


Use PHP to develop a second-hand recycling website to implement full-text search function
Use PHP to develop a second-hand recycling website to implement full-text search function
In second-hand recycling websites, users often need to search for a specific item or keyword to find second-hand products that meet their needs. Developing a website with full-text search capabilities can help users find what they need quickly. This article will introduce how to use PHP language to achieve this function and provide code examples.
1. Establish a database
First, you need to establish a database to store the second-hand product information released by users. Suppose we have created a database named "goods", which contains a table named "goods_table", which contains the following fields:
id: unique identifier of the product, using an auto-increasing integer type ;
title: Product title, using VARCHAR type;
description: Product description, using TEXT type.
2. Create a search page
First, we need to create a search page for users to enter keywords to search. You can create a file named "search.php" and write the following code in the file:
<html> <head> <title>二手回收网站-搜索</title> </head> <body> <h1 id="二手回收网站搜索">二手回收网站搜索</h1> <form method="GET" action="result.php"> <input type="text" name="keyword" placeholder="请输入关键词"> <input type="submit" value="搜索"> </form> </body> </html>
3. Query the database
Next, we need to write PHP code to query the database and Return matching results. Create a file called "result.php" and write the following code:
<?php // 连接数据库 $servername = "localhost"; $username = "root"; $password = ""; $database = "goods"; $conn = new mysqli($servername, $username, $password, $database); if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 获取用户输入的关键词 $keyword = $_GET['keyword']; // 查询数据库 $sql = "SELECT * FROM goods_table WHERE MATCH(title, description) AGAINST('$keyword' IN BOOLEAN MODE)"; $result = $conn->query($sql); // 打印搜索结果 if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "商品ID: " . $row["id"]. "<br>标题: " . $row["title"]. "<br>描述: " . $row["description"]. "<br><br>"; } } else { echo "未找到匹配的商品"; } $conn->close(); ?>
In this code, we first connect to the database named "goods". Then, obtain the keywords entered by the user through the GET method. Next, we use MySQL's FULLTEXT index to perform full-text search and return matching results. Finally, print out the search results through a loop.
So far, we have implemented a second-hand recycling website with full-text search function. Users can enter keywords on the search page, and the website will query the database based on the keywords and return matching product information.
Summary:
Through PHP language and MYSQL database, we can easily implement a second-hand recycling website with full-text search function. Through this function, users can quickly find the second-hand products they need. I believe the above code examples can help you develop an efficient second-hand recycling website.
The above is the detailed content of Use PHP to develop a second-hand recycling website to implement full-text search function. For more information, please follow other related articles on the PHP Chinese website!
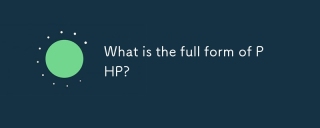
The article discusses PHP, detailing its full form, main uses in web development, comparison with Python and Java, and its ease of learning for beginners.
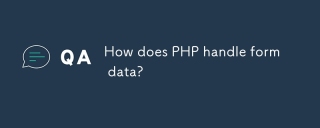
PHP handles form data using $\_POST and $\_GET superglobals, with security ensured through validation, sanitization, and secure database interactions.
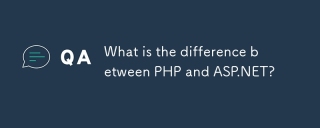
The article compares PHP and ASP.NET, focusing on their suitability for large-scale web applications, performance differences, and security features. Both are viable for large projects, but PHP is open-source and platform-independent, while ASP.NET,
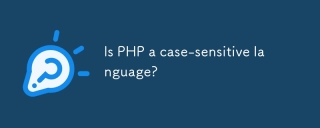
PHP's case sensitivity varies: functions are insensitive, while variables and classes are sensitive. Best practices include consistent naming and using case-insensitive functions for comparisons.
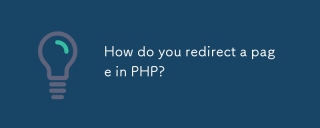
The article discusses various methods for page redirection in PHP, focusing on the header() function and addressing common issues like "headers already sent" errors.
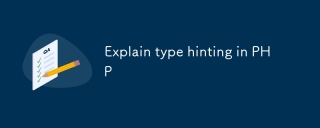
Article discusses type hinting in PHP, a feature for specifying expected data types in functions. Main issue is improving code quality and readability through type enforcement.

The article discusses PHP Data Objects (PDO), an extension for database access in PHP. It highlights PDO's role in enhancing security through prepared statements and its benefits over MySQLi, including database abstraction and better error handling.
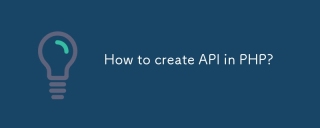
Article discusses creating and securing PHP APIs, detailing steps from endpoint definition to performance optimization using frameworks like Laravel and best security practices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
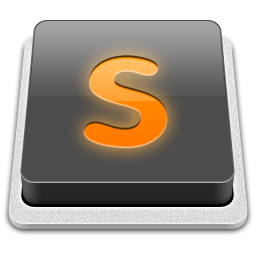
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
