


How to detect and fix security issues with web pages using PHP and the WebDriver extension
如何使用PHP和WebDriver扩展检测和修复网页的安全问题
导言:随着互联网的快速发展,网页安全问题日益凸显,如SQL注入、跨站脚本攻击等,给用户和网站运营者带来了巨大的安全风险。为了保障网站的安全性,我们可以利用PHP和WebDriver扩展来检测和修复网页的安全问题。本文将介绍如何使用这两个工具来对网页进行安全检测和修复。
一、PHP的安全检测功能
PHP作为一种高级的服务器端脚本语言,具有许多安全检测的工具和函数,我们可以使用这些工具和函数来检测和修复网页的安全问题。
- 防止SQL注入
SQL注入是最常见的网页安全问题之一。为了防止SQL注入,我们可以使用PHP的预处理语句(prepared statement)来过滤用户输入的数据。
以下是一个使用预处理语句防止SQL注入的示例代码:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检测连接 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 使用预处理语句来查询数据库 $sql = "SELECT * FROM users WHERE username = ?"; $stmt = $conn->prepare($sql); $stmt->bind_param("s", $username); // 执行查询 $stmt->execute(); $result = $stmt->get_result(); // 处理查询结果 while ($row = $result->fetch_assoc()) { echo "用户名: " . $row["username"]. "<br>"; } // 关闭连接 $stmt->close(); $conn->close(); ?>
- 防止跨站脚本攻击(XSS)
跨站脚本攻击是另一个常见的网页安全问题。为了防止XSS攻击,我们可以使用PHP的htmlspecialchars函数来对用户输入的数据进行转义。
以下是一个使用htmlspecialchars函数防止XSS攻击的示例代码:
<?php // 检测输入 if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = htmlspecialchars($_POST["name"]); $email = htmlspecialchars($_POST["email"]); $comment = htmlspecialchars($_POST["comment"]); } ?>
二、WebDriver扩展的使用
WebDriver是一个用于Web应用程序自动化的API。我们可以使用WebDriver来模拟用户的行为,包括访问网页、填写表单等,通过检测和模拟用户行为,可以发现网页中的安全问题。
以下是一个使用WebDriver扩展进行安全检测的示例代码:
<?php require_once 'vendor/autoload.php'; use FacebookWebDriver; use PHPUnitFrameworkTestCase; class SecurityTest extends TestCase { protected $driver; protected function setUp(): void { $options = new WebDriverChromeChromeOptions(); $options->addArguments(['--headless']); // 在后台运行浏览器 $capabilities = WebDriverRemoteDesiredCapabilities::chrome(); $capabilities->setCapability(WebDriverChromeChromeOptions::CAPABILITY, $options); $this->driver = WebDriverRemoteRemoteWebDriver::create('http://localhost:9515', $capabilities); } public function testSQLInjection() { $this->driver->get('http://example.com/login'); $usernameInput = $this->driver->findElement(WebDriverWebDriverBy::name('username')); $passwordInput = $this->driver->findElement(WebDriverWebDriverBy::name('password')); $usernameInput->sendKeys("admin' OR '1'='1"); $passwordInput->sendKeys("password"); $submitButton = $this->driver->findElement(WebDriverWebDriverBy::tagName('button')); $submitButton->click(); $errorMessage = $this->driver->findElement(WebDriverWebDriverBy::className('error-message'))->getText(); $this->assertEquals("用户名或密码错误", $errorMessage); } public function testXSSAttack() { $this->driver->get('http://example.com/comment'); $commentInput = $this->driver->findElement(WebDriverWebDriverBy::name('comment')); $commentInput->sendKeys("<script>alert('XSS Attack')</script>"); $submitButton = $this->driver->findElement(WebDriverWebDriverBy::tagName('button')); $submitButton->click(); $commentText = $this->driver->findElement(WebDriverWebDriverBy::className('comment-text'))->getText(); $this->assertEquals("<script>alert('XSS Attack')</script>", $commentText); } protected function tearDown(): void { $this->driver->quit(); } } ?>
以上示例代码演示了如何使用WebDriver扩展来模拟用户输入,并对网页的安全性进行检测。
结语:通过使用PHP和WebDriver扩展,我们可以有效地检测和修复网页的安全问题。在开发和维护网页时,应始终注意用户输入的安全性,并采取适当的措施来防止潜在的安全威胁。
The above is the detailed content of How to detect and fix security issues with web pages using PHP and the WebDriver extension. For more information, please follow other related articles on the PHP Chinese website!
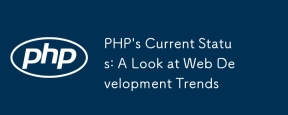
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
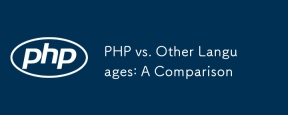
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
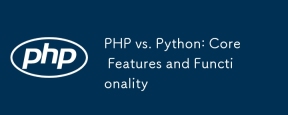
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
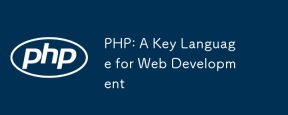
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
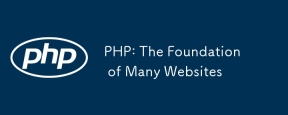
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
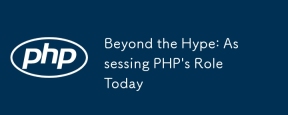
PHP remains a powerful and widely used tool in modern programming, especially in the field of web development. 1) PHP is easy to use and seamlessly integrated with databases, and is the first choice for many developers. 2) It supports dynamic content generation and object-oriented programming, suitable for quickly creating and maintaining websites. 3) PHP's performance can be improved by caching and optimizing database queries, and its extensive community and rich ecosystem make it still important in today's technology stack.
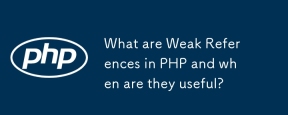
In PHP, weak references are implemented through the WeakReference class and will not prevent the garbage collector from reclaiming objects. Weak references are suitable for scenarios such as caching systems and event listeners. It should be noted that it cannot guarantee the survival of objects and that garbage collection may be delayed.
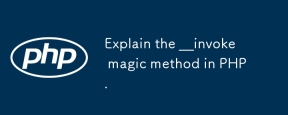
The \_\_invoke method allows objects to be called like functions. 1. Define the \_\_invoke method so that the object can be called. 2. When using the $obj(...) syntax, PHP will execute the \_\_invoke method. 3. Suitable for scenarios such as logging and calculator, improving code flexibility and readability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor