How to use PHP to implement selection sorting algorithm
Selection sorting is a simple and intuitive sorting algorithm. Its basic idea is to select the smallest (or largest) from the data elements to be sorted in each pass. An element is stored at the beginning of the result sequence until all data elements to be sorted are exhausted. Below we will implement the selection sort algorithm through PHP code and explain it in detail.
First, let’s take a look at the implementation steps of the selection sort algorithm:
- Traverse the entire array to be sorted, taking the first element as the minimum value (default).
- According to the minimum value, traverse the remaining elements, find elements smaller than the current minimum value, and update the minimum value.
- Exchange the minimum value with the current traversed position.
- Repeat steps 2-3 until the array sorting is completed.
The following is a code example of using PHP to implement the selection sort algorithm:
function selectionSort($arr) { $len = count($arr); for($i = 0; $i < $len - 1; $i++) { $minIndex = $i; for($j = $i + 1; $j < $len; $j++) { if($arr[$j] < $arr[$minIndex]) { $minIndex = $j; } } // Swap the minimum value with the current position $temp = $arr[$minIndex]; $arr[$minIndex] = $arr[$i]; $arr[$i] = $temp; } return $arr; } // Test the selectionSort function $testArray = [64, 25, 12, 22, 11]; echo "Before sorting: "; print_r($testArray); echo "After sorting: "; print_r(selectionSort($testArray));
Run the above code, the output result will be:
Before sorting: Array ( [0] => 64 [1] => 25 [2] => 12 [3] => 22 [4] => 11 ) After sorting: Array ( [0] => 11 [1] => 12 [2] => 22 [3] => 25 [4] => 64 )
This is sorting by selection The result of an algorithm sorting an array. Next, let’s explain the specific implementation process of the code.
