


PHP error handling and exception logging in small program development
PHP error handling and exception logging in mini program development
With the continuous popularity of mini programs, more and more developers are beginning to use the PHP language to develop mini program backends. During development, error handling and exception logging are crucial. This article will introduce how to handle PHP errors and record exception logs in small program development, and give corresponding code examples.
1. PHP error handling
- Error reporting settings
In PHP, we can modify error_reporting
and display_errors
To control the reporting level and display mode of errors. When we develop small programs, we usually hope that errors can be discovered and fixed in a timely manner in the development environment, and specific error messages are not displayed in the production environment to ensure security. The following is a sample code for error reporting settings:
// 开发环境中显示所有错误 error_reporting(E_ALL); ini_set('display_errors', 'On'); // 生产环境中不显示错误 error_reporting(0); ini_set('display_errors', 'Off');
- Custom error handling function
In addition to using PHP's built-in error handling function, we can also customize error handling function to output error information or do other processing according to actual needs. The following is a sample code for a custom error handling function:
function customErrorHandler($severity, $message, $file, $line) { echo "错误信息:{$message},文件:{$file},行号:{$line}"; } // 设置错误处理函数 set_error_handler("customErrorHandler");
The above code outputs error information to the browser, and you can modify the output method as needed.
2. Exception logging
In the process of developing small programs, we need to record exception information so that we can troubleshoot and fix it when necessary. The following is a simple example code for exception logging:
function customExceptionHandler($exception) { $message = $exception->getMessage(); $file = $exception->getFile(); $line = $exception->getLine(); $trace = $exception->getTraceAsString(); $logMessage = "异常信息:{$message} 文件:{$file} 行号:{$line} 堆栈跟踪: {$trace} "; // 写入日志文件 file_put_contents('/path/to/error.log', $logMessage, FILE_APPEND); } // 设置异常处理函数 set_exception_handler("customExceptionHandler");
The above code records exception information to the specified log file. You can modify the path and file name of the log file as needed.
3. Application of error handling and exception logging
In actual development, we usually set error handling and exception handling functions in the project entry file. The following is a complete sample code:
// 错误报告设置 error_reporting(E_ALL); ini_set('display_errors', 'On'); // 自定义错误处理函数 function customErrorHandler($severity, $message, $file, $line) { echo "错误信息:{$message},文件:{$file},行号:{$line}"; } // 自定义异常处理函数 function customExceptionHandler($exception) { $message = $exception->getMessage(); $file = $exception->getFile(); $line = $exception->getLine(); $trace = $exception->getTraceAsString(); $logMessage = "异常信息:{$message} 文件:{$file} 行号:{$line} 堆栈跟踪: {$trace} "; // 写入日志文件 file_put_contents('/path/to/error.log', $logMessage, FILE_APPEND); } // 设置错误处理函数 set_error_handler("customErrorHandler"); // 设置异常处理函数 set_exception_handler("customExceptionHandler"); // 其他代码...
Through the above settings, we can promptly discover and solve PHP errors when developing small programs, and at the same time record the exception information into the log file to facilitate subsequent troubleshooting. .
Summary:
For PHP error handling and exception logging in small program development, it is very important to set the error reporting level, custom error handling functions and exception handling functions. Reasonably set the error reporting level and display method, and distinguish between development and production environments; customize error handling and exception handling functions to output error information or record exception logs according to actual needs. The sample code provided above can help you better handle errors and record exception information, and improve the efficiency and quality of small program development.
The above is the detailed content of PHP error handling and exception logging in small program development. For more information, please follow other related articles on the PHP Chinese website!
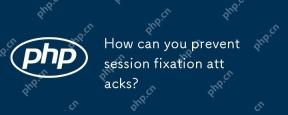
Effective methods to prevent session fixed attacks include: 1. Regenerate the session ID after the user logs in; 2. Use a secure session ID generation algorithm; 3. Implement the session timeout mechanism; 4. Encrypt session data using HTTPS. These measures can ensure that the application is indestructible when facing session fixed attacks.
