Data preparation skills for PHP and Oracle database
Overview:
When developing using PHP and Oracle database, data preparation is a very important link. This article will introduce some common data preparation techniques, including connecting to the database, creating tables, inserting data, querying data, etc. At the same time, to facilitate understanding, relevant code examples will be provided in the article.
Connecting to the database:
In PHP, you can use the OCI extension to connect to the Oracle database. First, make sure the extension is enabled. Then, use the oci_connect()
function provided by the OCI extension to connect. The following is an example of connecting to an Oracle database:
<?php $username = 'your_username'; $password = 'your_password'; $connection = oci_connect($username, $password, 'localhost/XE'); if (!$connection) { $error = oci_error(); die('数据库连接失败: ' . $error['message']); } echo '数据库连接成功'; oci_close($connection); ?>
Creating tables:
Creating tables is an important step in database preparation. In Oracle database, you can use the CREATE TABLE
statement to create a table. Here is an example of creating a simple table:
<?php $sql = "CREATE TABLE users ( id NUMBER PRIMARY KEY, name VARCHAR(50), email VARCHAR(50) )"; $stmt = oci_parse($connection, $sql); oci_execute($stmt); echo '表格创建成功'; ?>
Inserting data:
Once the table is created, we can insert data. Use the INSERT INTO
statement to insert data. The following is an example of inserting data:
<?php $sql = "INSERT INTO users (id, name, email) VALUES (1, 'John', 'john@example.com')"; $stmt = oci_parse($connection, $sql); oci_execute($stmt); echo '数据插入成功'; ?>
Querying data:
Querying data is one of the common operations. In the Oracle database, you can use the SELECT
statement to query data. The following is an example of querying data:
<?php $sql = "SELECT * FROM users"; $stmt = oci_parse($connection, $sql); oci_execute($stmt); while ($row = oci_fetch_array($stmt, OCI_ASSOC+OCI_RETURN_NULLS)) { echo 'ID: ' . $row['ID'] . ', Name: ' . $row['NAME'] . ', Email: ' . $row['EMAIL']; echo '<br>'; } ?>
Updating data:
In addition to inserting and querying data, we can also update data. Use the UPDATE
statement to update data. The following is an example of updating data:
<?php $sql = "UPDATE users SET email = 'new_email@example.com' WHERE id = 1"; $stmt = oci_parse($connection, $sql); oci_execute($stmt); echo '数据更新成功'; ?>
