How to use PHP to implement recursive algorithms
Introduction:
Recursion is a very important algorithmic idea that is often used in programming. As a scripting language widely used in web development, PHP can also support recursive algorithms well. This article will introduce in detail how to implement recursive algorithms using PHP and give some practical code examples.
1. What is recursive algorithm
Recursion refers to a technique that calls the function itself in the definition of the function. Simply put, it is the process of a function calling itself. The recursive algorithm is based on the idea of recursive definition in the problem solving process. Each recursion is a smaller-scale solution to the same problem based on this problem.
2. Basic elements of recursive algorithm
When using PHP to implement a recursive algorithm, you need to consider the following basic elements:
1. Termination condition: The recursive algorithm must have a termination conditions, otherwise an infinite loop will occur.
2. Recursive calls: In recursive algorithms, the function needs to call itself to solve the same problem on a smaller scale.
3. Problem decomposition: Recursive algorithms usually decompose the problem into smaller-scale identical problems to solve.
3. Use PHP to implement recursive algorithms
Below we use several specific examples to illustrate how to use PHP to implement recursive algorithms.
1. Calculate factorial
Factorial refers to the product of all integers from 1 to a given number. The calculation of factorial can be easily realized through recursive algorithm.
function factorial($n) { if ($n <= 1) { return 1; // 终止条件 } return $n * factorial($n-1); // 递归调用 } echo factorial(5); // 输出120
2. Fibonacci Sequence
The Fibonacci Sequence means that starting from the 3rd number, each number is the sum of the previous two numbers. The calculation of Fibonacci sequence can be easily realized through recursive algorithm.
function fib($n) { if ($n <= 1) { return $n; // 终止条件 } return fib($n-1) + fib($n-2); // 递归调用 } echo fib(6); // 输出8
