


The second-hand recycling website uses the product release and review function developed in PHP
The second-hand recycling website uses the product release and review function developed by PHP
With the rapid development of the second-hand commodity trading market, the second-hand recycling website has become the first choice platform for users to deal with idle items. However, in order to ensure the security and quality of transactions, the website needs to implement a product release review function. This article will introduce how to use PHP to develop the product release review function of a second-hand recycling website, and provide some code examples for reference.
- Requirements Analysis
Before implementing the product release review function, you first need to clarify the requirements. Generally speaking, the product release review function should have the following functions:
- After the user releases the product, the administrator needs to review and confirm whether it meets the transaction standards stipulated by the website;
- Administrators can review, pass or reject the published products, and give corresponding feedback information;
- Users can check whether the products they published have passed the review, and view the administrator's review opinions .
- Database design
In order to realize the product release review function, it is necessary to design the corresponding database table. The following is the design of the sample table:
- users table: stores user information, including user ID, user name, password, etc.
- products table: stores product information, including product ID, title, description, price, etc.
- product_review table: stores product review information, including review ID, product ID, review status (pass/reject), review opinions, etc.
- Page development
First, we need to design the page for the administrator to review the product. After logging in, the administrator can view the list of products to be reviewed. Click on a product to view details and conduct review. The following is a simple page example:
<?php // 待审核商品列表页面 session_start(); // 启动会话 // 检查管理员是否已登录,若未登录则跳转到登录页面 if (!isset($_SESSION['admin'])) { header("Location: login.php"); exit(); } // 查询待审核的商品列表 $query = "SELECT * FROM products WHERE status = 'pending'"; $result = mysqli_query($conn, $query); ?> <!DOCTYPE html> <html> <head> <title>商品审核</title> </head> <body> <h1 id="待审核商品列表">待审核商品列表</h1> <table> <tr> <th>商品ID</th> <th>标题</th> <th>价格</th> <th>操作</th> </tr> <?php while ($row = mysqli_fetch_assoc($result)): ?> <tr> <td><?php echo $row['id']; ?></td> <td><?php echo $row['title']; ?></td> <td><?php echo $row['price']; ?></td> <td><a href="review.php?id=<?php echo $row['id']; ?>">审核</a></td> </tr> <?php endwhile; ?> </table> </body> </html>
When the administrator clicks the "review" link of a product, it will jump to the review page. The following is a sample code for the audit page:
<?php // 审核具体商品页面 session_start(); // 启动会话 // 检查管理员是否已登录,若未登录则跳转到登录页面 if (!isset($_SESSION['admin'])) { header("Location: login.php"); exit(); } // 获取商品ID $id = $_GET['id']; // 查询商品信息 $query = "SELECT * FROM products WHERE id = $id"; $result = mysqli_query($conn, $query); $row = mysqli_fetch_assoc($result); // 提交审核结果 if ($_SERVER['REQUEST_METHOD'] === 'POST') { $status = $_POST['status']; $feedback = $_POST['feedback']; // 更新商品审核信息 $updateQuery = "UPDATE product_review SET status = '$status', feedback = '$feedback' WHERE product_id = $id"; mysqli_query($conn, $updateQuery); } ?> <!DOCTYPE html> <html> <head> <title>商品审核</title> </head> <body> <h1 id="审核商品">审核商品</h1> <h2><?php echo $row['title']; ?></h2> <p><?php echo $row['description']; ?></p> <form method="POST"> <input type="radio" name="status" value="approved"> 通过 <input type="radio" name="status" value="rejected"> 拒绝 <br> <textarea name="feedback" placeholder="审核意见"></textarea> <br> <input type="submit" value="提交"> </form> </body> </html>
- Database operation
In the audit page, the audit results need to be saved to the database. The following is a simple code example:
// 提交审核结果 if ($_SERVER['REQUEST_METHOD'] === 'POST') { $status = $_POST['status']; $feedback = $_POST['feedback']; // 更新商品审核信息 $updateQuery = "UPDATE product_review SET status = '$status', feedback = '$feedback' WHERE product_id = $id"; mysqli_query($conn, $updateQuery); }
The above example is just a simple demonstration, and the actual business logic and page design may be more complex. But through this example, we can understand how to use PHP to develop the product release review function of a second-hand recycling website. Hope this article helps you!
The above is the detailed content of The second-hand recycling website uses the product release and review function developed in PHP. For more information, please follow other related articles on the PHP Chinese website!
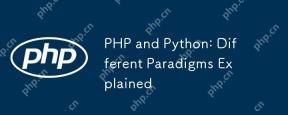
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
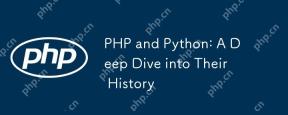
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
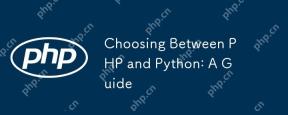
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
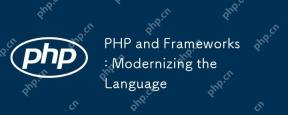
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
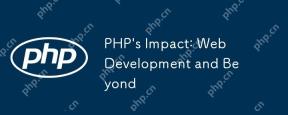
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
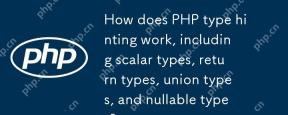
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
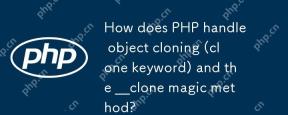
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
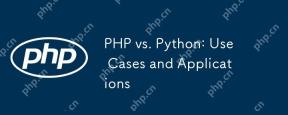
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
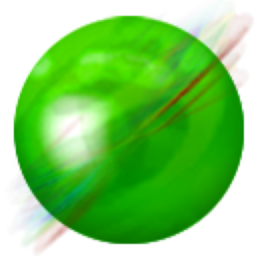
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
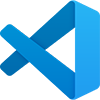
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.