


Learn Python to implement Qiniu Cloud interface docking and image conversion function
Learn Python to implement Qiniu Cloud interface docking and realize image conversion function
Introduction:
With the development of the Internet, pictures play a very important role in our daily lives. In website development, image conversion is a common requirement, such as image scaling, cropping, or format conversion. Qiniu Cloud is a well-known cloud storage service provider in China, which provides powerful and stable image processing functions. This article will introduce how to use Python language to connect to the interface of Qiniu Cloud to realize the image conversion function.
1. Preparation:
- Register a Qiniu Cloud account and create a storage space.
- Install Python's requests library for sending HTTP requests.
- Get the AccessKey and SecretKey of Qiniu Cloud Storage Space.
2. Import dependent libraries:
To use the requests library to send HTTP requests in a Python project, we need to import the requests library first in the code.
import requests
3. Obtain Qiniu Cloud's upload certificate:
Before uploading images, we need to obtain an upload certificate first. Qiniu Cloud's upload certificate is a token used to upload files and is used to verify the legality of the upload behavior. The following code demonstrates how to obtain upload credentials through Qiniu Cloud's API.
access_key = 'your_access_key' # 七牛云的AccessKey secret_key = 'your_secret_key' # 七牛云的SecretKey bucket_name = 'your_bucket_name' # 存储空间名称 def get_upload_token(access_key, secret_key, bucket_name): url = 'http://api.qiniu.com/put-policy/{}/put-policy'.format(bucket_name) auth = requests.auth.HTTPBasicAuth(access_key, secret_key) response = requests.get(url, auth=auth) result = response.json() if 'token' in result: return result['token'] else: raise ValueError('Failed to get upload token.') upload_token = get_upload_token(access_key, secret_key, bucket_name)
4. Upload image files:
After obtaining the upload credentials, we can start uploading image files. In Qiniu Cloud, we can use a custom key to identify uploaded file resources. The following code demonstrates how to use Python language to upload image files to Qiniu Cloud.
def upload_image(file_path, upload_token): url = 'http://upload.qiniu.com/' headers = { 'Content-Type': 'multipart/form-data', } files = {'file': open(file_path, 'rb')} data = {'token': upload_token} response = requests.post(url, headers=headers, files=files, data=data) result = response.json() if 'key' in result: return result['key'] else: raise ValueError('Failed to upload image.') image_path = 'your_image_path' # 待上传的图片文件路径 image_key = upload_image(image_path, upload_token)
5. Perform image conversion operations:
After uploading the image file successfully, we can perform various conversion operations on the image through Qiniu Cloud's API. Qiniu Cloud provides many powerful image processing functions, such as image scaling, cropping, format conversion, etc. The following code demonstrates how to use Python language to call Qiniu Cloud's interface to achieve image scaling and format conversion.
def image_tranformation(image_key, new_image_key, width, height, format): url = 'http://api.qiniu.com/image/v2/{}'.format(image_key) headers = { 'Content-Type': 'application/x-www-form-urlencoded', } params = { 'imageView2': '/{}.w_{}/h_{}/format/{}'.format(new_image_key, width, height, format), } response = requests.get(url, headers=headers, params=params) with open(new_image_key, 'wb') as f: f.write(response.content) new_image_key = 'your_new_image_key' # 新生成的图片文件key width = 500 # 新图片的宽度 height = 500 # 新图片的高度 format = 'jpg' # 新图片的格式 image_tranformation(image_key, new_image_key, width, height, format)
6. Summary:
This article introduces how to use Python language to connect to the Qiniu Cloud interface to realize the image conversion function. By studying this article, you can master how to use Python language and Qiniu Cloud's API to upload and convert images. I hope this article can be helpful to you when using Qiniu Cloud for image processing.
The above is the detailed content of Learn Python to implement Qiniu Cloud interface docking and image conversion function. For more information, please follow other related articles on the PHP Chinese website!
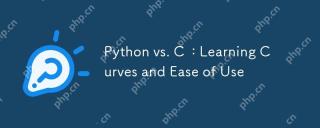
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
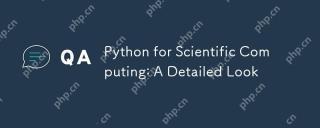
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
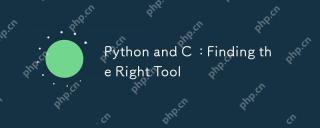
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
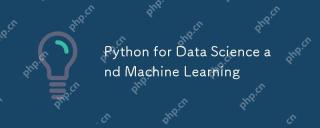
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
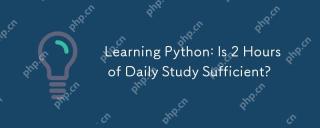
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
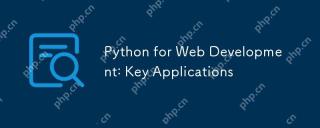
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
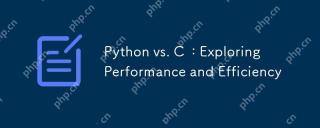
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
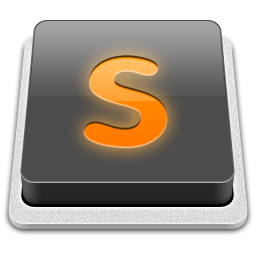
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.