Java connects to Tencent Cloud to implement face recognition system
Java connects to Tencent Cloud to implement face recognition system
Abstract:
The development of face recognition technology has penetrated into many fields, such as social entertainment, security protection, etc. This article will introduce how to use Java language and Tencent Cloud's face recognition API to build a simple face recognition system. We will start with creating a Tencent Cloud account and calling the face recognition API, and then write code through Java to implement the face recognition function.
- Creation of Tencent Cloud Account
Before starting, we need to create an account on Tencent Cloud. If you already have a Tencent Cloud account, you can use it directly. If not, you can visit Tencent Cloud official website to register and create an account. - Preparation for calling the face recognition API
In the Tencent Cloud console, we need to activate the face recognition API and obtain the API key and API key ID. This information will be used when calling the API. -
Maven dependency configuration
In Java projects, we can use Maven as a dependency management tool. In the project's pom.xml file, add the following dependencies:<dependency> <groupId>com.tencentcloudapi</groupId> <artifactId>tencentcloud-sdk-java</artifactId> <version>3.0.83</version> </dependency>
- Java code implementation
The following is a sample code for calling Tencent Cloud Face Recognition API using Java language:
import com.tencentcloudapi.common.Credential; import com.tencentcloudapi.common.exception.TencentCloudSDKException; import com.tencentcloudapi.common.profile.ClientProfile; import com.tencentcloudapi.common.profile.HttpProfile; import com.tencentcloudapi.common.profile.Language; import com.tencentcloudapi.faceid.v20180301.FaceidClient; import com.tencentcloudapi.faceid.v20180301.models.GetDetectInfoEnhancedRequest; import com.tencentcloudapi.faceid.v20180301.models.GetDetectInfoEnhancedResponse; public class FaceRecognitionSystem { public static void main(String[] args) { try { // 设置密钥和密钥ID Credential cred = new Credential("your-secret-id", "your-secret-key"); // 实例化一个HTTP选项,可选配置 HttpProfile httpProfile = new HttpProfile(); httpProfile.setReqMethod("POST"); // 默认为POST请求 httpProfile.setConnTimeout(60); // 连接超时时间,单位为秒 httpProfile.setEndpoint("faceid.tencentcloudapi.com"); // 设置接入的腾讯云服务域名 // 实例化一个客户端选项,可选配置 ClientProfile clientProfile = new ClientProfile(); clientProfile.setLanguage(Language.ZH_CN); // 设置SDK日志显示语言,默认为英文 clientProfile.setHttpProfile(httpProfile); // 实例化要请求的接口对应client对象,client对象需要传入clientProfile对象 FaceidClient client = new FaceidClient(cred, "", clientProfile); // 实例化一个请求对象 GetDetectInfoEnhancedRequest req = new GetDetectInfoEnhancedRequest(); // 设置请求参数,根据自己的需求进行设置 req.setBizToken("your-bizToken"); // 发起请求并且获取结果 GetDetectInfoEnhancedResponse res = client.GetDetectInfoEnhanced(req); System.out.println(GetDetectInfoEnhancedResponse.toJsonString(res)); } catch (TencentCloudSDKException e) { System.out.println(e.toString()); } } }
- Run the code
In the code, you need to replace "your-secret-id" and "your-secret-key" with your own Tencent Cloud key and key ID, and "your -bizToken" with the actual business token. You can then use the Java IDE to run the code and view the results.
Summary:
Through the above steps, we successfully used Java language and Tencent Cloud's face recognition API to build a simple face recognition system. In addition, system functions can be further expanded and optimized according to actual needs, such as adding face entry functions, face comparison functions, etc. I hope this article has provided some help for everyone to understand and use Java to connect to Tencent Cloud to implement the face recognition system.
The above is the detailed content of Java connects to Tencent Cloud to implement face recognition system. For more information, please follow other related articles on the PHP Chinese website!
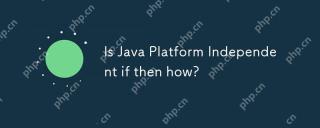
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
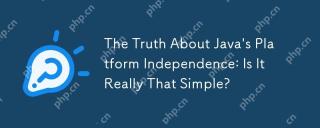
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
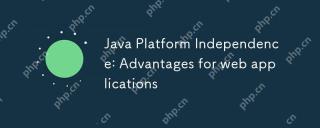
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
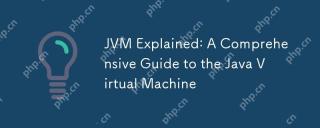
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
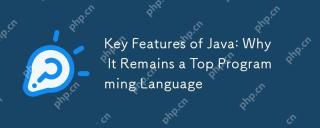
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
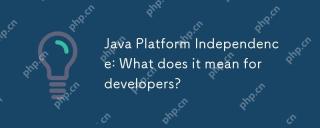
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
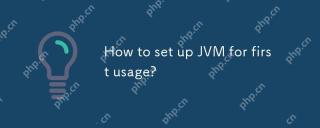
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
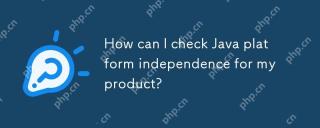
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
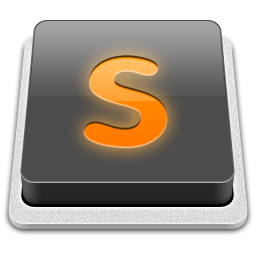
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
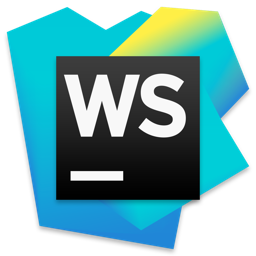
WebStorm Mac version
Useful JavaScript development tools
