


How to use Python to connect to Youpaiyun interface to realize video clipping and merging functions
Abstract: This article introduces how to use Python language to connect to Youpaiyun interface to realize video clipping and merging functions. First, we need to understand the basic concepts and API interfaces of Youpaiyun. Secondly, we will use Python to write code to implement video cropping and merging functions, and upload and download video files through the API interface of Youpai Cloud. Finally, we will demonstrate how to trim and merge multiple video files into a complete video file.
Keywords: Python, Youpaiyun, API interface, video clipping, video merging
1. Youpaiyun introduction
Youpaiyun is a leading domestic cloud computing service provider It has powerful cloud storage, cloud acceleration and cloud processing capabilities. By connecting to Youpai Cloud's API interface, we can easily implement various cloud storage and cloud processing functions in our applications.
2. Python docking and cloud interface
Python is a programming language that is simple, easy to learn, and highly efficient in development. It is very suitable for docking and interacting with cloud platforms. We can use Python's requests library to communicate with Youpaiyun's API interface and complete various operations.
The following is a basic code example of Python docking with Youpaiyun interface:
import requests # API请求地址 api_url = "https://api.upyun.com" # 又拍云操作员用户名和密码 operator = "your_operator" password = "your_password" # 授权字符串 auth = requests.auth.HTTPBasicAuth(operator, password) # 上传文件 def upload_file(filepath, filename): api = "/bucketname/" + filename with open(filepath, 'rb') as file: response = requests.put(api_url + api, data=file, auth=auth) return response # 下载文件 def download_file(filename): api = "/bucketname/" + filename response = requests.get(api_url + api, auth=auth) return response.content # 删除文件 def delete_file(filename): api = "/bucketname/" + filename response = requests.delete(api_url + api, auth=auth) return response # 其他操作...
3. Implementation of video clipping function
By connecting with Youpaiyun API interface, we can implement the video clipping function . First, we need to obtain the URL address of the original video file, and then use FFmpeg, an open source tool, to achieve video cropping.
The following is a code example for Python to implement the video trimming function:
import subprocess # 剪裁视频 def crop_video(original_url, start_time, end_time): # 从又拍云下载原始视频文件 original_data = download_file(original_url) with open("original.mp4", "wb") as file: file.write(original_data) # 使用FFmpeg进行视频剪裁 subprocess.call(["ffmpeg", "-i", "original.mp4", "-ss", start_time, "-to", end_time, "cropped.mp4"]) # 上传剪裁后的视频文件到又拍云 response = upload_file("cropped.mp4", "cropped.mp4") # 返回剪裁后的视频文件URL cropped_url = response.url return cropped_url
4. Implementation of the video merging function
By connecting to the API interface of Youpaiyun, we can implement the video merging function. First, we need to obtain the URL addresses of multiple video files, and then use FFmpeg, an open source tool, to merge the videos.
The following is a code example for Python to implement the video merging function:
# 合并视频 def merge_videos(video_urls, output_file): # 下载多个视频文件 for url in video_urls: video_data = download_file(url) filename = url.split("/")[-1] with open(filename, "wb") as file: file.write(video_data) # 使用FFmpeg进行视频合并 subprocess.call(["ffmpeg", "-i", "concat:{}".format("|".join([f for f in os.listdir() if f.endswith(".mp4")])), "-c", "copy", output_file]) # 上传合并后的视频文件到又拍云 response = upload_file(output_file, output_file) # 返回合并后的视频文件URL merged_url = response.url return merged_url
5. Video cropping and merging practice
Finally, we will demonstrate how to crop and merge multiple video files into one Complete video file.
The following is a code example for practicing video clipping and merging functions in Python:
# 测试视频剪裁与合并 if __name__ == "__main__": original_url = "https://your_bucket.b0.upaiyun.com/original.mp4" cropped_url = crop_video(original_url, "00:00:10", "00:00:20") new_video_urls = [original_url, cropped_url] merged_url = merge_videos(new_video_urls, "output.mp4") print("剪裁后的视频URL:", cropped_url) print("合并后的视频URL:", merged_url)
6. Summary
This article introduces how to use Python language to connect to the Youpai Cloud interface to realize video clipping and merging. function. By connecting to Youpaiyun's API interface, we can easily upload, download and delete video files. At the same time, we also demonstrated how to use the FFmpeg tool for video cropping and merging operations. I hope this article will be helpful for readers to understand how to use Python to connect to the cloud interface to implement video cropping and merging functions.
