


Analysis of the interface docking steps between PHP and Youpaiyun
Overview:
Youpaiyun is an excellent cloud storage service provider, providing developers with rich interfaces and functions to facilitate File storage, management and access. This article will introduce how to use PHP to connect with Youpaiyun's interface, and provide relevant code examples.
Step 1: Register a Youpaiyun account
First, you need to register an account on the Youpaiyun official website and obtain the access key and secret key. These two parameters are important credentials for interface operations and will be used in subsequent steps.
Step 2: Install Youpaiyun SDK
In the PHP project, we can install Youpaiyun SDK through composer. The specific installation command is as follows:
composer require upyun/sdk
Step 3: Initialize Youpaiyun SDK Cloud object
Before the interface is called, the cloud object needs to be initialized and photographed. You can initialize according to the following sample code:
require_once 'vendor/autoload.php'; // 初始化又拍云对象 use UpyunUpyun; use UpyunConfig; $config = new Config('your_operator', 'your_operator_password'); $upyun = new Upyun($config);
Step 4: Upload files
Next, we can use the interface provided by Youpaiyun to upload files. The following is a sample code for uploading files:
$file = fopen('/path/to/local/file', 'r'); $remotePath = '/path/to/remote/file'; $result = $upyun->write($remotePath, $file, true); if ($result) { echo '上传成功!'; } else { echo '上传失败!'; }
Among them, $file
is the file handle of the local file, and $remotePath
is the file path uploaded to Youpaiyun. $upyun->write()
The method is used to perform the upload operation. The third parameter indicates whether to perform server-side file verification.
Step 5: Download the file
After the file has been uploaded to Youpaiyun, we can use the interface provided by Youpaiyun to download the file. The following is a sample code for downloading a file:
$remotePath = '/path/to/remote/file'; $localPath = '/path/to/local/file'; $result = $upyun->read($remotePath, $localPath); if ($result) { echo '下载成功!'; } else { echo '下载失败!'; }
Among them, $remotePath
is the file path of Youpaiyun, and $localPath
is the file path downloaded to the local. $upyun->read()
The method is used to perform download operations.
Step 6: Delete files
If a file is no longer needed, we can use the interface provided by Youpaiyun to delete the file. The following is a sample code to delete a file:
$remotePath = '/path/to/remote/file'; $result = $upyun->delete($remotePath); if ($result) { echo '删除成功!'; } else { echo '删除失败!'; }
Among them, $remotePath