In the code, we define a function named selectionSort
, which accepts an array to be sorted as a parameter and returns the sorted array.
First, we use the count
function to get the length of the array and assign it to the variable $len
. We then use two nested for
loops to iterate through the entire array.
In the outer for
loop, we define a variable $minIndex
to save the index of the current minimum value, which defaults to the current loop variable $i
. In the inner for
loop, we update the index of the minimum value by comparing the size of the current element and the minimum value.
When the inner for
loop ends, we exchange the current minimum value with the current position. Swap the values of two elements by using a temporary variable $temp
.
Finally, we return the sorted array.
The time complexity of the selection sort algorithm is O(n^2), where n is the length of the array to be sorted. This is because each traversal needs to find the minimum value among the remaining elements and perform a swap operation. Regardless of the initial state of the array, n-1 traversals must be performed.
I hope that through the code examples and explanations in this article, you can better understand the implementation process of the selection sort algorithm and be able to use it flexibly in actual development.
The above is the detailed content of How to implement selection sort algorithm with PHP. For more information, please follow other related articles on the PHP Chinese website!
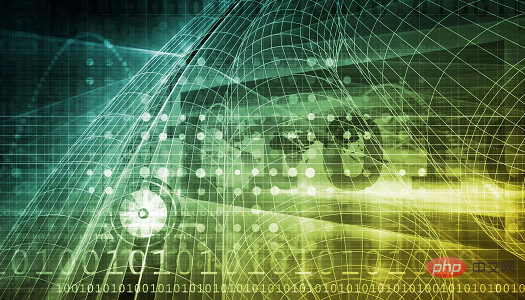
一、问题背景1、双边市场实验介绍双边市场,即平台,包含生产者与消费者两方参与者,双方相互促进。比如快手有视频的生产者,视频的消费者,两种身份可能存在一定程度重合。双边实验是在生产者和消费者端组合分组的实验方式。双边实验具有以下优点:(1)可以同时检测新策略对两方面的影响,例如产品DAU和上传作品人数变化。双边平台往往有跨边网络效应,读者越多,作者越活跃,作者越活跃,读者也会跟着增加。(2)可以检测效果溢出和转移。(3)帮助我们更好得理解作用的机制,AB实验本身不能告诉我们原因和结果之间的关系,只