The above is the detailed content of How to use Python to connect to the cloud interface to implement video cropping and merging functions. For more information, please follow other related articles on the PHP Chinese website!
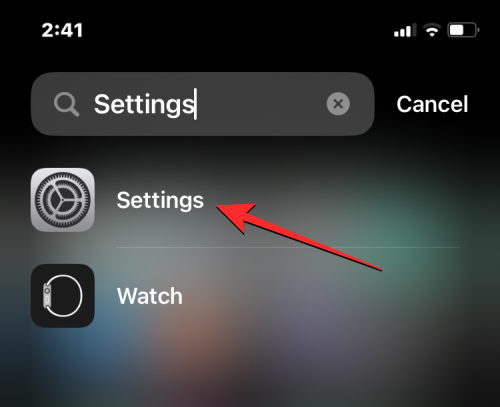
白平衡是一项相机功能,可根据照明条件调整演色性。此iPhone设置可确保白色物体在照片或视频中显示为白色,从而补偿由于典型照明而导致的任何颜色变化。如果您想在整个视频拍摄过程中保持白平衡一致,可以将其锁定。在这里,我们将指导您如何为iPhone视频保持固定的白平衡。如何在iPhone上锁定白平衡必需:iOS17更新。(检查“常规>软件更新”下的“设置”>)。在iPhone上打开“设置”应用。在“设置”中,向下滚动并选择“相机”。在“相机”屏幕上,点击“录制视频”。在这
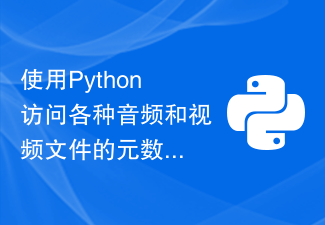
我们可以使用Mutagen和Python中的eyeD3模块访问音频文件的元数据。对于视频元数据,我们可以使用电影和Python中的OpenCV库。元数据是提供有关其他数据(例如音频和视频数据)的信息的数据。音频和视频文件的元数据包括文件格式、文件分辨率、文件大小、持续时间、比特率等。通过访问这些元数据,我们可以更有效地管理媒体并分析元数据以获得一些有用的信息。在本文中,我们将了解Python提供的一些用于访问音频和视频文件元数据的库或模块。访问音频元数据一些用于访问音频文件元数据的库是-使用诱变
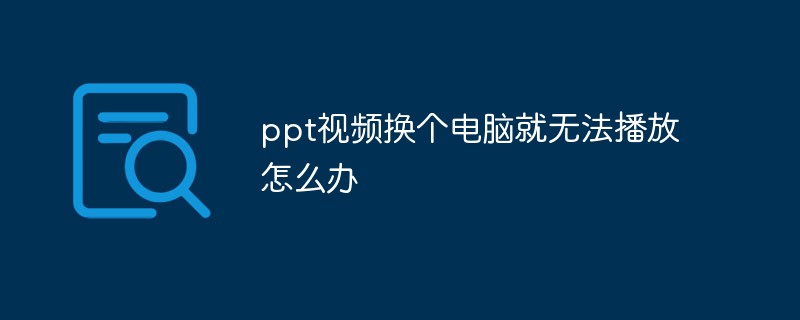
ppt视频换个电脑就无法播放是因为路径不对,其解决办法:1、将PPT和视频放入U盘的同一个文件夹内;2、双击打开该PPT,找到想要插入视频的页数,点击“插入”按钮;3、在弹出的对话框内选择想要插入的视频即可。
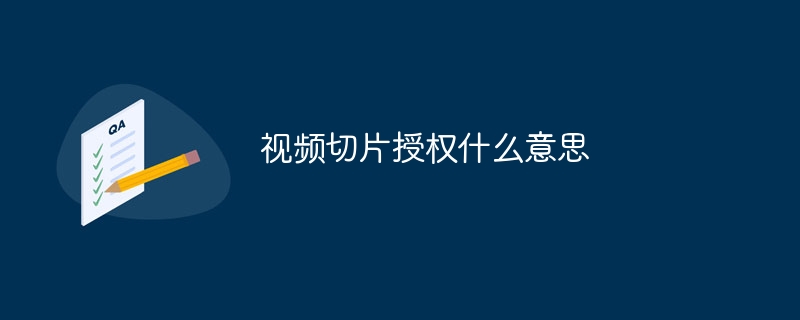
视频切片授权是指在视频服务中,将视频文件分割成多个小片段并进行授权的过程。这种授权方式能提供更好的视频流畅性、适应不同网络条件和设备,并保护视频内容的安全性。通过视频切片授权,用户可以更快地开始播放视频,减少等待和缓冲时间,视频切片授权可以根据网络条件和设备类型动态调整视频参数,提供最佳的播放效果,视频切片授权还有助于保护视频内容的安全性,防止未经授权的用户进行盗播和侵权行为。
