Application practice of PhpFastCache in big data processing
Introduction:
In today's big data era, data processing is becoming more and more important and complex. When dealing with large data sets, we need to optimize algorithms and reduce the time of reading and writing data. PhpFastCache is a powerful and easy-to-use caching solution that can effectively improve the performance of data processing. In this article, we will introduce the basic concepts and usage of PhpFastCache, and demonstrate its application practice in big data processing through sample code.
1. Introduction to PhpFastCache
PhpFastCache is a lightweight, open source, customizable PHP caching library. It supports multiple cache storage backends, including file cache, database cache, memory cache, etc. PhpFastCache also provides a convenient API to easily access and manage data cache.
2. Install PhpFastCache
Before starting to use PhpFastCache, we need to install it first. PhpFastCache can be installed through Composer, just execute the following command in the project directory:
composer require phpfastcache/phpfastcache
3. Basic usage of PhpFastCache
The following is a simple code example that demonstrates how to use PhpFastCache to cache a piece of data to the file and check the cache first before reading the data.
<?php use phpFastCacheCacheManager; // 初始化PhpFastCache CacheManager::setDefaultConfig([ "path" => "/path/to/cache/folder", "itemDetailedDate" => false ]); $cache = CacheManager::getInstance("files"); // 检查缓存中是否存在数据 $key = "my_data_key"; $data = $cache->getItem($key, $success); if(!$success) { // 从数据库或其他数据源获取数据 $data = fetchDataFromDatabase(); // 将数据缓存到文件中,设置过期时间为1个小时 $cache->setItem($key, $data)->expiresAfter(3600); } // 使用数据 processData($data);
In the above example, we first set the cache path through the CacheManager::setDefaultConfig()
method. Then a cache instance is initialized through the CacheManager::getInstance()
method. Here we choose the file caching method.
Next, we check if the data exists in the cache. If the data does not exist in the cache, the data is obtained from the database or other data source and cached into a file. Here, we set the expiration time of the data to 1 hour.
Finally, we use the obtained data for further processing.
4. Application practice of PhpFastCache in big data processing
In big data processing, we usually need to process a very large amount of data, and we need to read and write data frequently. At this time, using cache can effectively reduce access to the database or other data sources and improve data processing performance.
The following is an example that demonstrates how to use PhpFastCache to process large amounts of data:
<?php use phpFastCacheCacheManager; // 初始化PhpFastCache CacheManager::setDefaultConfig([ "path" => "/path/to/cache/folder", "itemDetailedDate" => false ]); $cache = CacheManager::getInstance("files"); // 模拟处理大量的数据 $numberOfData = 100000; for ($i = 0; $i < $numberOfData; $i++) { $key = "data_" . $i; $data = $cache->getItem($key, $success); if (!$success) { // 从数据库或其他数据源获取数据 $data = fetchDataFromDatabase($i); // 将数据缓存到文件中,设置过期时间为1个小时 $cache->setItem($key, $data)->expiresAfter(3600); } // 使用数据 processData($data); }
In the above example, we simulated a large amount of data processing through a loop. Before processing each piece of data, we first check whether the data exists in the cache. If the data does not exist in the cache, the data is obtained from the database or other data source and cached into a file. Finally, we use the obtained data for further processing.
By using PhpFastCache, we can avoid repeated access to the database, thus greatly improving the performance of big data processing.
Conclusion:
In this article, we introduced the basic concepts and usage of PhpFastCache, and demonstrated its application practice in big data processing through sample code. By using PhpFastCache, we can easily implement data caching to improve the performance of big data processing. In actual development, we can choose the appropriate cache backend according to specific needs, and optimize the cache strategy by setting parameters such as expiration time. I hope this article can help readers better understand and apply PhpFastCache.
The above is the detailed content of Application practice of PhpFastCache in big data processing. For more information, please follow other related articles on the PHP Chinese website!
