


MySQL creates an email subscription table to implement email subscription function
MySQL creates an email subscription table to implement the email subscription function
In a website or application, the email subscription function is usually used to allow users to subscribe to specific content updates or receive the latest news and information. In order to achieve this function, we can use the MySQL database to create an email subscription table and write the corresponding code to handle the subscription request.
First, we need to create a database table to store subscription information. The following is a sample SQL code for creating a table named "subscribers":
CREATE TABLE subscribers ( id INT AUTO_INCREMENT PRIMARY KEY, email VARCHAR(255) NOT NULL, subscribed_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
In the above code, we define a table named "subscribers", which has three columns: id , email and subscribed_at. Among them, id is an auto-incremented primary key, email is used to store the subscriber's email address, and subscribed_at is used to record the timestamp of the subscription.
Then, we need to write some code to handle the subscription request. Here is a sample PHP code to receive a subscription request from a user and insert it into the "subscribers" table:
<?php // 获取用户提交的电子邮件地址 $email = $_POST['email']; // 检查电子邮件地址是否为空 if(empty($email)) { echo "请输入有效的电子邮件地址"; exit; } // 连接到MySQL数据库 $host = 'localhost'; $user = 'your_username'; $password = 'your_password'; $dbname = 'your_database_name'; $conn = mysqli_connect($host, $user, $password, $dbname); // 检查数据库连接是否成功 if(!$conn) { die("数据库连接失败:" . mysqli_connect_error()); } // 插入订阅者信息到数据库表中 $sql = "INSERT INTO subscribers (email) VALUES ('$email')"; if(mysqli_query($conn, $sql)) { echo "订阅成功!"; } else { echo "订阅失败:" . mysqli_error($conn); } // 关闭数据库连接 mysqli_close($conn); ?>
In the above code, we first get the email from the form submitted by the user address. We then connect to the MySQL database and insert the subscribers' email addresses into the "subscribers" table. If the insertion operation is successful, we will output a subscription success message; if the insertion fails, we will output the corresponding error message.
Finally, we can embed the above code into the corresponding page or interface in our website or application to implement the email subscription function.
Summary:
By using a MySQL database to create an email subscription table, we can easily store and manage subscribers' email addresses. Combined with the corresponding code processing logic, we can implement the email subscription function and provide users with subscription-related services. Of course, this is just a simple example of the email subscription function. Please modify and expand it according to actual needs.
The above is the detailed content of MySQL creates an email subscription table to implement email subscription function. For more information, please follow other related articles on the PHP Chinese website!
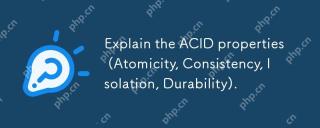
ACID attributes include atomicity, consistency, isolation and durability, and are the cornerstone of database design. 1. Atomicity ensures that the transaction is either completely successful or completely failed. 2. Consistency ensures that the database remains consistent before and after a transaction. 3. Isolation ensures that transactions do not interfere with each other. 4. Persistence ensures that data is permanently saved after transaction submission.
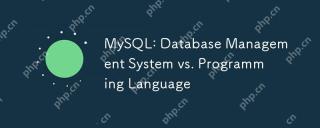
MySQL is not only a database management system (DBMS) but also closely related to programming languages. 1) As a DBMS, MySQL is used to store, organize and retrieve data, and optimizing indexes can improve query performance. 2) Combining SQL with programming languages, embedded in Python, using ORM tools such as SQLAlchemy can simplify operations. 3) Performance optimization includes indexing, querying, caching, library and table division and transaction management.
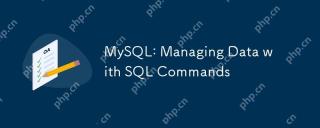
MySQL uses SQL commands to manage data. 1. Basic commands include SELECT, INSERT, UPDATE and DELETE. 2. Advanced usage involves JOIN, subquery and aggregate functions. 3. Common errors include syntax, logic and performance issues. 4. Optimization tips include using indexes, avoiding SELECT* and using LIMIT.
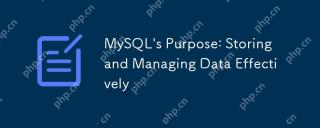
MySQL is an efficient relational database management system suitable for storing and managing data. Its advantages include high-performance queries, flexible transaction processing and rich data types. In practical applications, MySQL is often used in e-commerce platforms, social networks and content management systems, but attention should be paid to performance optimization, data security and scalability.
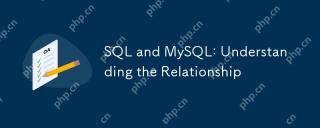
The relationship between SQL and MySQL is the relationship between standard languages and specific implementations. 1.SQL is a standard language used to manage and operate relational databases, allowing data addition, deletion, modification and query. 2.MySQL is a specific database management system that uses SQL as its operating language and provides efficient data storage and management.
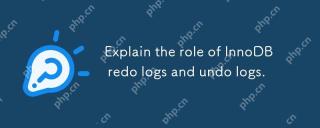
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
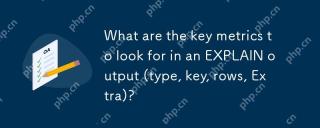
Key metrics for EXPLAIN commands include type, key, rows, and Extra. 1) The type reflects the access type of the query. The higher the value, the higher the efficiency, such as const is better than ALL. 2) The key displays the index used, and NULL indicates no index. 3) rows estimates the number of scanned rows, affecting query performance. 4) Extra provides additional information, such as Usingfilesort prompts that it needs to be optimized.
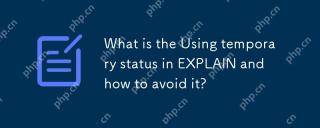
Usingtemporary indicates that the need to create temporary tables in MySQL queries, which are commonly found in ORDERBY using DISTINCT, GROUPBY, or non-indexed columns. You can avoid the occurrence of indexes and rewrite queries and improve query performance. Specifically, when Usingtemporary appears in EXPLAIN output, it means that MySQL needs to create temporary tables to handle queries. This usually occurs when: 1) deduplication or grouping when using DISTINCT or GROUPBY; 2) sort when ORDERBY contains non-index columns; 3) use complex subquery or join operations. Optimization methods include: 1) ORDERBY and GROUPB


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
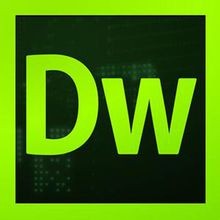
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
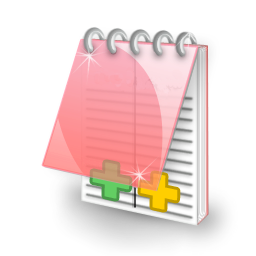
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.