


PHP development of enterprise resource planning (ERP) system to build sales forecasting function
Introduction:
With the expansion of enterprise scale and fierce market competition, understanding and predicting sales changes have become increasingly important. An important part of business management. In order to meet the needs of enterprises for sales forecasting functions, this article will introduce a method to implement the sales forecasting function in an enterprise resource planning (ERP) system developed using PHP, and provide corresponding code examples.
1. Functional Requirements Analysis
Before building an ERP system with sales forecasting function, it is first necessary to clarify the functional requirements. The sales forecast function usually includes the following aspects:
- Data collection: Obtain sales data, including historical sales data, market environment data, etc.
- Data cleaning: Clean and process the collected data, including removing outliers, processing missing values, etc.
- Data analysis: Carry out sales forecast analysis based on historical sales data and market environment data, such as trend analysis, seasonal analysis, regression analysis, etc.
- Prediction results display: Display the prediction results in charts, tables, etc., so that corporate management can refer to them for decision-making.
2. Technical Implementation
In the development of the ERP system, PHP is used as the back-end development language and combined with the database for data storage and management. The specific implementation steps are as follows:
- Data collection and storage
Use ready-made open source data collection tools or self-developed data collection modules to obtain sales data from various channels and store it in the database. Taking MySQL as an example, assume that we have created a data table named sales. The table structure is as follows:
CREATE TABLE sales ( id INT PRIMARY KEY AUTO_INCREMENT, date DATE, sales_amount DECIMAL(10, 2) );
- Data cleaning and processing
According to actual needs, write PHP code to The collected data is cleaned and processed, including outlier processing and missing value processing. The following is a sample code:
// 查询数据库中的销售数据 $query = "SELECT * FROM sales"; $result = mysqli_query($conn, $query); // 清洗处理数据 while ($row = mysqli_fetch_assoc($result)) { $date = $row['date']; $salesAmount = $row['sales_amount']; // 处理异常值 if ($salesAmount < 0 || $salesAmount > 1000000) { continue; // 跳过异常值 } // 处理缺失值 if (empty($salesAmount)) { $salesAmount = 0; } // 存储处理后的数据 $query = "INSERT INTO cleaned_sales (date, sales_amount) VALUES ('$date', $salesAmount)"; mysqli_query($conn, $query); }
- Data analysis
Perform sales forecast analysis based on the cleaned data. Here we take trend analysis as an example, using a linear regression model to conduct trend analysis. The following is a sample code:
// 查询数据库中的清洗后的销售数据 $query = "SELECT * FROM cleaned_sales"; $result = mysqli_query($conn, $query); // 准备数据 $x = []; // 日期 $y = []; // 销售金额 while ($row = mysqli_fetch_assoc($result)) { $x[] = strtotime($row['date']); $y[] = $row['sales_amount']; } // 线性回归分析 $coefficients = linear_regression($x, $y); // 输出结果 echo "斜率:".$coefficients['slope']."<br>"; echo "截距:".$coefficients['intercept']."<br>"; function linear_regression($x, $y) { $n = count($x); // 数据点个数 $sumX = array_sum($x); $sumY = array_sum($y); $sumXY = 0; $sumXX = 0; for ($i = 0; $i < $n; ++$i) { $sumXY += $x[$i] * $y[$i]; $sumXX += $x[$i] * $x[$i]; } $slope = ($n * $sumXY - $sumX * $sumY) / ($n * $sumXX - $sumX * $sumX); $intercept = ($sumY - $slope * $sumX) / $n; return ['slope' => $slope, 'intercept' => $intercept]; }
- Prediction result display
According to the analysis results, the prediction results are displayed in charts, tables, etc., to facilitate the viewing and decision-making of enterprise management. Here we take chart display as an example, using the Chart.js library to generate a line chart. The following is a sample code:
// 查询数据库中的预测数据 $query = "SELECT * FROM sales_forecast"; $result = mysqli_query($conn, $query); // 准备数据 $labels = []; // 日期 $data = []; // 预测销售金额 while ($row = mysqli_fetch_assoc($result)) { $labels[] = $row['date']; $data[] = $row['sales_amount']; } // 生成折线图 echo '<canvas id="salesForecastChart"></canvas>'; // 绘制折线图 echo '<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>'; echo '<script>'; echo 'var ctx = document.getElementById("salesForecastChart").getContext("2d");'; echo 'var myChart = new Chart(ctx, {'; echo ' type: "line",'; echo ' data: {'; echo ' labels: '.json_encode($labels).','; echo ' datasets: [{'; echo ' label: "Sales Forecast",'; echo ' data: '.json_encode($data).','; echo ' fill: false,'; echo ' backgroundColor: "rgba(75, 192, 192, 0.4)",'; echo ' borderColor: "rgba(75, 192, 192, 1)",'; echo ' borderWidth: 2'; echo ' }]'; echo ' },'; echo ' options: {'; echo ' responsive: true,'; echo ' scales: {'; echo ' y: {'; echo ' beginAtZero: true'; echo ' }'; echo ' }'; echo ' }'; echo '});'; echo '</script>';
3. Summary
Through the above steps, we successfully implemented the PHP development of the ERP system with sales forecasting function. In practical applications, the functions can be further improved according to specific needs and combined with other modules, such as marketing, warehousing management, etc., to build a complete enterprise resource planning system.
