How to design an efficient PHP message queue system
With the development of the Internet, the scale of applications continues to expand, and the demand for data processing is also getting higher and higher. The traditional synchronous request method cannot meet the high concurrency requirements. At this time, message queue has become a very effective solution. Message queue is a way of application decoupling, which can transfer data between different components, reduce dependencies between systems, and improve application performance and scalability.
This article will introduce how to use PHP to design an efficient message queue system. We will use Redis as the storage engine of the message queue for demonstration. Redis is a high-performance Key-Value storage system that supports rich data structures and built-in publish/subscribe mechanisms, making it very suitable as a message queue engine.
First, we need to install Redis on the server. You can use the following command to install:
apt-get install redis-server
After the installation is complete, you can start the Redis service through the following command:
service redis-server start
Next, we use Composer to install the PHP Redis extension. Execute the following command in the terminal:
composer require predis/predis
After the installation is complete, we can start writing PHP code to implement the message queue system.
First, we need to create a Producer class to publish messages. The message can be any PHP serialized data, such as strings, arrays, objects, etc. The following is a simple Producer class. Each time the pushMessage
method is called, the message will be pushed to the Redis queue:
<?php require 'vendor/autoload.php'; class Producer { private $redis; public function __construct() { $this->redis = new PredisClient(); } public function pushMessage($channel, $message) { $this->redis->rpush($channel, serialize($message)); } } $producer = new Producer(); $producer->pushMessage('channel1', 'Hello, World!');
Next, we need to create a Consumer class to process the message. The Consumer class needs to continuously monitor the Redis queue and retrieve messages from the queue for processing. The following is an example of a simple Consumer class:
<?php require 'vendor/autoload.php'; class Consumer { private $redis; public function __construct() { $this->redis = new PredisClient(); } public function processMessage($channel, $callback) { while (true) { $message = $this->redis->blpop($channel, 0)[1]; $callback(unserialize($message)); } } } $consumer = new Consumer(); $consumer->processMessage('channel1', function($message) { echo "Received message: " . $message . PHP_EOL; });
In the above example, the processMessage
method of the Consumer class uses Redis's blpop
