


Method of using PHP and Qiniu cloud storage interface to implement image management function
Introduction:
With the rapid development of Internet technology, image management functions are becoming more and more popular in many websites and applications important. Using cloud storage services, such as Qiniu Cloud Storage, can help us manage and store large amounts of picture files efficiently. This article will introduce how to use PHP and Qiniu cloud storage interface to implement image management functions, and provide corresponding code examples.
1. Introduction to Qiniu Cloud Storage
Qiniu Cloud Storage is a flexible, scalable and secure cloud storage solution. It provides rich APIs and functions, such as uploading, downloading, deleting, managing resources, etc. We can use Qiniu Cloud Storage to build our own image management system and implement operations such as uploading, storing and displaying images.
2. Environment preparation
Before using Qiniu Cloud Storage, we need to make some preparations:
- Register a Qiniu Cloud Storage account and obtain AccessKey and SecretKey. These two keys are used for authentication and access control.
- Download and install PHP SDK. Qiniu Cloud Storage provides a PHP version of the SDK, which we can install through the composer tool.
3. Upload images
The following is a code example for using PHP to implement the image upload function:
<?php require 'vendor/autoload.php'; use QiniuAuth; use QiniuStorageUploadManager; // 需要填写你的 Access Key 和 Secret Key $accessKey = '<Your Access Key>'; $secretKey = '<Your Secret Key>'; // 构建鉴权对象 $auth = new Auth($accessKey, $secretKey); // 生成上传凭证 $bucket = '<Your Bucket>'; $token = $auth->uploadToken($bucket); // 上传文件的本地路径 $filePath = './path/to/image.jpg'; // 上传到七牛云存储 $uploadMgr = new UploadManager(); list($ret, $err) = $uploadMgr->putFile($token, null, $filePath); if ($err !== null) { echo '上传失败:' . var_export($err, true); } else { echo '上传成功'; }
The above code first references the SDK file and uses Access Key and Secret Key The authentication object is initialized. Then the upload credentials are generated through the authentication object, and the upload target space (bucket) is specified. Finally, call the putFile method of uploadManager to upload the image file, and output the corresponding information based on the upload result.
4. Image Management
In addition to uploading images, we also need to implement image management functions, such as obtaining and deleting images, etc. The following is a code example that uses PHP to implement image acquisition and deletion functions:
<?php require 'vendor/autoload.php'; use QiniuAuth; use QiniuStorageBucketManager; // 需要填写你的 Access Key 和 Secret Key $accessKey = '<Your Access Key>'; $secretKey = '<Your Secret Key>'; // 构建鉴权对象 $auth = new Auth($accessKey, $secretKey); // 空间名 $bucket = '<Your Bucket>'; // 生成资源管理对象 $bucketManager = new BucketManager($auth); // 获取空间中的所有文件列表 $filesList = $bucketManager->listFiles($bucket); // 输出文件列表 foreach ($filesList[0] as $file) { echo $file['key'] . " "; } // 删除文件 $fileKey = '<Your File Key>'; $bucketManager->delete($bucket, $fileKey); echo '文件删除成功';
The above code first references the SDK file and initializes the authentication object using Access Key and Secret Key. Then the resource management object is generated based on the authentication object, and the target space to be operated is specified. You can get a list of all files in the space by calling the listFiles method through the resource management object; you can delete the specified file by calling the delete method.
Conclusion:
Through the above sample code, we can implement basic image upload and management functions. Of course, Qiniu Cloud Storage also provides more functions and interfaces, such as image processing, thumbnail generation, persistence processing, etc. For specific usage, please refer to the official documentation and SDK documentation of Qiniu Cloud Storage. I hope this article can help you quickly get started using PHP and Qiniu Cloud Storage to implement image management functions.
The above is the detailed content of Method to implement picture management function using PHP and Qiniu cloud storage interface. For more information, please follow other related articles on the PHP Chinese website!