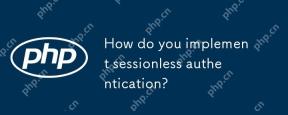
Implementing session-free authentication can be achieved by using JSONWebTokens (JWT), a token-based authentication system where all necessary information is stored in the token without server-side session storage. 1) Use JWT to generate and verify tokens, 2) Ensure that HTTPS is used to prevent tokens from being intercepted, 3) Securely store tokens on the client side, 4) Verify tokens on the server side to prevent tampering, 5) Implement token revocation mechanisms, such as using short-term access tokens and long-term refresh tokens.
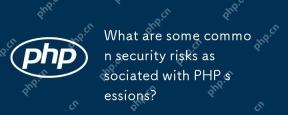
The security risks of PHP sessions mainly include session hijacking, session fixation, session prediction and session poisoning. 1. Session hijacking can be prevented by using HTTPS and protecting cookies. 2. Session fixation can be avoided by regenerating the session ID before the user logs in. 3. Session prediction needs to ensure the randomness and unpredictability of session IDs. 4. Session poisoning can be prevented by verifying and filtering session data.
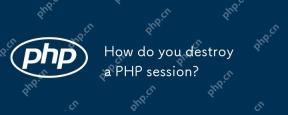
To destroy a PHP session, you need to start the session first, then clear the data and destroy the session file. 1. Use session_start() to start the session. 2. Use session_unset() to clear the session data. 3. Finally, use session_destroy() to destroy the session file to ensure data security and resource release.
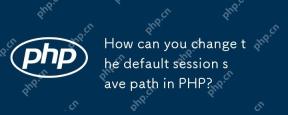
How to change the default session saving path of PHP? It can be achieved through the following steps: use session_save_path('/var/www/sessions');session_start(); in PHP scripts to set the session saving path. Set session.save_path="/var/www/sessions" in the php.ini file to change the session saving path globally. Use Memcached or Redis to store session data, such as ini_set('session.save_handler','memcached'); ini_set(
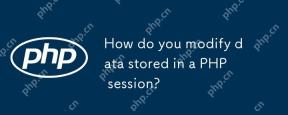
TomodifydatainaPHPsession,startthesessionwithsession_start(),thenuse$_SESSIONtoset,modify,orremovevariables.1)Startthesession.2)Setormodifysessionvariablesusing$_SESSION.3)Removevariableswithunset().4)Clearallvariableswithsession_unset().5)Destroythe
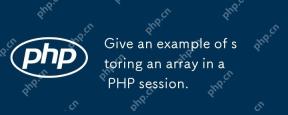
Arrays can be stored in PHP sessions. 1. Start the session and use session_start(). 2. Create an array and store it in $_SESSION. 3. Retrieve the array through $_SESSION. 4. Optimize session data to improve performance.
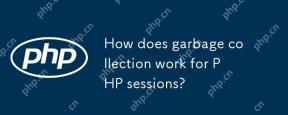
PHP session garbage collection is triggered through a probability mechanism to clean up expired session data. 1) Set the trigger probability and session life cycle in the configuration file; 2) You can use cron tasks to optimize high-load applications; 3) You need to balance the garbage collection frequency and performance to avoid data loss.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
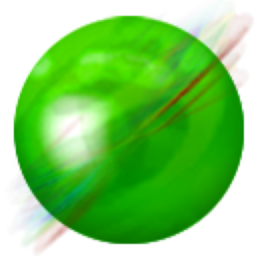
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
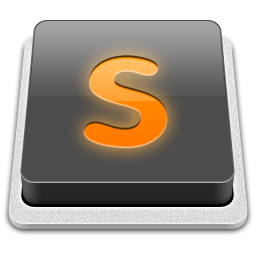
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!
