


PHP Amazon API development: how to handle product classification and tags
PHP Amazon API development: How to deal with product classification and tags
Introduction:
Amazon is one of the largest e-commerce platforms in the world. Its API (Application Programming Interface) allows developers to program through ways to interact with its platform. Handling product classification and tags is a very important step when developing an application based on Amazon API. This article will introduce how to use PHP language to process Amazon product classification and tags, and attach relevant code examples.
1. Obtain product classification
- First, we need to use the Product Advertising API provided by Amazon to obtain product classification information. Before using the API, you need to register a developer account on the Amazon Developer Platform and create an API key.
- In PHP, we can use the curl library to send HTTP requests to interact with the Amazon API. The following is a simple code example that shows how to use the Amazon API to obtain product classification information:
<?php $access_key = "YOUR_ACCESS_KEY"; $secret_key = "YOUR_SECRET_KEY"; $associate_tag = "YOUR_ASSOCIATE_TAG"; $base_url = "http://webservices.amazon.com/onca/xml"; $params = array( "Service" => "AWSECommerceService", "Operation" => "BrowseNodeLookup", "BrowseNodeId" => "0", // 根菜单分类 "ResponseGroup" => "BrowseNodes", "AssociateTag" => $associate_tag, "AWSAccessKeyId" => $access_key, ); $canonical_query_string = http_build_query($params); $signature = base64_encode(hash_hmac("sha256", "GET webservices.amazon.com /onca/xml " . $canonical_query_string, $secret_key, true)); $request_url = $base_url . "?" . $canonical_query_string . "&Signature=" . urlencode($signature); // 发送HTTP请求并获取响应 $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $request_url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); // 解析响应XML并提取商品分类信息 $xml = simplexml_load_string($response); $browse_nodes = $xml->xpath('//BrowseNode'); foreach ($browse_nodes as $browse_node) { $node_id = (string)$browse_node->BrowseNodeId; $name = (string)$browse_node->Name; // 打印分类信息 echo "分类ID: " . $node_id . ", 分类名称: " . $name . " "; } ?>
In the above code, we obtain product classification information by sending a BrowseNodeLookup request to the API. YOUR_ACCESS_KEY
, YOUR_SECRET_KEY
, and YOUR_ASSOCIATE_TAG
need to be replaced with your own Amazon API key and associated tag.
2. Obtain product tags
- Similarly, we can also use Amazon API to obtain product tag information. The following is a sample code that shows how to use PHP to obtain product tags:
<?php $access_key = "YOUR_ACCESS_KEY"; $secret_key = "YOUR_SECRET_KEY"; $associate_tag = "YOUR_ASSOCIATE_TAG"; $base_url = "http://webservices.amazon.com/onca/xml"; $params = array( "Service" => "AWSECommerceService", "Operation" => "ItemLookup", "ItemId" => "B00EOE0WKQ", // 商品ASIN码 "ResponseGroup" => "ItemAttributes", "AssociateTag" => $associate_tag, "AWSAccessKeyId" => $access_key, ); $canonical_query_string = http_build_query($params); $signature = base64_encode(hash_hmac("sha256", "GET webservices.amazon.com /onca/xml " . $canonical_query_string, $secret_key, true)); $request_url = $base_url . "?" . $canonical_query_string . "&Signature=" . urlencode($signature); // 发送HTTP请求并获取响应 $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $request_url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); // 解析响应XML并提取商品标签信息 $xml = simplexml_load_string($response); $tags = $xml->xpath('//ItemAttributes/Feature'); foreach ($tags as $tag) { // 打印商品标签 echo "标签: " . (string)$tag . " "; } ?>
In the above code, we obtain the product tag by sending an ItemLookup request to the API and passing in the ASIN code of the product. YOUR_ACCESS_KEY
, YOUR_SECRET_KEY
, and YOUR_ASSOCIATE_TAG
need to be replaced with your own Amazon API key and associated tag.
Conclusion:
Handling product classification and labeling is an important task when developing applications based on Amazon API. By using PHP language, we can easily interact with Amazon API and get the required classification and tag information. The above provides code examples for processing product classification and labeling. I hope it can help you practice in Amazon API development.
The above is the detailed content of PHP Amazon API development: how to handle product classification and tags. For more information, please follow other related articles on the PHP Chinese website!
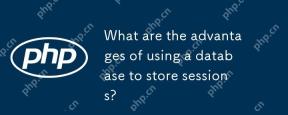
The main advantages of using database storage sessions include persistence, scalability, and security. 1. Persistence: Even if the server restarts, the session data can remain unchanged. 2. Scalability: Applicable to distributed systems, ensuring that session data is synchronized between multiple servers. 3. Security: The database provides encrypted storage to protect sensitive information.
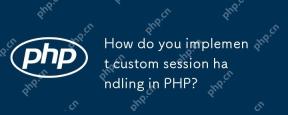
Implementing custom session processing in PHP can be done by implementing the SessionHandlerInterface interface. The specific steps include: 1) Creating a class that implements SessionHandlerInterface, such as CustomSessionHandler; 2) Rewriting methods in the interface (such as open, close, read, write, destroy, gc) to define the life cycle and storage method of session data; 3) Register a custom session processor in a PHP script and start the session. This allows data to be stored in media such as MySQL and Redis to improve performance, security and scalability.
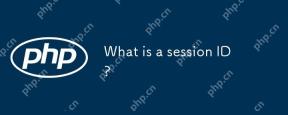
SessionID is a mechanism used in web applications to track user session status. 1. It is a randomly generated string used to maintain user's identity information during multiple interactions between the user and the server. 2. The server generates and sends it to the client through cookies or URL parameters to help identify and associate these requests in multiple requests of the user. 3. Generation usually uses random algorithms to ensure uniqueness and unpredictability. 4. In actual development, in-memory databases such as Redis can be used to store session data to improve performance and security.
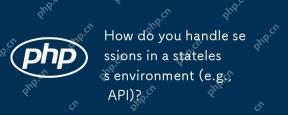
Managing sessions in stateless environments such as APIs can be achieved by using JWT or cookies. 1. JWT is suitable for statelessness and scalability, but it is large in size when it comes to big data. 2.Cookies are more traditional and easy to implement, but they need to be configured with caution to ensure security.
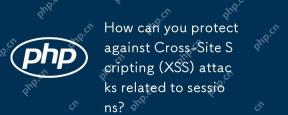
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
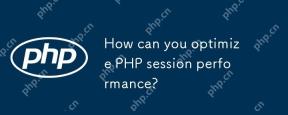
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
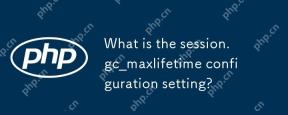
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
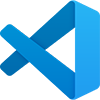
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use
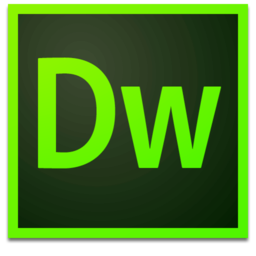
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