command to obtain the queue blockingly message in the message, and then call the callback function to process the message. This enables asynchronous processing of consumers.
Finally, we can use the Producer class to publish messages and the Consumer class to process messages in the application. According to specific needs, more Producers and Consumers can be built to build a more complex message queue system.
Summary
By using Redis as the storage engine of the message queue, combined with PHP's Predis extension, we can easily implement an efficient PHP message queue system. Message queues can effectively decouple the various components of an application and improve application performance and scalability.
The above is the detailed content of How to design an efficient PHP message queue system. For more information, please follow other related articles on the PHP Chinese website!
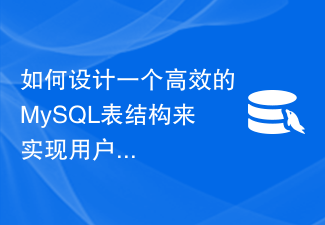
如何设计一个高效的MySQL表结构来实现用户管理功能?为了实现用户管理功能,我们需要在数据库中设计一张用户表来存储用户相关信息,如用户名、密码、邮箱等。下面将逐步介绍如何设计高效的MySQL表结构来实现用户管理功能。一、创建用户表首先,我们需要创建一个用户表来存储用户的相关信息。在MySQL中,可以使用CREATETABLE语句来创建表,如下:CREATE
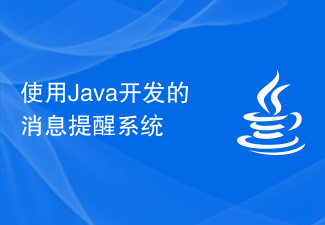
使用Java开发的消息提醒系统摘要:消息提醒系统是当我们需要在特定时间或特定条件下发送消息给用户时非常常见的功能。本文将介绍如何通过使用Java语言开发一个简单的消息提醒系统,并提供相应的代码示例。项目背景在很多应用程序中,我们通常需要进行消息提醒的功能,比如定时发送提醒、事件触发提醒等。这些提醒功能可以通过短信、邮件或者手机推送实现。为了使这些提醒功能更加
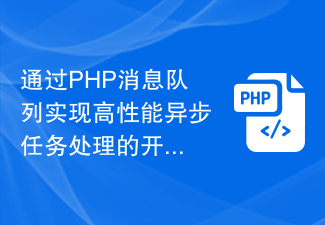
通过PHP消息队列实现高性能异步任务处理的开发方法随着互联网的快速发展,各种网站和应用程序的性能要求也越来越高。在实际开发中,有很多情况下需要处理一些耗时任务,例如发送大量邮件、生成报表等,这些任务可能会大大降低网站的性能,甚至导致服务器资源耗尽。为了解决这个问题,我们可以使用消息队列来实现任务的异步处理。消息队列是一种基于生产者-消费者模型的通信方式,生产
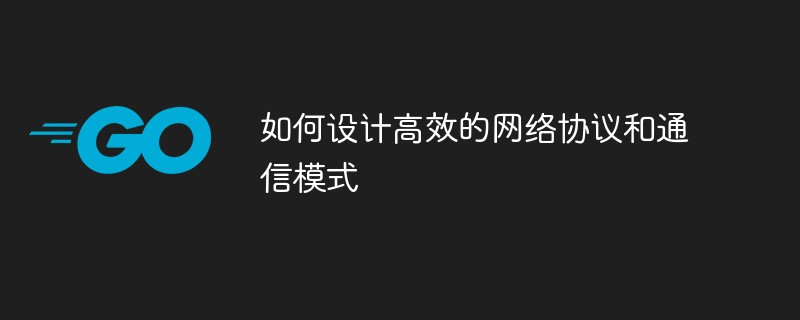
网络协议和通信模式是保障网络正常运行的关键要素。不论是在企业内部局域网的构建,还是互联网世界的互通,网络协议和通信模式都发挥着非常大的作用。要设计高效的网络协议和通信模式,有以下几个方面需要考虑和关注:一、充分理解网络通信的基础知识网络通信的基础知识包括传输协议、数据包格式、流量控制等方面。对于不同的数据传输形式,如实时音视频、文件传输等应用场景,需要选择适
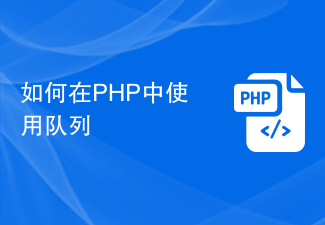
随着互联网技术的不断发展,Web应用程序的功能需求越来越复杂,处理海量数据和高并发访问已成为了一个司空见惯的应用场景。在这样的背景下,队列的应用变得越来越普遍。队列是一种简单、高效的数据结构,在处理大量数据和任务时具有显著优势。队列的本质是一种基于先进先出(FIFO)原则的数据结构。生产者将任务放入队列中,然后消费者从队列中取出任务并进行处理。这个过程
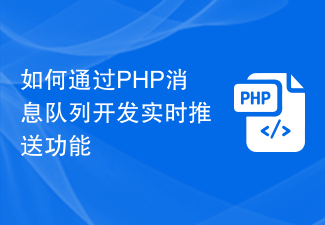
如何通过PHP消息队列开发实时推送功能随着互联网的发展,实时推送成为了许多网站和应用程序的重要功能之一。通过实时推送,网站和应用程序可以在服务器端有数据更新时即时地将新数据推送给客户端。这种实时推送功能可以提升用户体验,使用户能够及时了解到最新的信息。在开发实时推送功能时,PHP消息队列是一种常用的技术。消息队列是一种可以存储和传递消息的机制,它使得不同的应
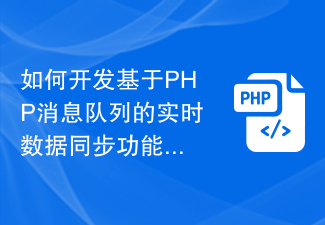
如何开发基于PHP消息队列的实时数据同步功能摘要:随着互联网应用的快速发展,服务器端实时数据同步功能变得越来越重要。本文介绍了基于PHP消息队列的实时数据同步功能的开发方法。首先,介绍消息队列的基本概念和工作原理。然后,详细介绍如何在PHP中使用消息队列实现实时数据同步功能。最后,给出了一些优化和扩展的建议,以提高实时数据同步功能的性能和可靠性。一、引言随着
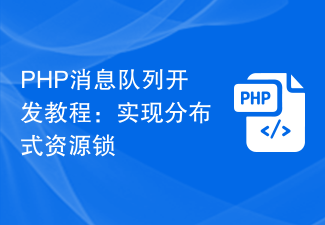
PHP消息队列开发教程:实现分布式资源锁引言:随着互联网技术的快速发展,分布式系统在企业级应用中的广泛应用成为了趋势。在分布式系统中,如何实现资源的合理调度和管理是一个重要的问题。本文将介绍如何使用PHP消息队列来实现分布式资源锁,以满足分布式系统中资源管理的需求。一、什么是分布式资源锁分布式资源锁是指对分布式系统中的资源进行加锁控制,保证同一时间只能有一个


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
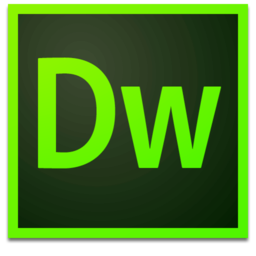
Dreamweaver Mac version
Visual web development tools
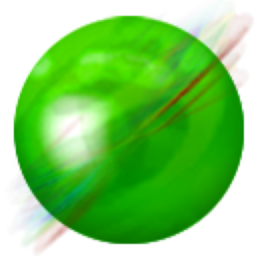
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