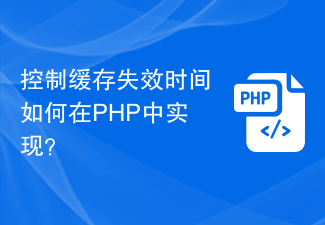
随着互联网应用的普及,网站响应速度越来越成为用户关注的重点。为了快速响应用户的请求,网站往往采用缓存技术缓存数据,从而减少数据库查询次数。但是,缓存的过期时间对响应速度有着重要影响。本文将对控制缓存失效时间的方法进行探讨,以帮助PHP开发者更好地应用缓存技术。一、什么是缓存失效时间?缓存失效时间是指缓存中的数据被认为已经过期的时间。它决定了缓存中的数据何时需
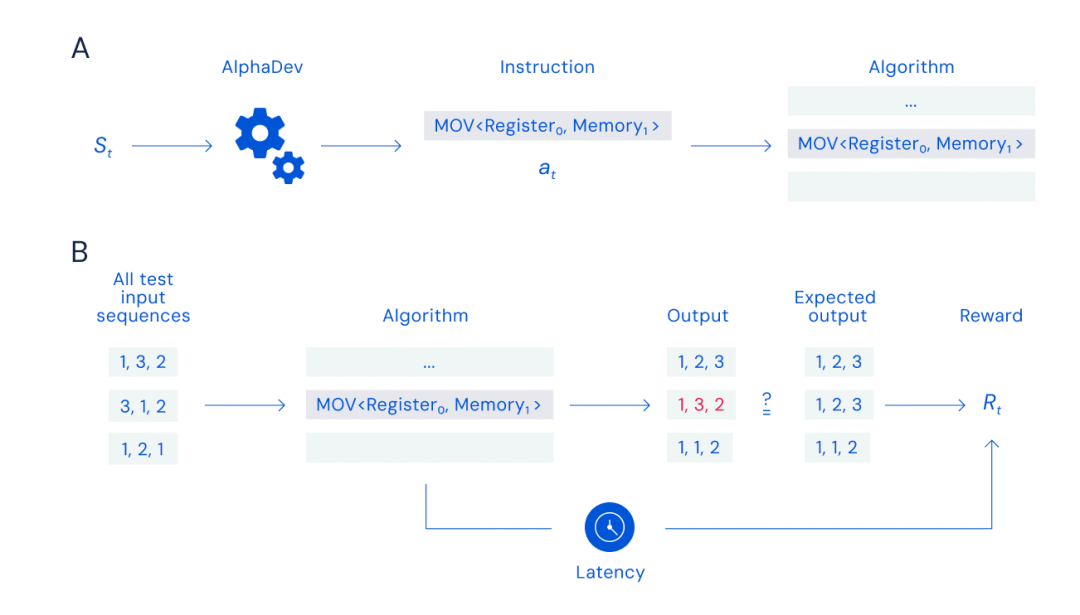
整理|核子可乐,褚杏娟接触过基础计算机科学课程的朋友们,肯定都曾亲自动手设计排序算法——也就是借助代码将无序列表中的各个条目按升序或降序方式重新排列。这是个有趣的挑战,可行的操作方法也多种多样。人们曾投入大量时间探索如何更高效地完成排序任务。作为一项基础操作,大多数编程语言的标准库中都内置有排序算法。世界各地的代码库中使用了许多不同的排序技术和算法来在线组织大量数据,但至少就与LLVM编译器配套使用的C++库而言,排序代码已经有十多年没有任何变化了。近日,谷歌DeepMindAI小组如今开发出一
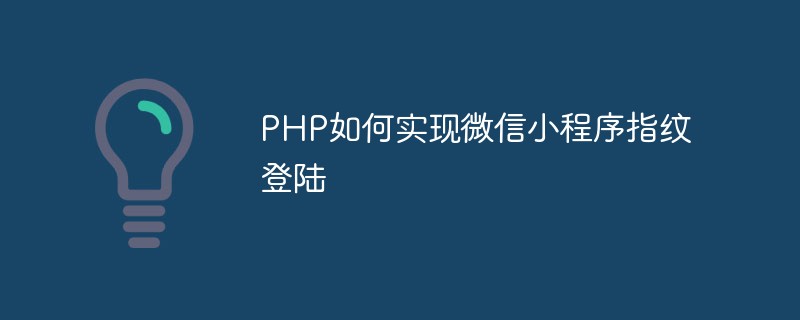
随着微信小程序的不断发展,越来越多的用户开始选择微信小程序进行登陆。为了提高用户的登录体验,微信小程序开始支持指纹登陆。在本文中,我们将会介绍如何使用PHP来实现微信小程序的指纹登陆。一、了解微信小程序的指纹登陆在微信小程序的基础上,开发者可以使用微信的指纹识别功能,让用户通过指纹登陆微信小程序,从而提高登录体验的安全性和便捷性。二、准备工作在使用PHP实现
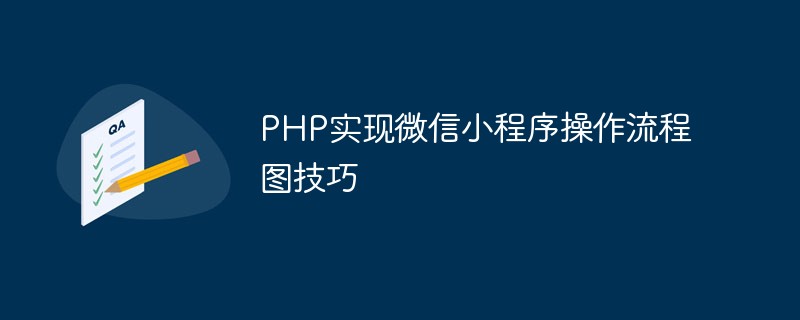
随着移动互联网的快速发展,微信小程序越来越受到广大用户的青睐,而PHP作为一种强大的编程语言,在小程序开发过程中也发挥着重要的作用。本文将介绍PHP实现微信小程序操作流程图的技巧。获取access_token在使用微信小程序开发过程中,首先需要获取access_token,它是实现微信小程序操作的重要凭证。在PHP中获取access_token的代码如下:f
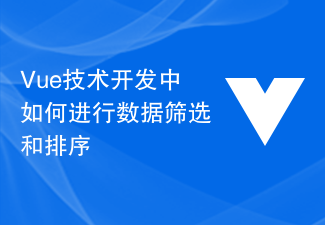
Vue技术开发中如何进行数据筛选和排序在Vue技术开发中,数据筛选和排序是非常常见和重要的功能。通过数据筛选和排序,我们可以快速查询和展示我们需要的信息,提高用户体验。本文将介绍在Vue中如何进行数据筛选和排序,并提供具体的代码示例,帮助读者更好地理解和运用这些功能。一、数据筛选数据筛选是指根据特定的条件筛选出符合要求的数据。在Vue中,我们可以通过comp
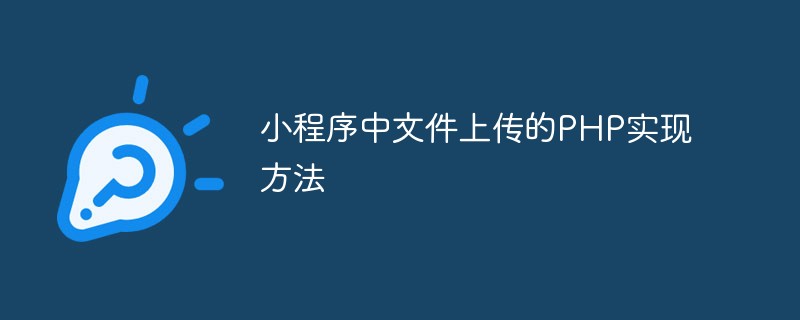
随着小程序的广泛应用,越来越多的开发者需要将其与后台服务器进行数据交互,其中最常见的业务场景之一就是上传文件。本文将介绍在小程序中实现文件上传的PHP后台实现方法。一、小程序中的文件上传在小程序中实现文件上传,主要依赖于小程序APIwx.uploadFile()。该API接受一个options对象作为参数,其中包含了要上传的文件路径、需要传递的其他数据以及
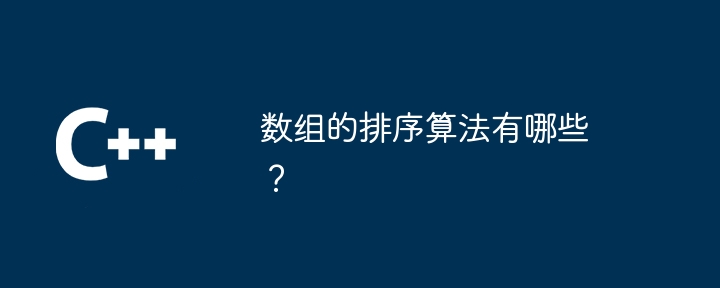
数组排序算法用于按特定顺序排列元素。常见的算法类型包括:冒泡排序:通过比较相邻元素交换位置。选择排序:找出最小元素交换到当前位置。插入排序:逐个插入元素到正确位置。快速排序:分治法,选择枢纽元素划分数组。合并排序:分治法,递归排序和合并子数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
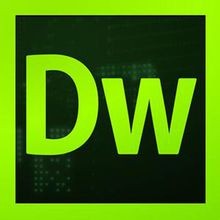
Dreamweaver CS6
Visual web development tools
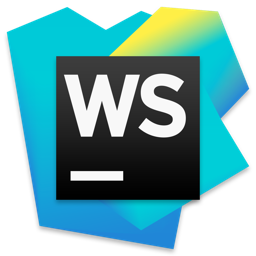
WebStorm Mac version
Useful JavaScript development tools