


Method to realize intelligent recognition and label classification of pictures using PHP and Qiniu cloud storage interface
Method of using PHP and Qiniu cloud storage interface to realize intelligent recognition and label classification of images
In recent years, with the rapid development of artificial intelligence technology, the field of image recognition has also made significant progress. Using machine learning and deep learning algorithms, we can allow computers to intelligently understand and analyze image content, thereby achieving intelligent recognition and label classification of images. This article will introduce how to use PHP and Qiniu cloud storage interface to implement this function, and give corresponding code examples.
First, we need to create an account on Qiniu Cloud Storage (https://www.qiniu.com/) and obtain the AccessKey and SecretKey of the account to access the API of Qiniu Cloud Storage .
Next, we need to use the SDK provided by Qiniu Cloud Storage to operate images. In PHP, you can use the officially provided Qiniu Cloud Storage SDK (https://developer.qiniu.com/sdk/php) to interact with Qiniu Cloud Storage.
The following is a simple code example that demonstrates how to use PHP and Qiniu Cloud Storage interface to upload an image, and call Qiniu Cloud Storage's image recognition API to obtain the tag classification of the image:
<?php require 'autoload.php'; // 导入七牛云存储的PHP SDK use QiniuStorageUploadManager; use QiniuAuth; // 需要填写七牛云存储的AccessKey和SecretKey $accessKey = 'your-access-key'; $secretKey = 'your-secret-key'; // 初始化Auth对象 $auth = new Auth($accessKey, $secretKey); // 需要上传的图片文件路径 $filePath = './path/to/your/image.jpg'; // 生成上传Token $token = $auth->uploadToken('your-bucket-name'); // 初始化UploadManager对象 $uploadMgr = new UploadManager(); // 上传图片到七牛云存储 list($ret, $err) = $uploadMgr->putFile($token, null, $filePath); if ($err !== null) { // 上传图片失败 echo 'Upload failed:', $err->message(); } else { // 上传图片成功 $imageKey = $ret['key']; // 调用七牛云存储的图像识别API,获取图片的标签分类 $url = 'http://ai.qiniuapi.com/v1/ai/predict'; $data = ['url' => 'your-image-url', 'model' => 'img_tag']; $headers = [ 'Content-Type:application/json', 'Authorization:Qiniu ' . $auth->signRequest($url, null, 'POST', $headers, json_encode($data)) ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data)); $response = curl_exec($ch); curl_close($ch); // 解析返回的结果 $result = json_decode($response, true); // 获取图片的标签分类 $labels = isset($result['result']) ? $result['result'] : []; // 输出图片的标签分类 echo 'Image labels:', implode(', ', $labels); }
In the above code, we first initialize an Auth object using AccessKey and SecretKey, and then generate an upload Token. Then, call the putFile method of the UploadManager object to upload the image file to Qiniu Cloud Storage, and get the upload result.
Next, we construct an HTTP request and call the image recognition API of Qiniu Cloud Storage. The API accepts an image URL and a model name as parameters and returns the label classification results of the image. We use the curl library to send HTTP requests. What needs to be noted is that the request header needs to be signed based on AccessKey and SecretKey.
Finally, we parse the return results of the API, obtain the label classification of the image, and output the results.
Through the above code examples, we can see that it is not complicated to use PHP and Qiniu cloud storage interface to realize intelligent recognition and label classification of images. By uploading images to Qiniu Cloud Storage and then calling Qiniu Cloud Storage's image recognition API, we can easily obtain the label classification information of the image, providing more possibilities for subsequent image processing and applications.
The above is the detailed content of Method to realize intelligent recognition and label classification of pictures using PHP and Qiniu cloud storage interface. For more information, please follow other related articles on the PHP Chinese website!
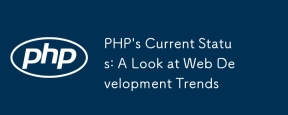
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
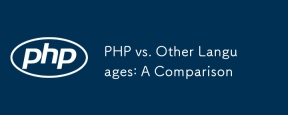
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
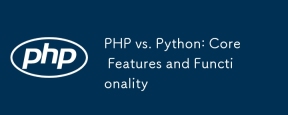
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
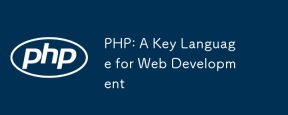
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
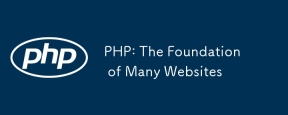
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
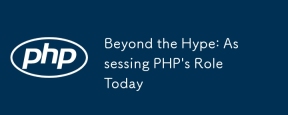
PHP remains a powerful and widely used tool in modern programming, especially in the field of web development. 1) PHP is easy to use and seamlessly integrated with databases, and is the first choice for many developers. 2) It supports dynamic content generation and object-oriented programming, suitable for quickly creating and maintaining websites. 3) PHP's performance can be improved by caching and optimizing database queries, and its extensive community and rich ecosystem make it still important in today's technology stack.
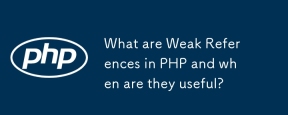
In PHP, weak references are implemented through the WeakReference class and will not prevent the garbage collector from reclaiming objects. Weak references are suitable for scenarios such as caching systems and event listeners. It should be noted that it cannot guarantee the survival of objects and that garbage collection may be delayed.
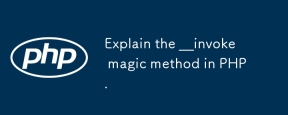
The \_\_invoke method allows objects to be called like functions. 1. Define the \_\_invoke method so that the object can be called. 2. When using the $obj(...) syntax, PHP will execute the \_\_invoke method. 3. Suitable for scenarios such as logging and calculator, improving code flexibility and readability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
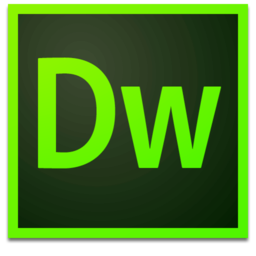
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.