


How to use Oracle database stored procedures and functions in PHP
How to use stored procedures and functions of Oracle database in PHP
Introduction:
Oracle is a commonly used relational database management system. In development, we often need to use stored procedures and functions. To provide more efficient and flexible data processing capabilities. This article will introduce how to use Oracle database stored procedures and functions in PHP, and provide some practical code examples.
1. Create a stored procedure
In Oracle, a stored procedure is a set of precompiled SQL statements that can receive parameters and return results. Below is a simple example that shows how to create a stored procedure in an Oracle database that calculates the sum of two numbers.
- First, open the SQL command line tool in the Oracle database or use a visual tool (such as PL/SQL Developer) to connect to the database.
- Then, enter the following SQL statement to create the stored procedure:
CREATE OR REPLACE PROCEDURE calculate_sum( num1 IN NUMBER, num2 IN NUMBER, sum OUT NUMBER ) AS BEGIN sum := num1 + num2; END; /
In the above example, calculate_sum
is the name of the stored procedure, num1
and num2
are input parameters, and sum
is an output parameter.
- Next, save and compile the stored procedure.
2. Use stored procedures
In PHP, we can connect to the Oracle database through the OCI8 extension and call stored procedures.
- First, make sure the OCI8 extension is installed and enabled.
- In PHP code, use the following code example to connect to the Oracle database:
<?php $conn = oci_connect('username', 'password', 'connection_string'); if (!$conn) { $e = oci_error(); trigger_error(htmlentities($e['message'], ENT_QUOTES), E_USER_ERROR); } ?>
In the above code, replace username
with the username of the database , replace password
with the database password and connection_string
with the database connection string.
- Next, the stored procedure can be called using the following code example:
<?php $sql = "BEGIN calculate_sum(:num1, :num2, :sum); END;"; $stmt = oci_parse($conn, $sql); $num1 = 10; $num2 = 20; oci_bind_by_name($stmt, ':num1', $num1); oci_bind_by_name($stmt, ':num2', $num2); oci_bind_by_name($stmt, ':sum', $sum, 20); oci_execute($stmt); echo "Sum: " . $sum; oci_free_statement($stmt); oci_close($conn); ?>
In the above code, calculate_sum
is the name of the stored procedure, :num1
, :num2
and :sum
are parameter names. You can use the oci_bind_by_name
function to bind PHP variables to the parameters of a stored procedure in order to pass parameters and receive results.
Please note that the oci_parse
function is used to parse the SQL statement and returns a statement handle, and the oci_execute
function is used to execute the SQL statement.
3. Create functions
In addition to stored procedures, Oracle also supports creating functions. A function is a set of precompiled SQL statements that can receive parameters and return a single result. Below is a simple example that shows how to create a function in Oracle database that calculates the difference between two numbers.
- Open the SQL command line tool in the Oracle database or use a visual tool (such as PL/SQL Developer) to connect to the database.
- Enter the following SQL statement to create the function:
CREATE OR REPLACE FUNCTION calculate_difference( num1 IN NUMBER, num2 IN NUMBER ) RETURN NUMBER AS diff NUMBER; BEGIN diff := num1 - num2; RETURN diff; END; /
In the above example, calculate_difference
is the name of the function, num1
and num2
is the input parameter.
- Save and compile the function.
4. Using functions
The method of using Oracle functions is similar to using stored procedures. The following is a sample code for calling the above function in PHP:
<?php $conn = oci_connect('username', 'password', 'connection_string'); if (!$conn) { $e = oci_error(); trigger_error(htmlentities($e['message'], ENT_QUOTES), E_USER_ERROR); } $sql = "SELECT calculate_difference(:num1, :num2) AS difference FROM DUAL"; $stmt = oci_parse($conn, $sql); $num1 = 50; $num2 = 30; oci_bind_by_name($stmt, ':num1', $num1); oci_bind_by_name($stmt, ':num2', $num2); oci_execute($stmt); $result = oci_fetch_assoc($stmt); echo "Difference: " . $result['DIFFERENCE']; oci_free_statement($stmt); oci_close($conn); ?>
In the above code, :num1
and :num2
are the parameter names of the function, DUAL
is a virtual table in Oracle, used to return single row and single column results.