is the file path of Youpaiyun. $upyun->delete()
The method is used to perform delete operations.
Summary:
Through the above steps, we can use the interface between PHP and Youpaiyun to upload, download and delete files. Youpaiyun provides rich interface documents to facilitate developers to perform more file management and operations. I hope this article can help you successfully connect the interface between PHP and Youpai Cloud to meet your business needs.
The above is the detailed content of Analysis of the interface docking steps between PHP and Youpaiyun. For more information, please follow other related articles on the PHP Chinese website!
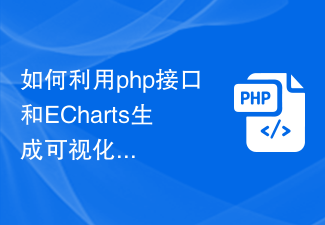
在今天数据可视化变得越来越重要的背景下,许多开发者都希望能够利用各种工具,快速生成各种图表与报表,以便能够更好的展示数据,帮助决策者快速做出判断。而在此背景下,利用Php接口和ECharts库可以帮助许多开发者快速生成可视化的统计图表。本文将详细介绍如何利用Php接口和ECharts库生成可视化的统计图表。在具体实现时,我们将使用MySQL
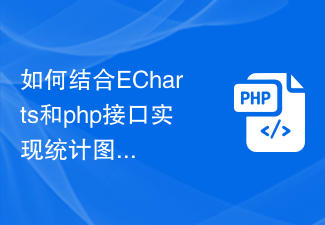
如何结合ECharts和PHP接口实现统计图的动态更新引言:数据可视化在现代应用程序中起着至关重要的作用。ECharts是一个优秀的JavaScript图表库,可以帮助我们轻松创建各种类型的统计图表。而PHP则是一种广泛应用于服务器端开发的脚本语言。通过结合ECharts和PHP接口,我们可以实现统计图的动态更新,使图表能够根据实时数据的变化进行自动更新。本
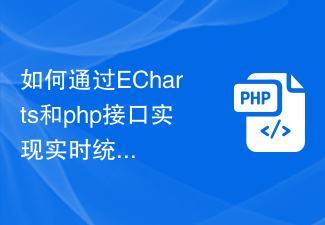
如何通过ECharts和PHP接口实现实时统计图的展示随着互联网和大数据技术的快速发展,数据可视化成为了重要的一环。而ECharts作为一款优秀的开源JavaScript数据可视化库,能够帮助我们简单、高效地实现各种统计图的展示。本文将介绍如何通过ECharts和PHP接口实现实时统计图的展示,并提供相关代码示例。一、前期准备在开始之前,我们需要做一些准备工
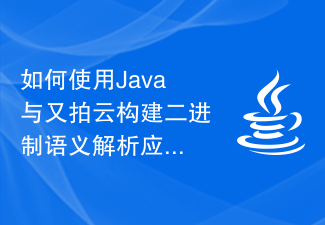
如何使用Java与又拍云构建二进制语义解析应用又拍云是一家提供云存储和云处理解决方案的服务商,开发者可以通过它提供的API来实现各种功能。在本文中,我们将介绍如何使用Java语言与又拍云的API来构建一个二进制语义解析应用。该应用可以读取上传到又拍云存储的二进制文件,并使用自然语言处理技术来解析其中的语义信息。创建又拍云账号和存储空间首先,我们需要在又拍云官
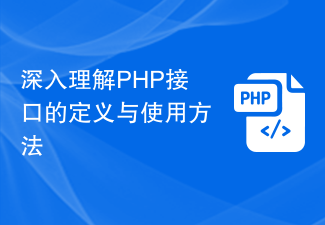
深入理解PHP接口的定义与使用方法PHP是一种强大的服务器端脚本语言,广泛应用于Web开发领域。在PHP中,接口(interface)是一种重要的概念,它可以用来定义一组方法的规范,而不关心方法的具体实现。本文将深入探讨PHP接口的定义和使用方法,并提供具体的代码示例。1.什么是接口?在面向对象编程中,接口是一种抽象的概念,它定义了一组方法的规范,但没有具
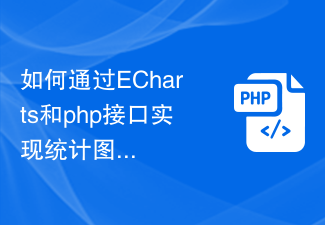
如何通过ECharts和PHP接口实现统计图的数据验证和校验随着数据可视化的需求增加,ECharts成为了一个非常流行的数据可视化工具。而PHP作为一种常见的后端脚本语言,也广泛应用于Web开发中。本文将介绍如何通过ECharts和PHP接口实现统计图的数据验证和校验,并提供具体的代码示例。首先,我们需要了解ECharts。ECharts是一个由百度开发的开
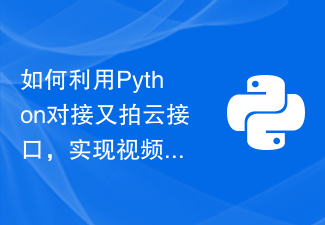
如何利用Python对接又拍云接口,实现视频剪切功能又拍云是一家国内知名的云存储和内容分发网络服务提供商,提供了丰富的云存储服务。本文将介绍如何利用Python编写代码对接又拍云接口,实现视频剪切功能。首先,我们需要在又拍云的官方网站上注册账户并创建项目。创建项目后,可以获得项目的服务名、操作员账号和操作员密码,这些信息后续会在代码中用到。接下来,我们需要在
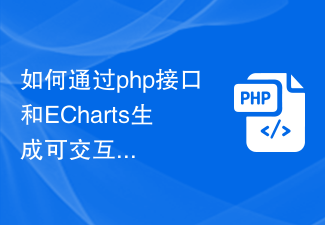
在现代化的应用程序中,数据的可视化变得越来越流行。统计图表是一种很好的数据可视化方式,可以轻松地帮助用户了解数据的趋势。ECharts是一个强大的前端图表框架,它提供了丰富的图表类型和交互式功能。Php是一种非常流行的后端语言,可以轻松地生成动态内容和接口。在本文中,我们将介绍如何使用php接口和ECharts生成可交互的统计图表,并提供具体的代码示例。一、


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
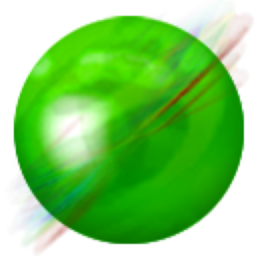
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
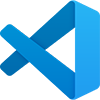
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),