3. Solving the number of combinations
The number of combinations refers to the number of non-repeating combinations of $k$ elements selected from $n$ elements, which can be solved by a recursive algorithm.
function combination($n, $k) { if ($k == 0 || $k == $n) { return 1; // 终止条件 } return combination($n-1, $k-1) + combination($n-1, $k); // 递归调用 } echo combination(5, 2); // 输出10
4. Summary
Recursive algorithm is an important algorithm idea and can also be well supported in PHP. By properly designing the termination conditions, recursive calls and problem decomposition of recursive functions, we can easily implement various recursive algorithms. I hope this article can help readers understand and master the recursive algorithm in PHP.
References:
[1] Deng Junhui. Data Structure and Algorithm. Tsinghua University Press, 2018.
The above is the detailed content of How to implement recursive algorithm in PHP. For more information, please follow other related articles on the PHP Chinese website!
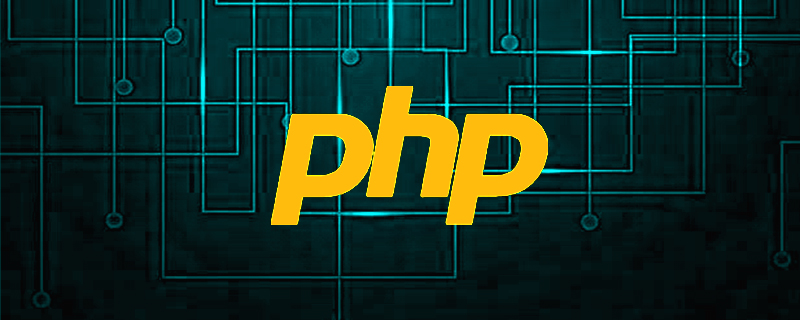
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
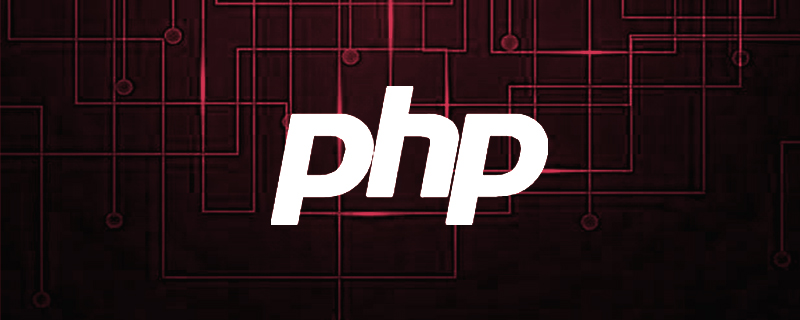
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
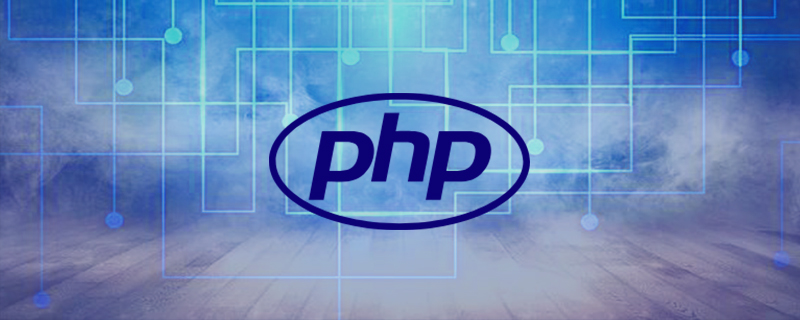
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
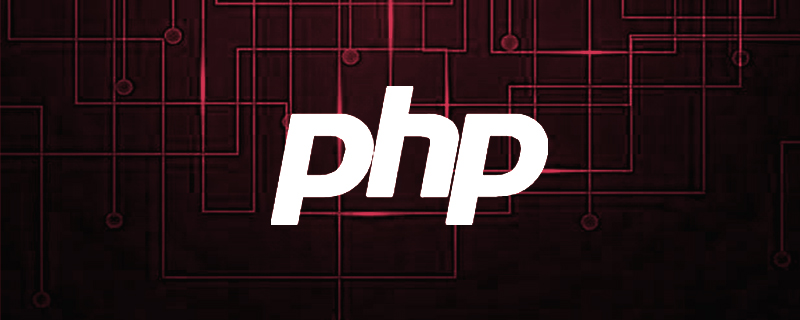
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
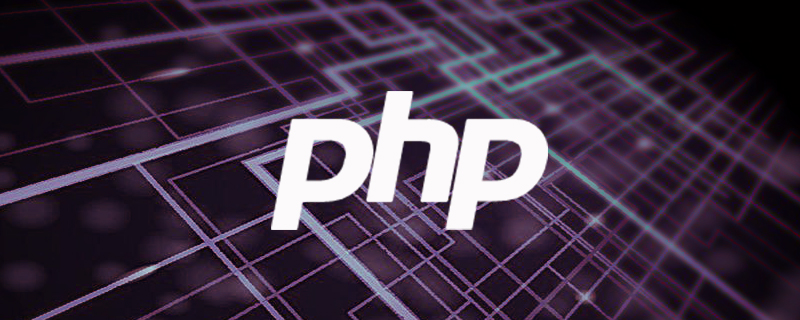
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
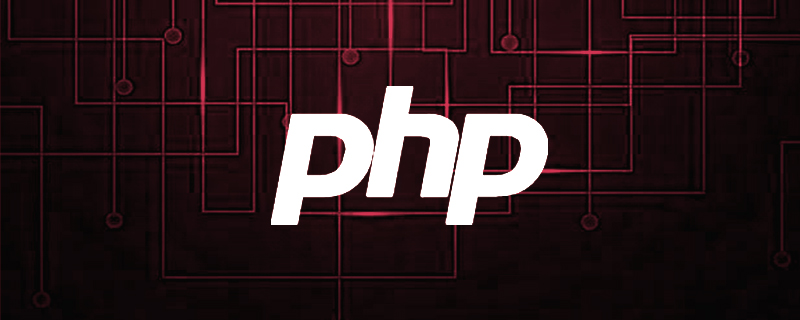
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
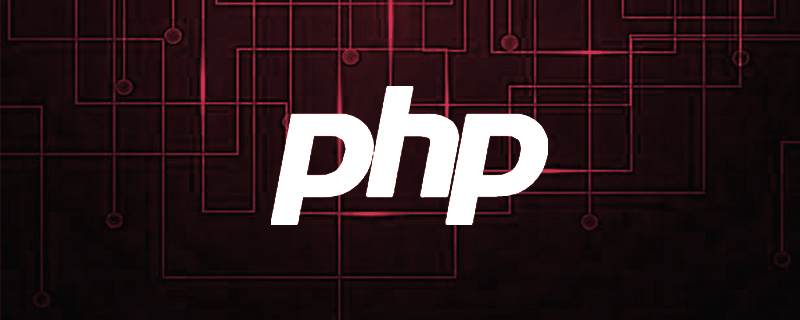
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
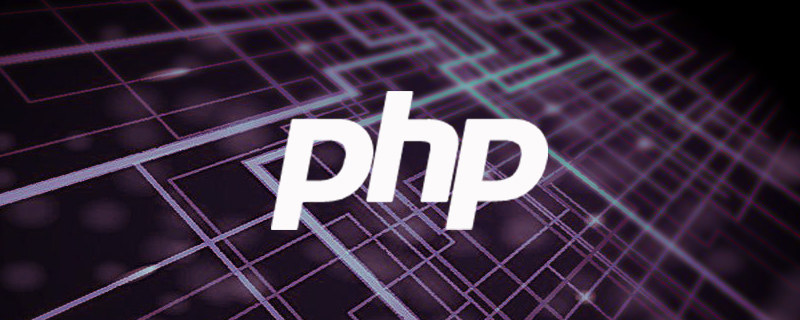
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
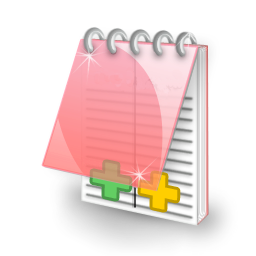
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!
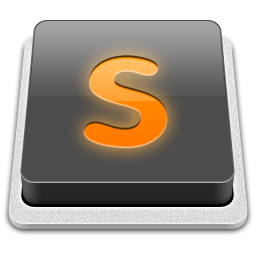
SublimeText3 Mac version
God-level code editing software (SublimeText3)
