


Build a real-time audio and video communication system using Alibaba Cloud SDK and Java
Using Alibaba Cloud SDK and Java to build a real-time audio and video communication system
In recent years, with the rapid development of the Internet, real-time audio and video communication has become an indispensable part of people's lives. Whether it is social entertainment, online education or remote working, real-time audio and video communication can provide a high-quality, low-latency communication experience. This article will introduce how to use Alibaba Cloud SDK and Java to build a real-time audio and video communication system, and provide corresponding code examples.
1. Preparation
Before starting, we need to ensure that we have registered an Alibaba Cloud account and activated the real-time audio and video service. You can create an application through the Alibaba Cloud console and obtain the application ID and application key. This information will be used in subsequent code.
2. Introduction of Alibaba Cloud SDK
We can use Maven or Gradle and other build tools to introduce the dependencies of Alibaba Cloud SDK. The following is an example of using Maven:
<dependency> <groupId>com.aliyun</groupId> <artifactId>aliyun-java-sdk-rts</artifactId> <version>1.0.0</version> </dependency>
3. Create a real-time audio and video call
Before making a real-time audio and video call, we need to create a call session. First, we need to initialize RTSClient and set the corresponding Region, AccessKey and other information. The code example is as follows:
import com.aliyun.rts.RTSClient; import com.aliyun.rts.model.CreateSessionRequest; import com.aliyun.rts.model.CreateSessionResult; public class CreateSessionDemo { public static void main(String[] args) { String accessKeyId = "your-access-key-id"; String accessKeySecret = "your-access-key-secret"; String regionId = "cn-hangzhou"; RTSClient client = new RTSClient(accessKeyId, accessKeySecret, regionId); CreateSessionRequest request = new CreateSessionRequest(); request.setSessionType("1v1_audio"); // 设置会话类型为1v1音频通话 CreateSessionResult result = client.createSession(request); if (result.isSuccess()) { String sessionId = result.getSessionId(); String sessionKey = result.getSessionKey(); System.out.println("创建会话成功,会话ID:" + sessionId); System.out.println("会话密钥:" + sessionKey); } else { System.out.println("创建会话失败,错误信息:" + result.getErrorMessage()); } } }
In the above code, we first create an RTSClient instance and set the corresponding AccessKey and Region. Next, we created a CreateSessionRequest instance and set the session type to 1v1 audio call. Then, we call RTSClient's createSession method to create a session, and what is returned is a CreateSessionResult object. If the session is created successfully, we can get the session ID and session key from the CreateSessionResult object.
4. Join the audio and video call
After creating the session, we can join the audio and video call through the session ID and session key. The following is an example of joining an audio call:
import com.aliyun.rts.RTSClient; import com.aliyun.rts.model.JoinSessionRequest; import com.aliyun.rts.model.JoinSessionResult; public class JoinSessionDemo { public static void main(String[] args) { String accessKeyId = "your-access-key-id"; String accessKeySecret = "your-access-key-secret"; String regionId = "cn-hangzhou"; RTSClient client = new RTSClient(accessKeyId, accessKeySecret, regionId); JoinSessionRequest request = new JoinSessionRequest(); request.setSessionId("your-session-id"); request.setSessionKey("your-session-key"); request.setRoleId("audio"); // 设置角色为音频 JoinSessionResult result = client.joinSession(request); if (result.isSuccess()) { String token = result.getToken(); System.out.println("加入通话成功,Token:" + token); } else { System.out.println("加入通话失败,错误信息:" + result.getErrorMessage()); } } }
In the above code, we also created an RTSClient instance and set the corresponding AccessKey and Region. Next, we create a JoinSessionRequest instance and set the session ID, session key, and role. Then, we call the joinSession method of RTSClient to join the call, and a JoinSessionResult object is returned. If joining the call is successful, we can get the Token from the JoinSessionResult object.
5. End the call
After the call ends, we need to call the interface to end the call and release the corresponding resources. The following is an example of ending a call:
import com.aliyun.rts.RTSClient; import com.aliyun.rts.model.LeaveSessionRequest; import com.aliyun.rts.model.LeaveSessionResult; public class LeaveSessionDemo { public static void main(String[] args) { String accessKeyId = "your-access-key-id"; String accessKeySecret = "your-access-key-secret"; String regionId = "cn-hangzhou"; RTSClient client = new RTSClient(accessKeyId, accessKeySecret, regionId); LeaveSessionRequest request = new LeaveSessionRequest(); request.setSessionId("your-session-id"); request.setSessionKey("your-session-key"); LeaveSessionResult result = client.leaveSession(request); if (result.isSuccess()) { System.out.println("结束通话成功"); } else { System.out.println("结束通话失败,错误信息:" + result.getErrorMessage()); } } }
Similarly, we create an RTSClient instance and set the corresponding AccessKey and Region. We then created a LeaveSessionRequest instance and set the session ID and session key. Finally, we call the RTSClient's leaveSession method to end the call, and return a LeaveSessionResult object.
Through the above steps, we successfully built a simple real-time audio and video communication system using Alibaba Cloud SDK and Java. Of course, in practical applications, more details such as audio and video encoding, decoding, and transmission need to be processed. However, through the introduction of this article, readers can have a preliminary understanding of how to use Alibaba Cloud SDK and Java to build a real-time audio and video communication system. I hope it will be helpful to readers.
The above is the detailed content of Build a real-time audio and video communication system using Alibaba Cloud SDK and Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
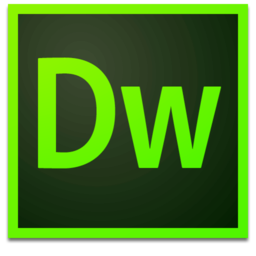
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
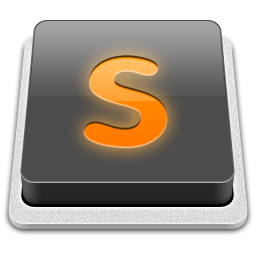
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.