Conclusion:
By using stored procedures and functions, we can provide more efficient and flexible data processing capabilities. When using the stored procedures and functions of the Oracle database in PHP, you can connect to the database through the OCI8 extension and call the stored procedures and functions. This article provides relevant code examples, hoping to help readers better understand and apply.
The above is the detailed content of How to use Oracle database stored procedures and functions in PHP. For more information, please follow other related articles on the PHP Chinese website!
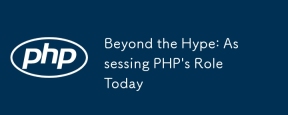
PHP remains a powerful and widely used tool in modern programming, especially in the field of web development. 1) PHP is easy to use and seamlessly integrated with databases, and is the first choice for many developers. 2) It supports dynamic content generation and object-oriented programming, suitable for quickly creating and maintaining websites. 3) PHP's performance can be improved by caching and optimizing database queries, and its extensive community and rich ecosystem make it still important in today's technology stack.
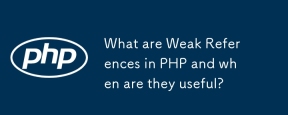
In PHP, weak references are implemented through the WeakReference class and will not prevent the garbage collector from reclaiming objects. Weak references are suitable for scenarios such as caching systems and event listeners. It should be noted that it cannot guarantee the survival of objects and that garbage collection may be delayed.
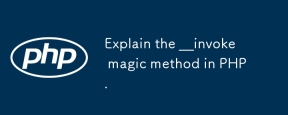
The \_\_invoke method allows objects to be called like functions. 1. Define the \_\_invoke method so that the object can be called. 2. When using the $obj(...) syntax, PHP will execute the \_\_invoke method. 3. Suitable for scenarios such as logging and calculator, improving code flexibility and readability.
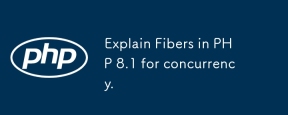
Fibers was introduced in PHP8.1, improving concurrent processing capabilities. 1) Fibers is a lightweight concurrency model similar to coroutines. 2) They allow developers to manually control the execution flow of tasks and are suitable for handling I/O-intensive tasks. 3) Using Fibers can write more efficient and responsive code.
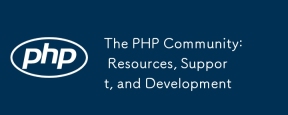
The PHP community provides rich resources and support to help developers grow. 1) Resources include official documentation, tutorials, blogs and open source projects such as Laravel and Symfony. 2) Support can be obtained through StackOverflow, Reddit and Slack channels. 3) Development trends can be learned by following RFC. 4) Integration into the community can be achieved through active participation, contribution to code and learning sharing.
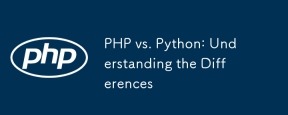
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
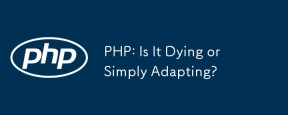
PHP is not dying, but constantly adapting and evolving. 1) PHP has undergone multiple version iterations since 1994 to adapt to new technology trends. 2) It is currently widely used in e-commerce, content management systems and other fields. 3) PHP8 introduces JIT compiler and other functions to improve performance and modernization. 4) Use OPcache and follow PSR-12 standards to optimize performance and code quality.
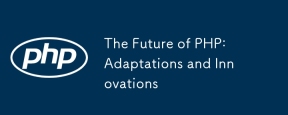
The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
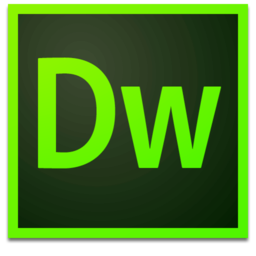
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.