


Configuration method of using Valgrind for memory leak detection on Linux systems
Introduction:
Memory leaks are one of the common problems in the software development process. It often causes programs to slow down or even crash. In order to discover and solve these problems in time, developers need tools to detect memory leaks. Under Linux systems, a widely used tool is Valgrind. This article will introduce how to configure and use Valgrind to detect memory leaks, and demonstrate the specific operation process through code examples.
Step 1: Install Valgrind
Installing Valgrind on a Linux system is very simple. We can install Valgrind directly through package management tools such as apt or yum. On Ubuntu, you can install it with the following command:
sudo apt-get install valgrind
Step 2: Write a code example
To demonstrate the use of Valgrind, we write a simple C program. The function of the program is to create an integer array, and the memory occupied by the array is not released before the end of the program. The following is a code example:
#include <stdio.h> #include <stdlib.h> void create_array(int length) { int* array = malloc(length * sizeof(int)); for (int i = 0; i < length; i++) { array[i] = i + 1; } } int main() { create_array(100); return 0; }
In this example, we use malloc in the create_array function to allocate a memory, but the memory is not released before the end of the program.
Step 3: Use Valgrind for memory leak detection
Run the Valgrind command in the terminal to detect memory leaks. The following is the basic syntax of the Valgrind command:
valgrind [选项] [待检测的程序及参数]
Run Valgrind through the following command and detect our code example:
valgrind --leak-check=full ./a.out
In the above command, "--leak-check=full" means proceed For complete memory leak detection, "./a.out" means running the executable file named "a.out" in the current directory.
Step 4: Analyze the output results of Valgrind
Valgrind will output detailed memory leak detection results. The following is the output of Valgrind for our code example:
==18708== Memcheck, a memory error detector ==18708== Copyright (C) 2002-2017, and GNU GPL'd, by Julian Seward et al. ==18708== Using Valgrind-3.13.0 and LibVEX; rerun with -h for copyright info ==18708== Command: ./a.out ==18708== ==18708== ==18708== HEAP SUMMARY: ==18708== in use at exit: 400 bytes in 1 blocks ==18708== total heap usage: 1 allocs, 0 frees, 400 bytes allocated ==18708== ==18708== 400 bytes in 1 blocks are definitely lost in loss record 1 of 1 ==18708== at 0x4C2AB80: malloc (in /usr/lib/valgrind/vgpreload_memcheck-amd64-linux.so) ==18708== by 0x40059D: create_array (main.c:6) ==18708== by 0x4005A8: main (main.c:11) ==18708== ==18708== LEAK SUMMARY: ==18708== definitely lost: 400 bytes in 1 blocks ==18708== indirectly lost: 0 bytes in 0 blocks ==18708== possibly lost: 0 bytes in 0 blocks ==18708== still reachable: 0 bytes in 0 blocks ==18708== suppressed: 0 bytes in 0 blocks ==18708== ==18708== For counts of detected and suppressed errors, rerun with: -v ==18708== ERROR SUMMARY: 1 errors from 1 contexts (suppressed: 0 from 0)