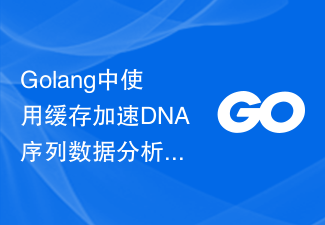
Golang中使用缓存加速DNA序列数据分析的实践技巧随着信息时代的发展,生物信息学成为越来越重要的领域。其中的DNA序列数据分析是生物信息学的基础。对于DNA序列数据的分析,通常需要处理海量的数据。在这种情况下,数据处理效率成为了关键。因此,如何提高DNA序列数据分析的效率成为了一个问题。本文将介绍一种使用缓存来加速DNA序列数据分析的实践技巧,以便提高数
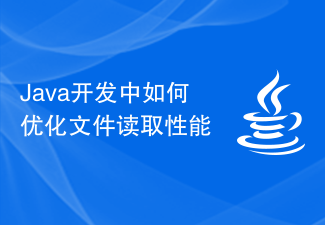
在Java开发过程中,文件读取是一项常见且重要的操作。无论是读取配置文件、日志文件还是大型数据文件,优化文件读取性能都能为我们的应用程序带来巨大的好处。本文将介绍一些常用的Java文件读取性能优化技巧,帮助开发者提高程序的效率。一、使用BufferedReader和BufferedWriter类Java提供了BufferedReader和BufferedWr
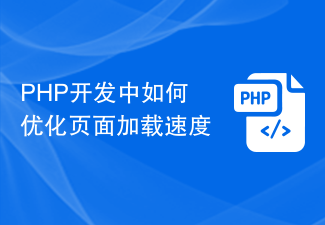
随着互联网技术的不断发展,对于网站页面加载速度的要求也越来越高,而作为一名PHP开发人员,我们需要了解一些优化方法以确保页面快速加载并提高用户体验。下面将为您介绍几种常见的PHP页面优化技巧。使用缓存缓存是一种将数据存储在临时存储器中以便快速访问的技术。在PHP中,我们可以使用Memcached和Redis等内存缓存系统,将经常使用的页面
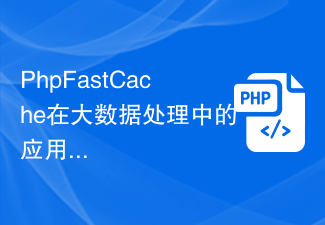
PhpFastCache在大数据处理中的应用实践引言:在当今大数据时代,数据处理变得越来越重要和复杂。在处理大数据集时,我们需要优化算法和减少读写数据的时间。PhpFastCache是一个功能强大并且易于使用的缓存解决方案,它可以有效地提高数据处理的性能。在本文中,我们将介绍PhpFastCache的基本概念和使用方法,并通过示例代码演示其在大数据处理中的应
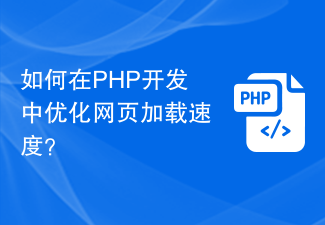
如何在PHP开发中优化网页加载速度?随着互联网的快速发展,网页加载速度对用户体验变得越来越重要。当网页加载速度慢时,用户往往会选择关闭页面或者离开网站。因此,对于PHP开发者来说,优化网页加载速度是一个非常重要的任务。下面将介绍一些优化网页加载速度的方法。1.使用缓存在PHP开发中,使用缓存是提高网页加载速度的最简单和最有效的方法之一。可以使用各种缓存技术,
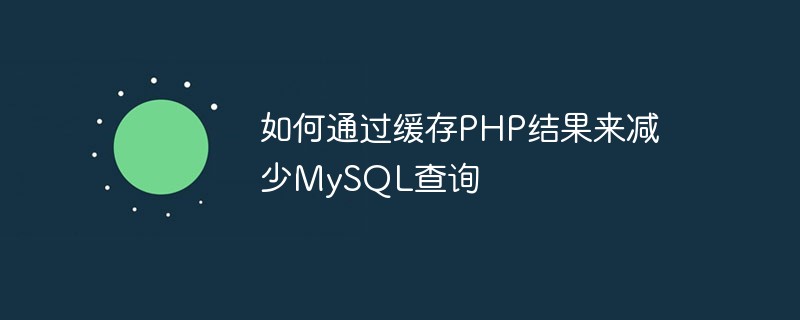
随着网站访问量的增加,MySQL数据库查询变得越来越频繁,响应速度逐渐变慢,导致用户体验变差。为了提高网站的性能,可以通过缓存PHP结果来减少MySQL查询,达到优化数据库的目的。一、缓存介绍缓存是一种存储介质,用于存储计算结果,以便未来使用。由于计算的结果被保存,因此可以在以后的使用中快速访问结果,而不必重新计算。在Web开发中,缓存可以帮助
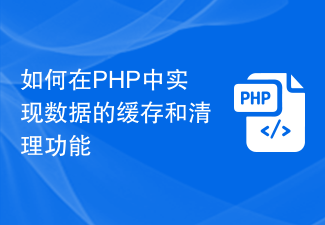
如何在PHP中实现数据的缓存和清理功能,需要具体代码示例缓存是在Web开发中常见的优化策略之一,可以提高网站的性能和响应速度。在PHP中,我们可以使用各种方法来实现数据的缓存和清理功能,本文将介绍几种常用的方法,并提供具体的代码示例。一、使用PHP原生的文件缓存PHP原生提供了一种简单的文件缓存方法,可以将数据存储在文件中,以减少数据库或其他资源的访问。以下
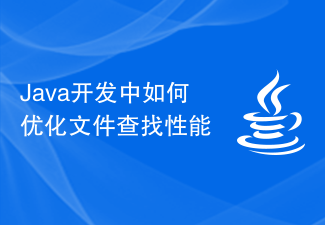
Java是一种广泛使用的编程语言,在软件开发中应用广泛。在许多应用程序中,文件查找是一个常见的操作,并且文件查找的性能对于应用程序的运行速度有重要影响。因此,在Java开发中,优化文件查找性能是一个关键的问题。文件查找是指在文件系统中查找特定文件的操作。在Java中,可以使用File类提供的方法来实现文件查找功能。但是,简单地使用File的方法可能会导致性能


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
