to use PHP and GD library to add transparency effects to images. We first create a transparent canvas, then load the image and copy it to the canvas, and finally achieve the transparency effect by setting the transparency. In practical applications, we can perform various processing on images according to needs, such as adding watermarks, cropping, adjusting brightness, etc.
Using PHP and GD library to process images is a common requirement. I hope this article can help everyone better understand and apply the related functions of GD library. In actual development, the code can be adjusted according to specific needs and combined with other functions to further optimize the image processing effect.
The above is the detailed content of PHP and GD library tutorial: How to add transparency to images. For more information, please follow other related articles on the PHP Chinese website!
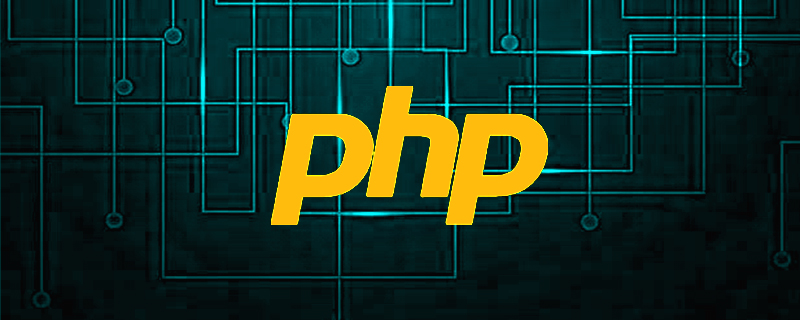
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