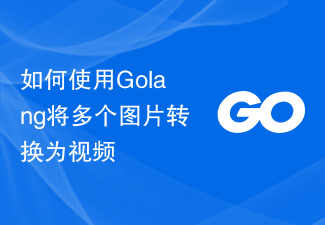
如何使用Golang将多个图片转换为视频随着互联网的发展和智能设备的普及,视频成为了一种重要的媒体形式。有时候我们可能需要将多个图片转换为视频,以便展示图片的连续变化或者制作幻灯片。本文将介绍如何使用Golang编程语言将多个图片转换为视频。在开始之前,请确保你已经安装了Golang以及相关的开发环境。步骤1:导入相关的包首先,我们需要导入一些相关的Gola
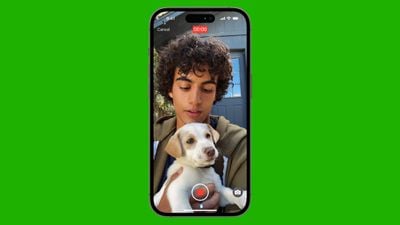
在iOS17中,当您FaceTime通话某人无法接听时,您可以留下视频或音频信息,具体取决于您使用的通话方式。如果您使用的是FaceTime视频,则可以留下视频信息,如果您使用的是FaceTime音频,则可以留下音频信息。您所要做的就是以通常的方式与某人进行FaceTime。未接来电后,您将看到“录制视频”选项,该选项可让您创建消息。录制完视频后,您会看到视频的预览,如果效果不佳,还可以选择重新录制。以下是在运行iOS17的设备上留下FaceTime消息的工作原理,以未接视频通话为例,在Face
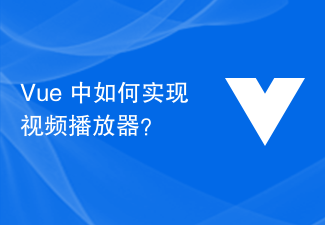
随着互联网的不断发展,视频已经成为了人们日常生活中必不可少的娱乐方式之一。为了给用户提供更好的视频观看体验,许多网站和应用程序都开始使用视频播放器,使得用户可以在网页中直接观看视频。而Vue作为目前非常流行的前端框架之一,也提供了很多简便且实用的方法来实现视频播放器。下面,我们将简要介绍一下在Vue中实现视频播放器的方法。一、使用HTML5的video标签H
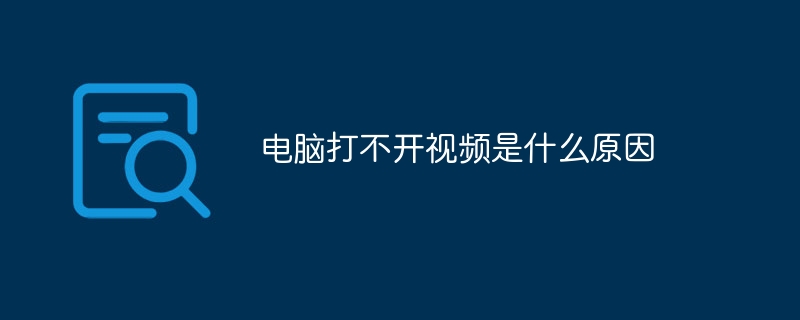
电脑打不开视频原因有:1、视频文件不完整;2、没有支持此视频的播放器;3、视频文件的后缀被修改过;4、视频文件关联不正确;5、没有使用插件。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
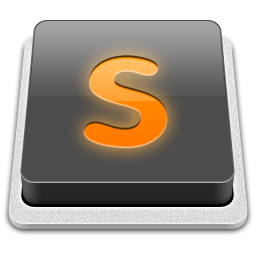
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment
