


Practical Guide to Connecting PHP and Qiniu Cloud Storage Interface
Practical Guide to Connecting PHP and Qiniu Cloud Storage Interface
Introduction:
Cloud storage has become one of the commonly used solutions in most website development. Qiniu Cloud Storage, as the leading domestic cloud storage service provider, is loved by many developers. This article will introduce how to use PHP to interface with Qiniu Cloud Storage, and provide relevant code examples.
1. Register a Qiniu cloud storage account and create a storage space
Before starting, we need to register a Qiniu cloud storage account and create a storage space. The registration URL is https://www.qiniu.com. After successful registration, log in to the Qiniu Cloud Storage Console. In the console, find the "Storage Space" option and click the "Create Storage Space" button to create a new storage space.
2. Install Qiniu Cloud Storage SDK
Qiniu Cloud provides PHP SDK, which we can install through Composer. Open the terminal, enter the root directory of the project, and execute the following command to install:
composer require qiniu/php-sdk
3. Obtain Qiniu Cloud Storage Access Key and Secret Key
In Before connecting the interface, we need to obtain the Access Key and Secret Key of Qiniu Cloud Storage to facilitate subsequent permission verification. In the Qiniu Cloud Storage console, find the "Personal Panel" in the left navigation bar, click to enter, and then you can see the Access Key and Secret Key under the API Keys column.
4. Upload files to Qiniu Cloud Storage
Next, we will learn how to use PHP code to upload files to Qiniu Cloud Storage. First, we need to introduce the Qiniu Cloud Storage SDK and initialize the configuration.
require_once 'vendor/autoload.php'; use QiniuAuth; use QiniuStorageUploadManager; $accessKey = 'your_access_key'; $secretKey = 'your_secret_key'; $auth = new Auth($accessKey, $secretKey);
In the above code, we introduced the Auth and UploadManager classes of Qiniu Cloud Storage, initialized an Auth object, and used Access Key and Secret Key for authentication.
Next, we need to define an upload function to implement the logic of file upload. The code is as follows:
function uploadFile($bucket, $key, $filePath) { global $auth; $token = $auth->uploadToken($bucket); $uploadMgr = new UploadManager(); list($ret, $err) = $uploadMgr->putFile($token, $key, $filePath); if ($err !== null) { return false; } else { return true; } }
In the above code, we first obtain the upload credentials based on the name of the storage space, and use the upload credentials, the file's saving path, and the file's local path to call the putFile method of UploadManager to upload the file. If the upload is successful, the function returns true; otherwise, it returns false.
Finally, we can call the uploadFile function to upload files to Qiniu Cloud Storage. The sample code is as follows:
$bucket = 'your_bucket_name'; $key = 'your_file_key'; $filePath = '/path/to/your/file'; if (uploadFile($bucket, $key, $filePath)) { echo '文件上传成功'; } else { echo '文件上传失败'; }
In the above code, we need to replace the $bucket variable with the name of your storage space, the $key variable with the file name you want to save (including the file path), and the $filePath variable with The absolute path to your local file. Then call the uploadFile function to upload the file, and determine whether the upload is successful based on the returned result.
5. Delete files on Qiniu Cloud Storage
In addition to uploading files, we can also use PHP code to delete files on Qiniu Cloud Storage. To delete a file, you need to provide the storage space name and file name of the file.
function deleteFile($bucket, $key) { global $auth; $bucketMgr = new BucketManager($auth); $err = $bucketMgr->delete($bucket, $key); if ($err !== null) { return false; } else { return true; } }
In the above code, we first initialize a BucketManager object and call the delete method to delete the specified file. If the deletion is successful, the function returns true; otherwise, it returns false.
The sample code for using the deleteFile function to delete a file is as follows:
$bucket = 'your_bucket_name'; $key = 'your_file_key'; if (deleteFile($bucket, $key)) { echo '文件删除成功'; } else { echo '文件删除失败'; }
In the above code, we need to replace the $bucket variable with the name of the storage space and the $key variable with the name of the file to be deleted. Then call the deleteFile function to delete the file, and judge whether the deletion is successful based on the returned result.
Conclusion:
Through the study of this article, you have learned how to use PHP to interface with Qiniu Cloud Storage, and realize the file upload and file deletion functions. I hope this article is helpful to your learning and use!
The above is the detailed content of Practical Guide to Connecting PHP and Qiniu Cloud Storage Interface. For more information, please follow other related articles on the PHP Chinese website!
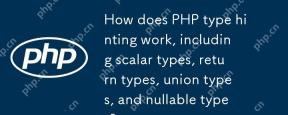
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
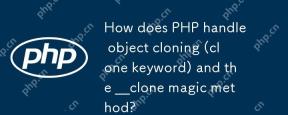
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
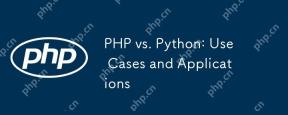
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
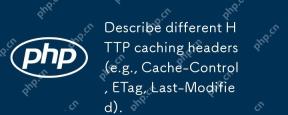
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
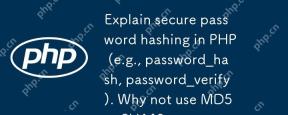
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
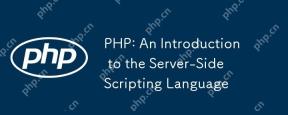
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
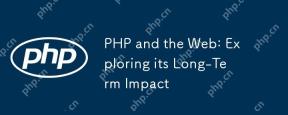
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
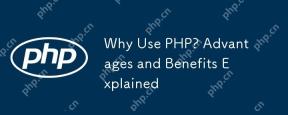
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
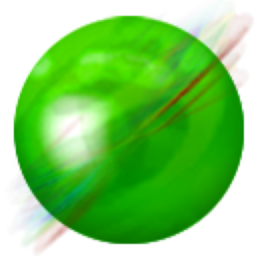
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
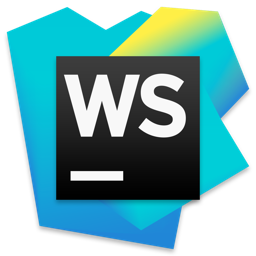
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version