The output of Valgrind contains the following important information:
- HEAP SUMMARY: used to illustrate memory usage. In this example, 400 bytes of memory are still in use when the program exits, and only one memory allocation has been made.
- LEAK SUMMARY: Used to summarize memory leak situations. In this example, 400 bytes of memory are not freed at the end of the code example.
- ERROR SUMMARY: used to display a summary of the error message. In this example, Valgrind detected an error.
Conclusion:
Valgrind is a powerful tool that can help us promptly discover and solve memory related problems such as memory leaks. This article describes how to install, configure, and use Valgrind for memory leak detection on a Linux system and illustrates it with a simple code example. Hopefully this content will help developers better manage memory and debug.
The above is the detailed content of How to configure memory leak detection on Linux systems using Valgrind. For more information, please follow other related articles on the PHP Chinese website!
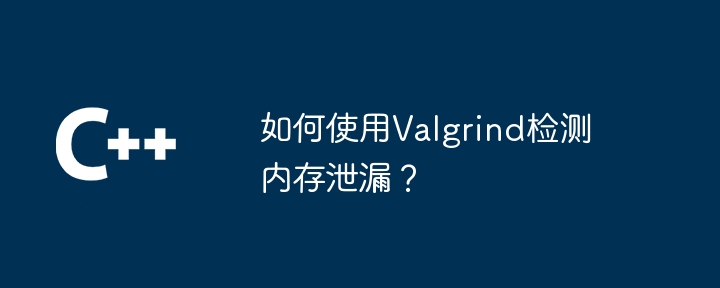
Valgrind通过模拟内存分配和释放来检测内存泄漏和错误,使用步骤如下:安装Valgrind:从官方网站下载并安装适用于您操作系统的版本。编译程序:使用Valgrind标志(如gcc-g-omyprogrammyprogram.c-lstdc++)编译程序。分析程序:使用valgrind--leak-check=fullmyprogram命令分析已编译的程序。检查输出:Valgrind将在程序执行后生成报告,显示内存泄漏和错误信息。
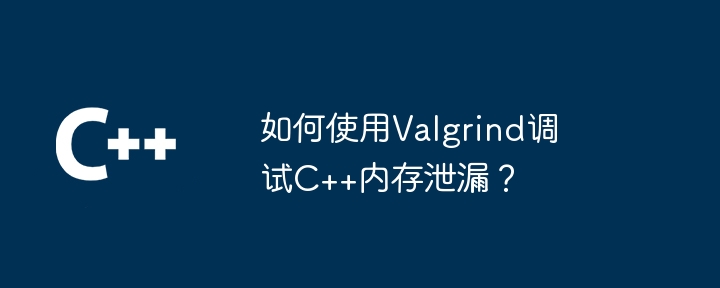
如何使用Valgrind调试C++内存泄漏Valgrind是一个功能强大的内存调试器,可用于检测C++程序中的内存泄漏、非法使用和分配问题。下面介绍如何使用Valgrind调试C++内存泄漏:1.安装Valgrind使用以下命令安装Valgrind:sudoaptinstallvalgrind2.编译和调试在编译程序时,添加-g标记以生成调试信息:g++-gmy_program.cpp-omy_program然后,使用Valgrind运行程序,并使用-
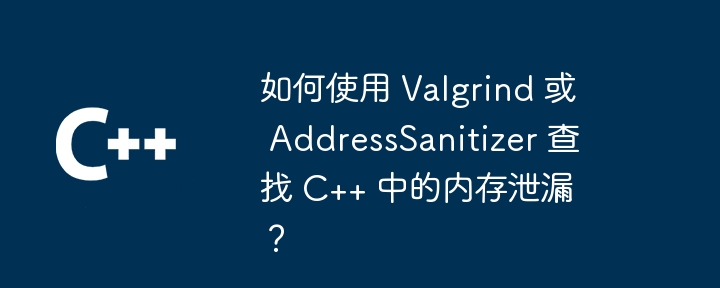
要查找C++中的内存泄漏,可以利用Valgrind和AddressSanitizer。Valgrind动态检测泄漏,显示地址、大小和调用栈。AddressSanitizer是一个Clang编译器插件,检测内存错误和泄漏。要启用ASan泄漏检查,请在编译时使用--leak-check=full选项,该选项将在程序运行后报告泄漏。
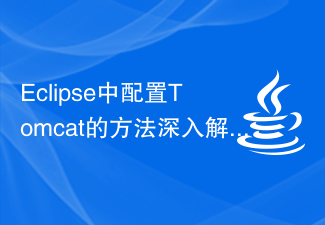
详解Eclipse中Tomcat的配置方法概述:Eclipse是广泛使用的集成开发环境(IDE)之一,而Tomcat是一个常用的JavaWeb应用服务器。在开发Web应用程序时,通常需要将Tomcat集成到Eclipse中以便进行调试和测试。本文将详细介绍如何在Eclipse中配置Tomcat,并提供一些具体的代码示例。配置Tomcat:下载和安装Tomc
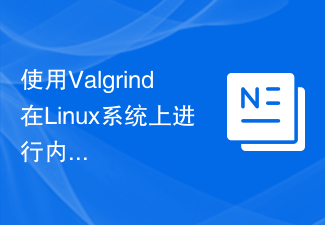
使用Valgrind在Linux系统上进行内存泄漏检测的配置方法引言:内存泄漏是软件开发过程中常见的问题之一。它通常会导致程序运行变慢,甚至崩溃。为了及时发现和解决这些问题,开发人员需要借助工具来进行内存泄漏的检测。在Linux系统下,一个广泛使用的工具就是Valgrind。本文将介绍如何配置和使用Valgrind进行内存泄漏的检测,并通过代码示例来展示具体
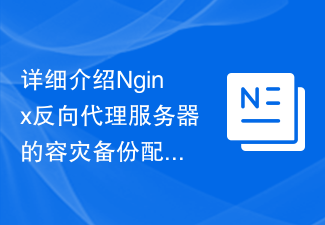
Nginx反向代理服务器的容灾备份配置方法详解概述在构建Web应用的环境中,高可用性和容灾备份是至关重要的。Nginx作为一款高性能的反向代理服务器,拥有强大的容灾备份配置功能,可以确保系统在服务器故障时持续可用。本文将介绍Nginx反向代理服务器的容灾备份配置方法,详细说明如何使用备份服务器保证系统的高可用性。安装Nginx首先,需要确保你的服务器上已经安
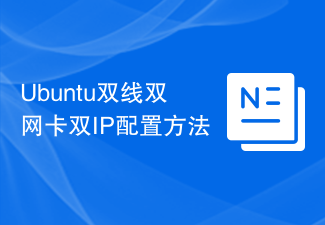
Ubuntu双线双网卡双IP配置方法,需要具体代码示例Ubuntu是一种流行的开源操作系统,可以在桌面和服务器环境中使用。配置双线双网卡双IP可以实现网络负载均衡和冗余备份,提高网络的可靠性和性能。本文将介绍在Ubuntu系统中如何配置双线双网卡双IP,并提供具体的代码示例。首先,我们需要查看系统中可用的网卡设备。打开终端,运行以下命令:$ifconfig
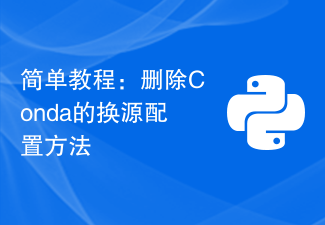
简单教程:删除Conda的换源配置方法Conda是一个非常强大的开源软件包管理系统,它可以帮助我们轻松安装、管理和使用各种不同的软件包。然而,在使用Conda时,有时我们需要更换软件包的源,以加快下载速度或者解决某些特定的问题。但是,如果不正确地更换源或者不再需要更换源时忘记撤销操作,可能会导致一些不必要的问题。本文将带您了解如何删除Conda的换源配置方法


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
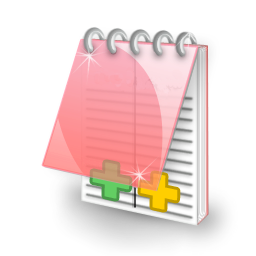
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
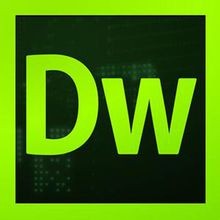
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