It is worth noting that the sample code provided in this article is for reference only, and needs to be modified and optimized based on specific business logic during the actual development process. I hope this article can provide some ideas and methods for PHP developers to build an ERP system with sales forecasting function.
The above is the detailed content of PHP development for building an enterprise resource planning (ERP) system with sales forecasting capabilities. For more information, please follow other related articles on the PHP Chinese website!
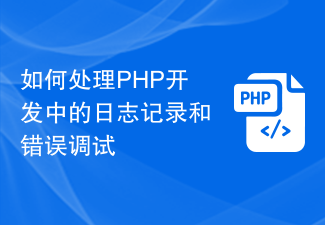
如何处理PHP开发中的日志记录和错误调试引言:在PHP开发过程中,日志记录和错误调试是非常重要的环节。良好的日志记录可以方便开发人员追踪代码执行情况、定位问题以及进行性能分析。而错误调试则可以帮助开发人员快速定位和解决代码中的bug。本文将介绍如何在PHP开发过程中进行日志记录和错误调试,并给出具体的代码示例。一、日志记录设置日志记录的格式在PHP中,可以使
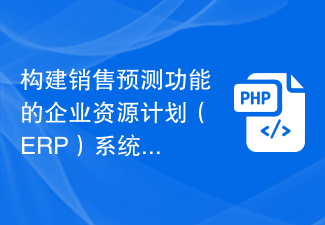
构建销售预测功能的企业资源计划(ERP)系统的PHP开发引言:随着企业规模的扩大和市场竞争的激烈,了解和预测销售变化越来越成为企业管理的重要一环。为了满足企业对销售预测功能的需求,本文将介绍一种利用PHP开发的企业资源计划(ERP)系统中销售预测功能的实现方法,并提供相应的代码示例。一、功能需求分析在构建销售预测功能的ERP系统前,首先需要明确功能需求。销售
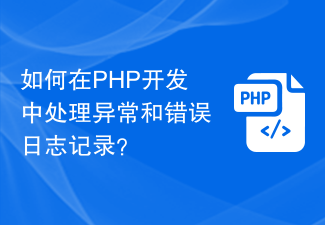
如何在PHP开发中处理异常和错误日志记录?PHP作为一种非常流行的后端编程语言,广泛应用于Web开发领域。在开发过程中,我们经常需要处理异常和记录错误日志,以便及时发现和解决问题。本文将介绍如何在PHP开发中处理异常和错误日志记录的最佳实践。一、异常处理在PHP中,异常是一种用于处理错误情况的特殊对象。当代码遇到无法处理的错误时,我们可以抛出一个异常,并在合
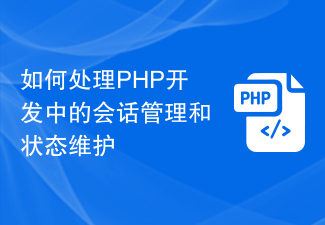
如何处理PHP开发中的会话管理和状态维护,需要具体代码示例随着互联网的发展,网站和应用程序的交互变得越来越复杂,用户需求也不断增加。在这个过程中,会话管理和状态维护变得至关重要。PHP作为一种常用的服务器端脚本语言,具有强大的会话管理和状态维护能力。本文将介绍在PHP开发中如何处理会话管理和状态维护,并提供具体的代码示例。会话管理是指通过维护会话信息来跟踪用
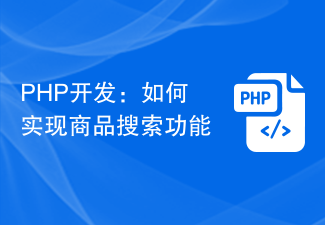
PHP开发:如何实现商品搜索功能商品搜索功能在电商网站中非常常见,它为用户提供了便捷的商品查询和浏览体验。在本文中,我们将探讨如何使用PHP来实现一个简单的商品搜索功能,并且提供具体的代码示例。一、数据库准备首先,我们需要准备一个适用于商品搜索的数据库。数据库中应该包含商品的相关信息,比如商品名称、价格、描述等。我们可以使用MySQL等关系型数据库来存储和管
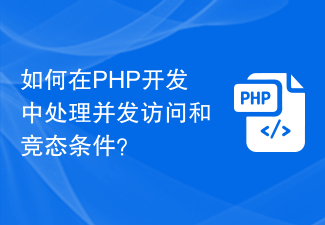
如何在PHP开发中处理并发访问和竞态条件?概述:在PHP开发中,处理并发访问和竞态条件是至关重要的。并发访问是指多个用户同时访问同一个资源,而竞态条件是指多个线程或进程在访问和操作共享资源时,由于执行顺序不确定而导致的结果不一致的情况。本文将介绍一些常见的处理并发访问和竞态条件的方法,以帮助开发者更好地处理这些问题。一、使用互斥锁互斥锁是一种用于保护共享资源
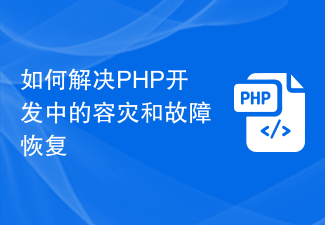
如何解决PHP开发中的容灾和故障恢复在PHP开发中,容灾和故障恢复是非常重要的方面。当系统遇到故障或意外停机时,我们需要有一套有效的解决办法,以确保系统能够快速恢复正常运行,避免造成严重的损失。本文将介绍一些常用的PHP容灾和故障恢复技术,并给出具体的代码示例。一、故障检测与恢复监控应用程序的健康状态在PHP开发中,我们可以使用一些监控工具来检测应用程序的健
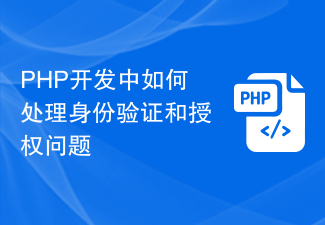
在当今互联网时代,随着互联网的普及和应用程序的发展,越来越多的网站和应用需要实现用户的身份验证和授权功能。特别是在PHP开发中,身份验证和授权问题被广泛关注。本文将探讨如何解决PHP开发中的身份验证和授权问题。首先,我们来了解什么是身份验证和授权。身份验证是指确认用户的身份,确保用户是合法的。授权是指给予用户在系统中进行某些操作的权限。对于PHP开发中的身份


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
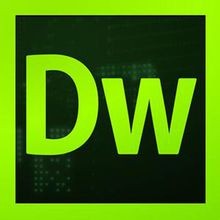
Dreamweaver CS6
Visual web development tools
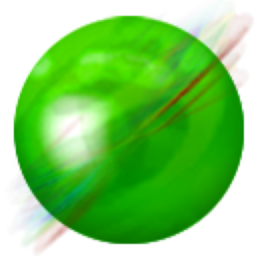
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