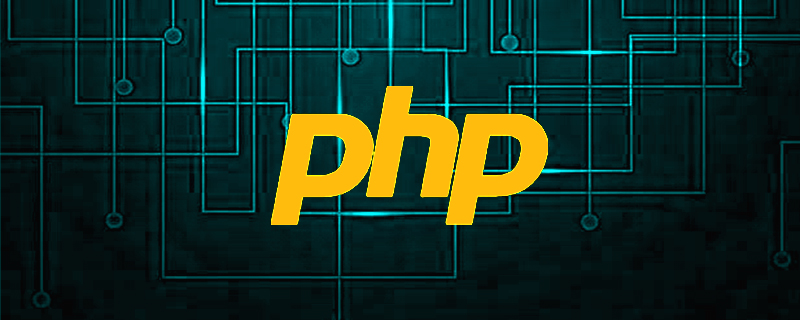
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
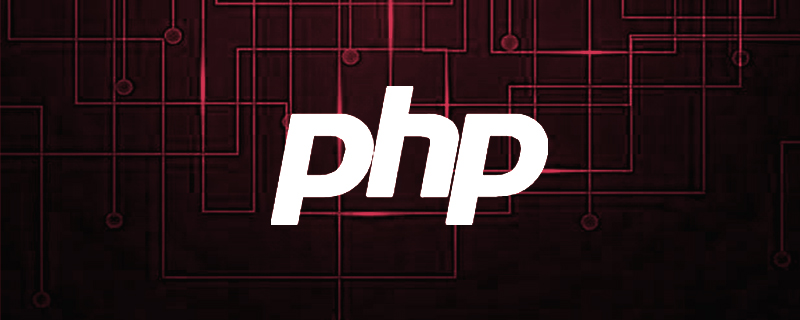
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
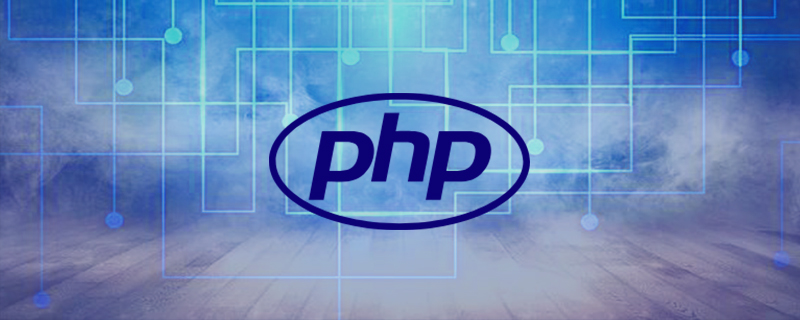
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
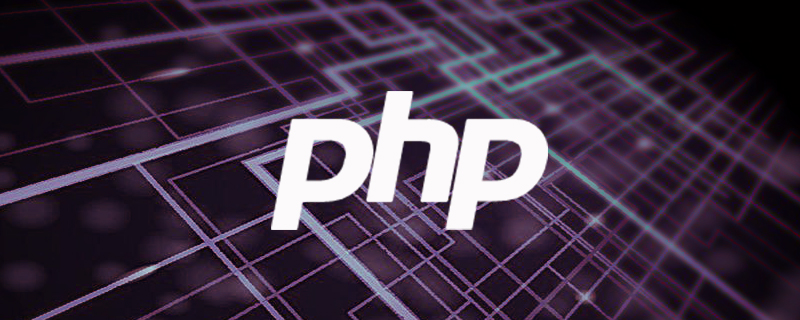
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
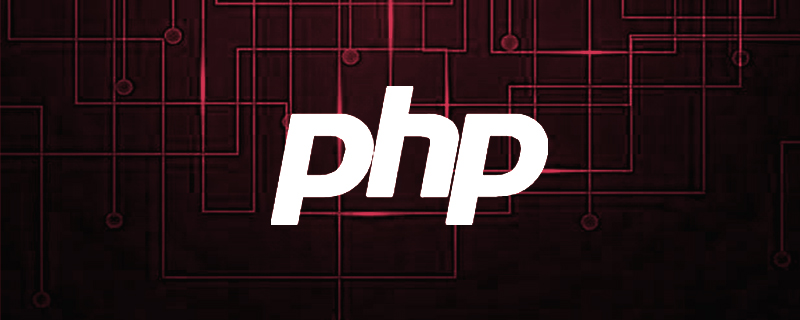
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
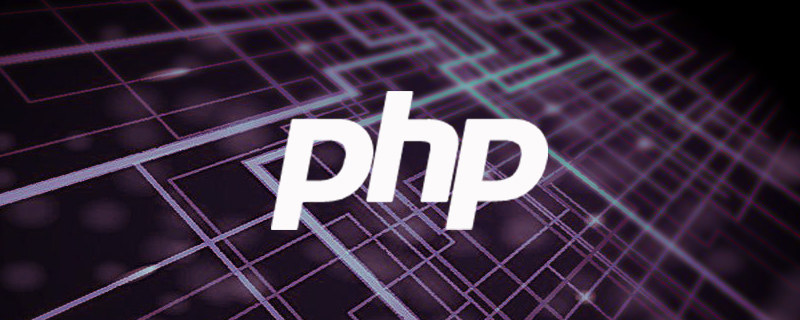
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
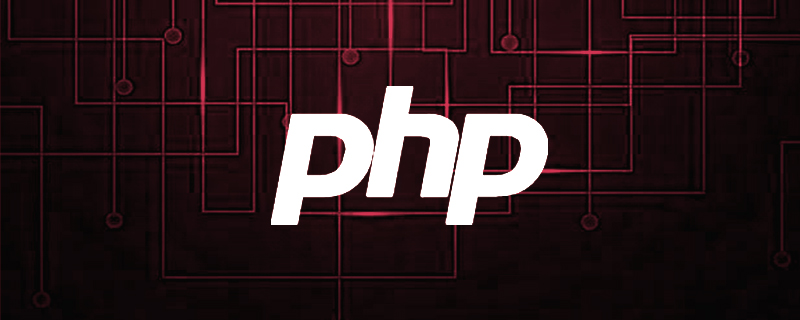
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
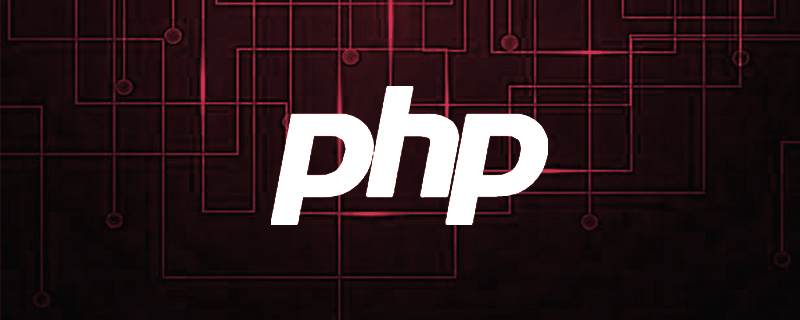
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
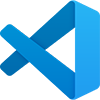
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
