


Method of using PHP and Qiniu Cloud Storage interface to implement image compression processing
In web development, it is often necessary to compress images to improve web page loading speed and save bandwidth, and Qiniu Cloud Storage It provides a wealth of interfaces and functions to easily compress images. This article will introduce how to use PHP and Qiniu cloud storage interface to implement image compression processing, and give corresponding code examples.
First, we need to create a storage space on the Qiniu cloud storage platform and obtain the corresponding AccessKey and SecretKey, which will be used to authenticate the interface.
Next, we need to use the CURL library provided by PHP to send HTTP requests and process the returned results. We can introduce the CURL library through the following code:
<?php $ch = curl_init();//初始化 curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);//将结果返回到变量而不是直接输出 curl_setopt($ch, CURLOPT_HEADER, false);//header信息不输出
Then, we use the following code to implement the function of compressing images:
<?php $accessKey = 'your_access_key'; $secretKey = 'your_secret_key'; $bucket = 'your_bucket'; $key = 'your_image_key'; $imageURL = "http://" . $bucket . ".qiniudn.com/" . $key;//获取图片的URL $options = "imageView2/2/w/500/h/500/q/90";//压缩图片的参数 $url = "http://pfop.qiniu.com/pfop/"; $data = array("bucket" => $bucket, "key" => $key, "fops" => $options);//构建请求参数 $encodedData = json_encode($data); $sign = hash_hmac('sha1', $encodedData, $secretKey, true);//对参数进行签名 $encodedSign = str_replace(array('+', '/'), array('-', '_'), base64_encode($sign));//对签名进行URL安全的base64编码 $uploadURL = $url . $encodedSign; curl_setopt($ch, CURLOPT_URL, $uploadURL); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS, $encodedData); $result = curl_exec($ch);//发送请求并获取返回结果 if ($result === false) { echo "Error: " . curl_error($ch);//发送请求失败,输出错误信息 } else { echo "Success: " . $result;//发送请求成功,输出返回结果 } curl_close($ch);//关闭请求 ?>