Deleting data:
Finally, we can use the DELETE
statement to delete data. The following is an example of deleting data:
<?php $sql = "DELETE FROM users WHERE id = 1"; $stmt = oci_parse($connection, $sql); oci_execute($stmt); echo '数据删除成功'; ?>
Notes:
There are some things to pay attention to when performing database operations. First, ensure the security of input data to avoid SQL injection attacks. Secondly, close the database connection in time to avoid resource occupation. Finally, use transactions appropriately to ensure data integrity and consistency.
Conclusion:
When developing using PHP and Oracle database, it is very important to prepare the data properly. By connecting to the database, creating tables, inserting data, querying data and other operations, we can make full use of the advantages of PHP and Oracle to quickly develop efficient and stable applications. Hopefully the sample code in this article will help readers better understand and apply these techniques.
The above is the detailed content of Data Preparation Tips for PHP and Oracle Database. For more information, please follow other related articles on the PHP Chinese website!
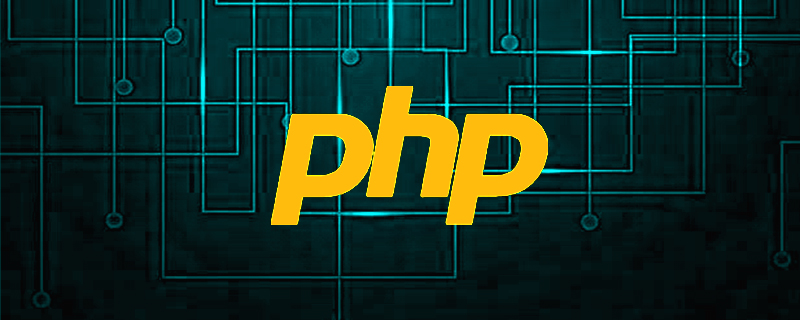
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
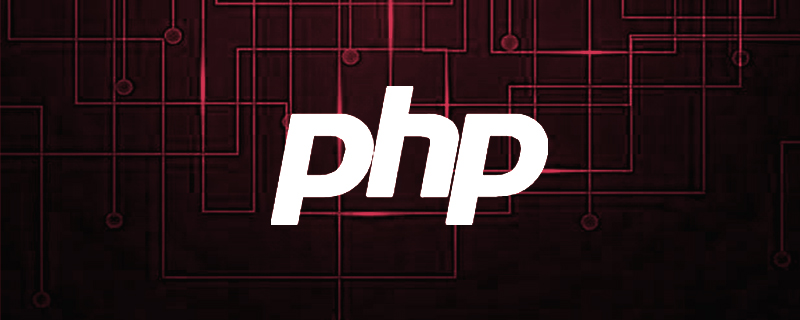
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
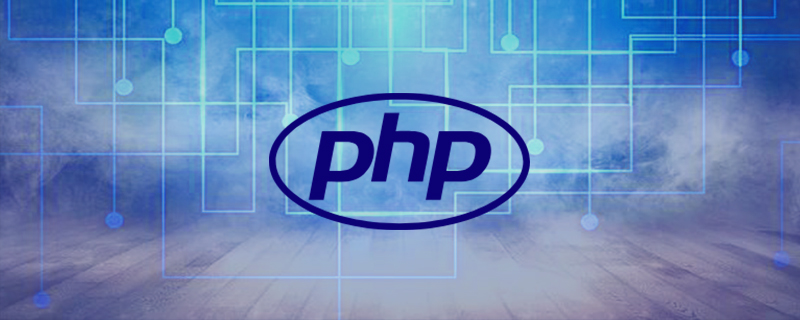
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
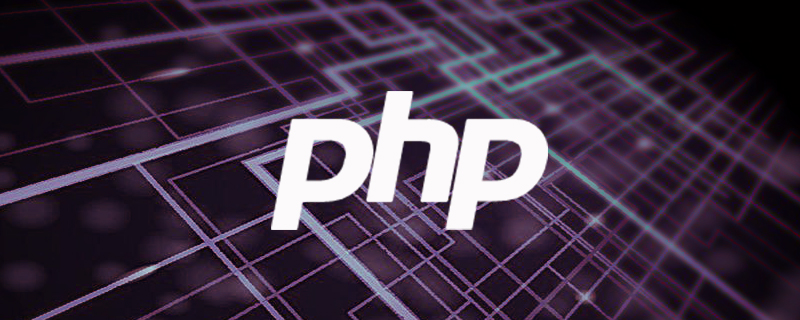
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
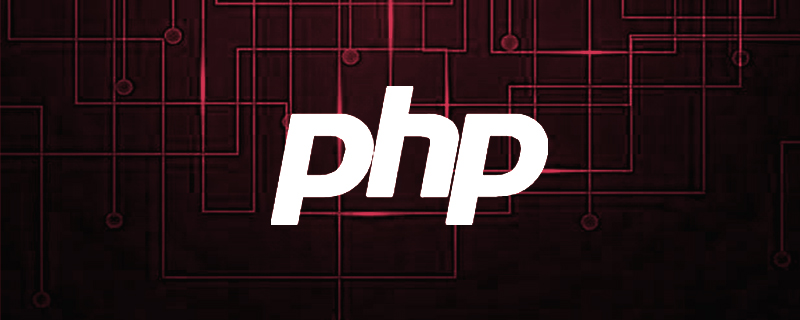
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
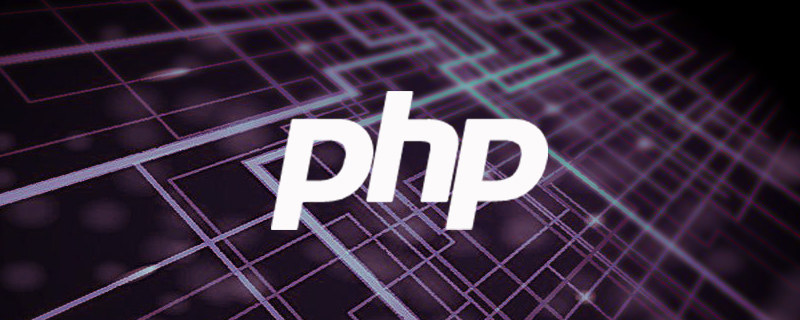
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
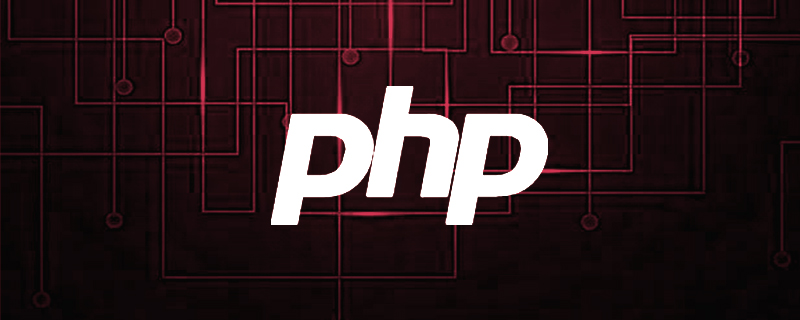
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
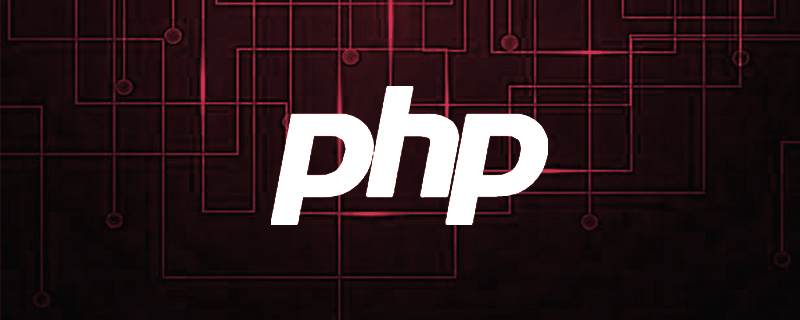
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
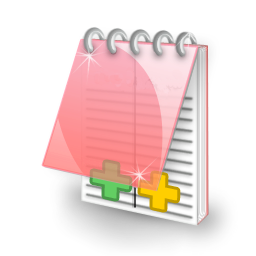
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
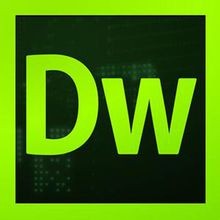
Dreamweaver CS6
Visual web development tools
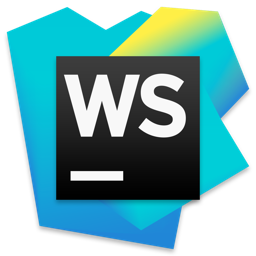
WebStorm Mac version
Useful JavaScript development tools
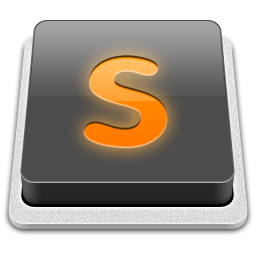
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