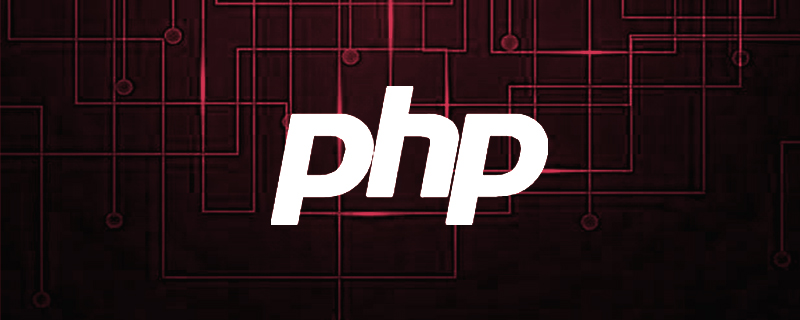
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
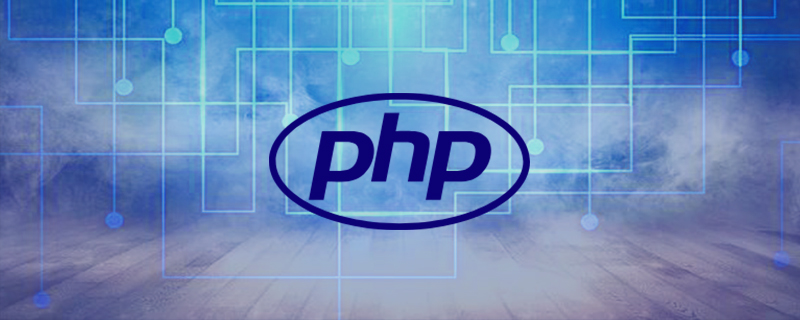
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
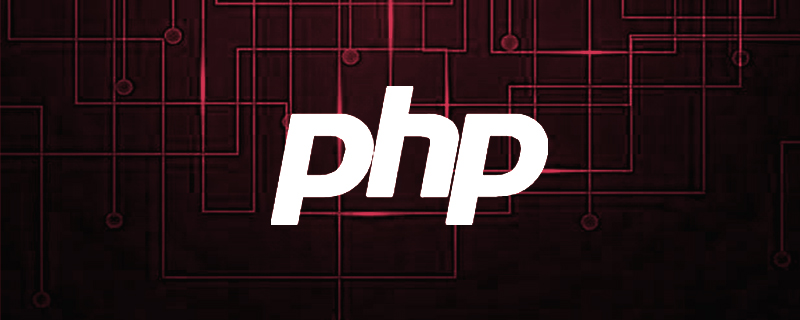
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
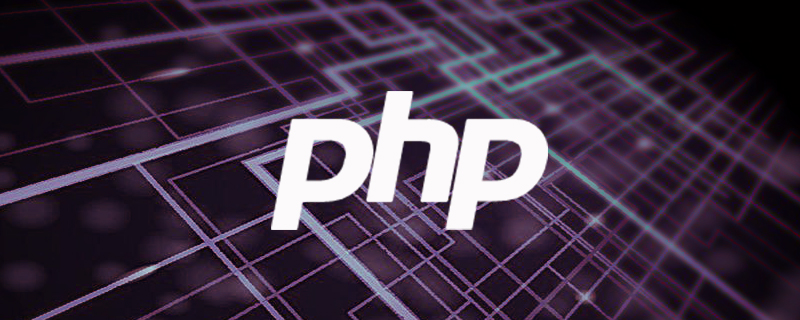
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
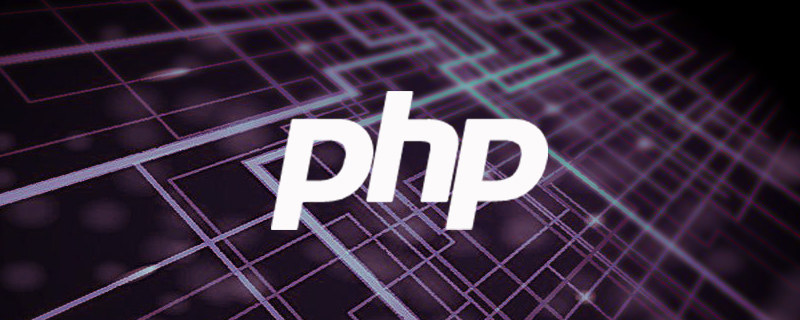
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
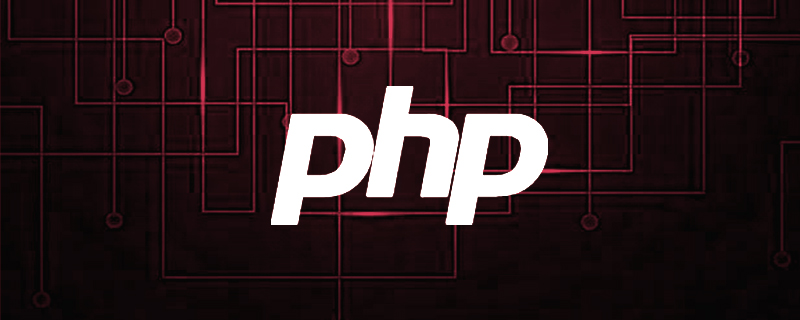
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
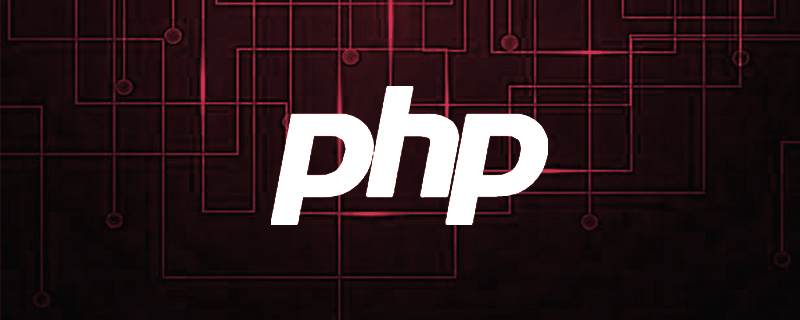
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
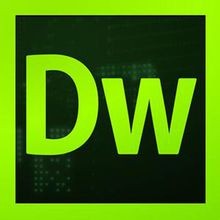
Dreamweaver CS6
Visual web development tools
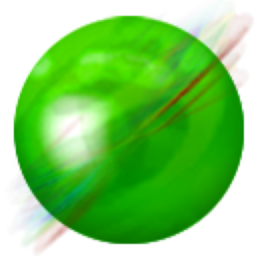
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
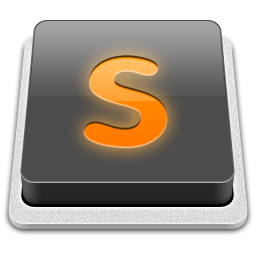
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
