


Using Python and WebDriver to implement automatic filling of date pickers on web pages
Using Python and WebDriver to implement a web page auto-fill date picker
Introduction:
In modern web applications, date pickers are very common and users need to select dates manually. However, for some scenarios such as automated testing and data collection, we need to automatically populate the date picker programmatically. This article will introduce how to use Python and WebDriver to implement the function of automatically filling a date picker.
1. Preparation:
First, we need to install Python and WebDriver drivers. Here we use the Selenium library to implement WebDriver operations.
- Install Python: Go to the Python official website (https://www.python.org/downloads/) to download and install the latest version of Python.
-
Install Selenium: Use the pip command to install the Selenium library. Open the command line interface and run the following command:
pip install selenium
- Download the WebDriver driver: WebDriver is a tool used to control the browser. Different browsers require different drivers. For example, if you use the Chrome browser, you need to download and install ChromeDriver (download address: https://sites.google.com/a/chromium.org/chromedriver/). Make sure to add the path to the driver to your system environment variables.
2. Write code:
We use Python and Selenium to write the code to automatically fill in the date picker. The specific steps are as follows:
-
Introduce the required libraries and classes:
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC from selenium.webdriver.common.keys import Keys
-
Create the WebDriver object and open the Chrome browser:
driver = webdriver.Chrome()
-
Navigate to the target page:
driver.get("http://example.com")
-
Locate the date picker element and click to open the picker:
date_input = driver.find_element(By.ID, "date-input") date_input.click()
-
Wait for the date picker to appear and locate the date element of the picker:
date_picker = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.CSS_SELECTOR, ".date-picker")) ) date_elements = date_picker.find_elements(By.CSS_SELECTOR, ".date-element")
-
Fill the value of the date picker:
date_to_select = "2022-01-01" for date_element in date_elements: if date_element.text == date_to_select: date_element.click() break
-
Close the browser:
driver.quit()
3. Complete example:
The following is a complete example code that demonstrates how to automatically fill a date picker using Python and WebDriver:
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC def fill_date_picker(url, date_input_id, date_to_select): driver = webdriver.Chrome() driver.get(url) date_input = driver.find_element(By.ID, date_input_id) date_input.click() date_picker = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.CSS_SELECTOR, ".date-picker")) ) date_elements = date_picker.find_elements(By.CSS_SELECTOR, ".date-element") for date_element in date_elements: if date_element.text == date_to_select: date_element.click() break driver.quit() if __name__ == "__main__": url = "http://example.com" date_input_id = "date-input" date_to_select = "2022-01-01" fill_date_picker(url, date_input_id, date_to_select)
Summary:
This article briefly introduces how to use Python and WebDriver to implement the function of automatically filling the date picker on a web page. With the support of the Selenium library, we can easily implement automated date picker operations, improving programming efficiency and accuracy. I hope this article can be helpful for using Python and WebDriver to implement the function of automatically filling in date pickers on web pages.
Note:
When using WebDriver, you must pay attention to comply with the usage regulations and laws and regulations of the relevant websites, and respect user privacy. Keep your WebDriver version updated to ensure optimal compatibility and security.
The above is the detailed content of Using Python and WebDriver to implement automatic filling of date pickers on web pages. For more information, please follow other related articles on the PHP Chinese website!
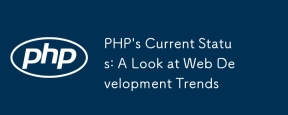
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
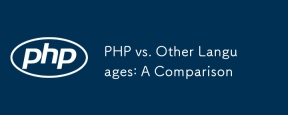
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
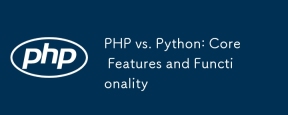
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
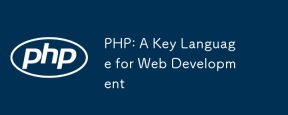
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
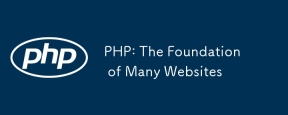
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
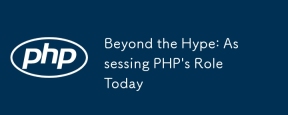
PHP remains a powerful and widely used tool in modern programming, especially in the field of web development. 1) PHP is easy to use and seamlessly integrated with databases, and is the first choice for many developers. 2) It supports dynamic content generation and object-oriented programming, suitable for quickly creating and maintaining websites. 3) PHP's performance can be improved by caching and optimizing database queries, and its extensive community and rich ecosystem make it still important in today's technology stack.
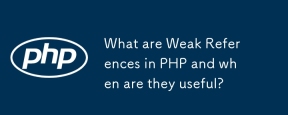
In PHP, weak references are implemented through the WeakReference class and will not prevent the garbage collector from reclaiming objects. Weak references are suitable for scenarios such as caching systems and event listeners. It should be noted that it cannot guarantee the survival of objects and that garbage collection may be delayed.
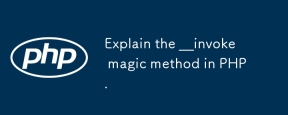
The \_\_invoke method allows objects to be called like functions. 1. Define the \_\_invoke method so that the object can be called. 2. When using the $obj(...) syntax, PHP will execute the \_\_invoke method. 3. Suitable for scenarios such as logging and calculator, improving code flexibility and readability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
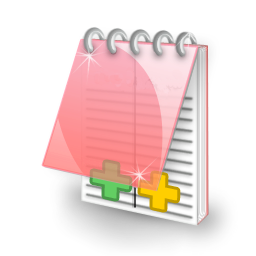
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use