In the above code, we first define AccessKey, SecretKey, storage space name (bucket) and key of the image. Then, we constructed a suitable image URL and defined the parameters (options) for compressing the image. Here we specified the width and height of the image to be 500 pixels, and set the compression quality to 90. Next, we build these parameters into an array and encode them via json_encode(). Then, we sign this encoded array to get a signed value. Finally, we build the signature value and parameters together into a URL and send the HTTP request using the CURL library. If the request is successful, we will get a return result and can print the result on success.
It should be noted that the above code is just a simple example and needs to be modified appropriately according to your own needs when used in practice.
To sum up, we have successfully achieved image compression by using PHP and Qiniu Cloud Storage interface. Through this method, we can easily reduce the size of the image and improve the loading speed of the web page to provide users with a better experience. I hope this article can be helpful to everyone who encounters image processing problems in development.
The above is the detailed content of Method to realize image compression processing using PHP and Qiniu cloud storage interface. For more information, please follow other related articles on the PHP Chinese website!
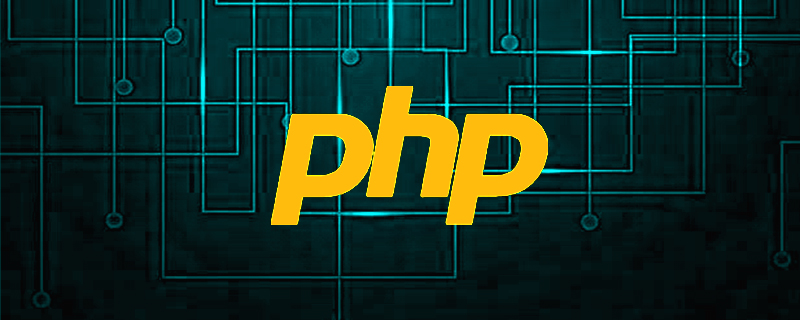
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
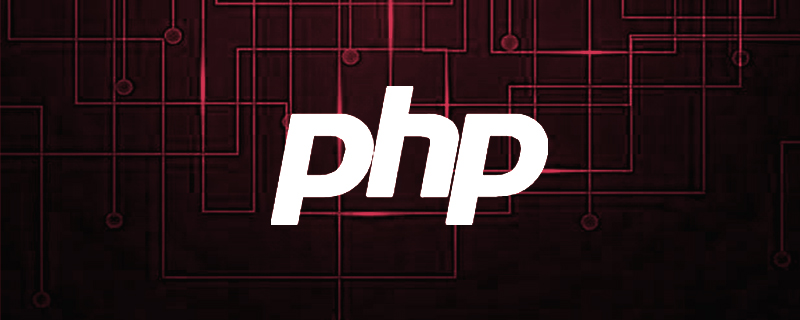
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
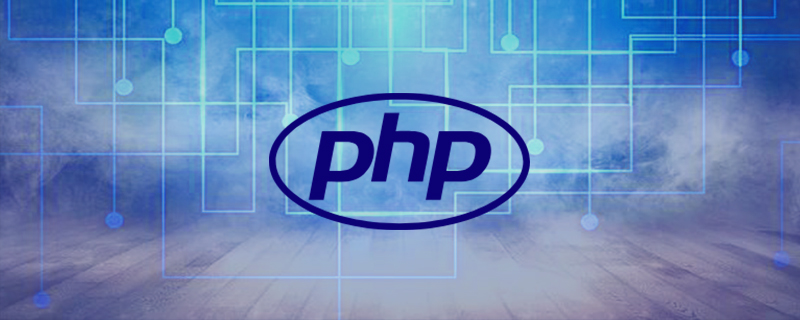
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
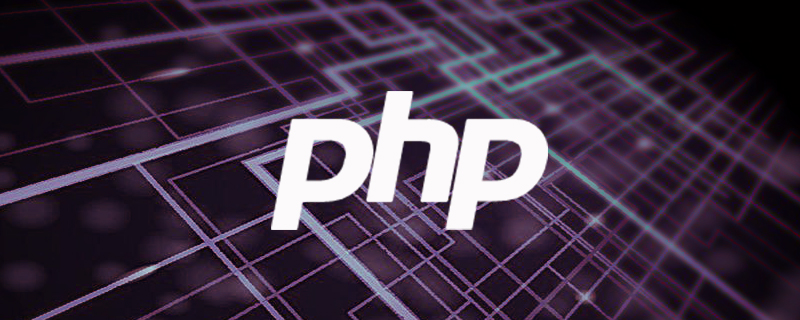
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
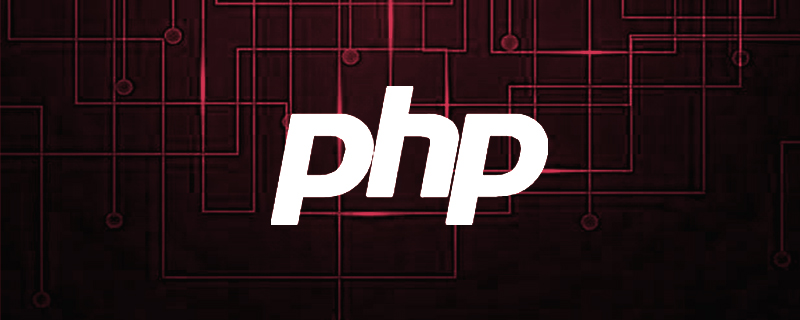
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
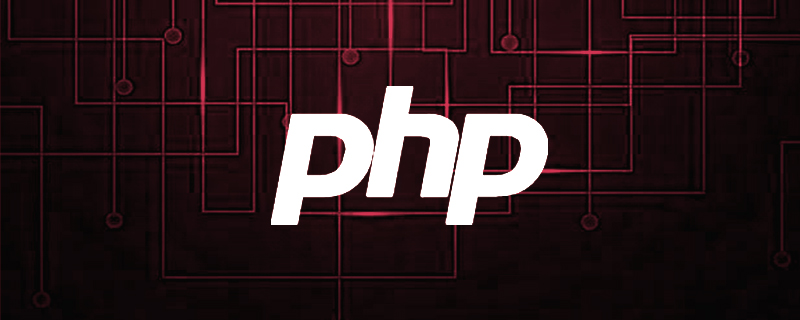
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
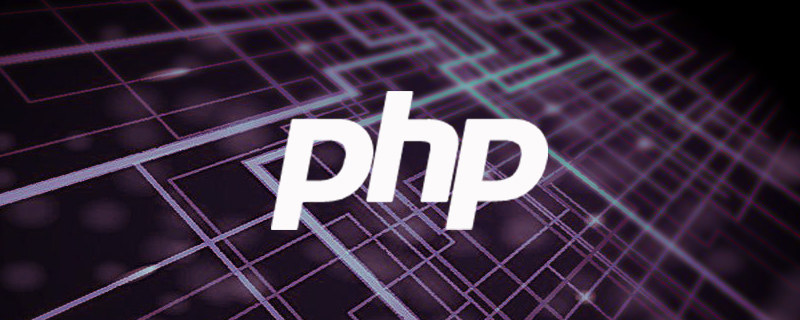
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
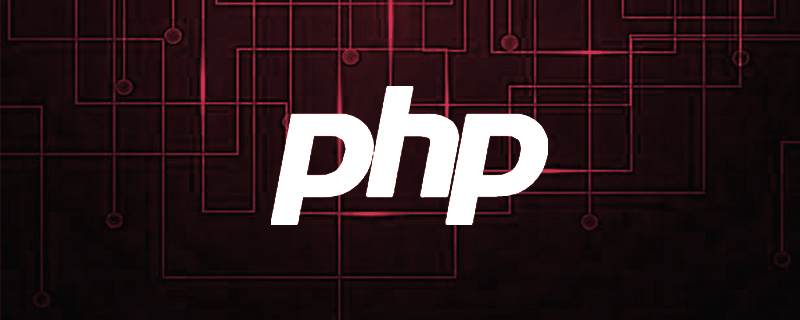
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
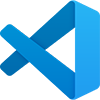
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
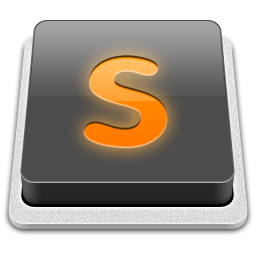
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
