


PHP implements the tag classification function in the knowledge question and answer website.
PHP implements the tag classification function in the knowledge question and answer website
With the development of the Internet, the knowledge question and answer website has gradually become one of the important channels for people to obtain information. On these sites, users can ask questions and get answers from other users. In order to better organize and manage questions, websites usually add tag categories to each question. This article will introduce how to use PHP to implement the tag classification function in a knowledge question and answer website.
First, we need to create corresponding tables in the database to store question and label data. In this example, we assume that our database is named "qa_database" and the tables are named "questions" and "tags". The "questions" table will store information about questions, including the question's title, content, creation time, etc. The "tags" table will store all available tags.
The following is the SQL statement to create the "questions" and "tags" tables:
CREATE TABLE `qa_database`.`questions` ( `id` INT NOT NULL AUTO_INCREMENT, `title` VARCHAR(100) NOT NULL, `content` TEXT NOT NULL, `created_at` TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP, PRIMARY KEY (`id`) ); CREATE TABLE `qa_database`.`tags` ( `id` INT NOT NULL AUTO_INCREMENT, `name` VARCHAR(50) NOT NULL, PRIMARY KEY (`id`) );
Next, we need to add a field to the questions table to store the relationship between questions and tags. We can add a field called "tag_id" to the "questions" table to store and identify the tag to which the question belongs.
ALTER TABLE `qa_database`.`questions` ADD COLUMN `tag_id` INT NULL AFTER `created_at`;
Now, we have prepared the basic structure of the database. Below we will implement the tag classification function through PHP code.
First, we need to connect to the database. The following code can be used to establish a connection to the database:
<?php $servername = "localhost"; $username = "your_username"; $password = "your_password"; $dbname = "qa_database"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 设置字符集 $conn->set_charset("utf8"); // 在这里执行其他操作... // 关闭连接 $conn->close(); ?>
Next, we can implement a function to get a list of all available tags:
<?php function getAllTags($conn) { $sql = "SELECT * FROM tags"; $result = $conn->query($sql); $tags = array(); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { $tags[] = $row; } } return $tags; } ?>
Then, we can implement a function to get List of questions under a specific tag:
<?php function getQuestionsByTag($conn, $tagId) { $sql = "SELECT * FROM questions WHERE tag_id = $tagId"; $result = $conn->query($sql); $questions = array(); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { $questions[] = $row; } } return $questions; } ?>
Finally, we can use these functions in the page to show the function of tag classification:
<?php // 获取所有标签 $tags = getAllTags($conn); // 获取第一个标签下的问题列表 $tagId = $tags[0]['id']; $questions = getQuestionsByTag($conn, $tagId); ?> <h1 id="标签分类">标签分类</h1> <!-- 标签列表 --> <ul> <?php foreach ($tags as $tag): ?> <li><a href="?tag=<?php echo $tag['id']; ?>"><?php echo $tag['name']; ?></a></li> <?php endforeach; ?> </ul> <!-- 问题列表 --> <ul> <?php foreach ($questions as $question): ?> <li><?php echo $question['title']; ?></li> <?php endforeach; ?> </ul>
With the above code example, we can use the above code example in the knowledge question and answer website Implement the function of label classification. Users can browse the corresponding list of questions by clicking on different tabs. Developers can also filter and categorize issues based on tags. This enables better organization and management of issues and improves user experience.
To sum up, it is not complicated to use PHP to implement the tag classification function in the knowledge question and answer website. We only need to create the corresponding database tables and fields, and implement the corresponding functions to achieve the association between tags and questions. In this way, issues can be better organized and managed, improving user experience. At the same time, developers can also filter and classify issues based on tags to further optimize website functions.
The above is the detailed content of PHP implements the tag classification function in the knowledge question and answer website.. For more information, please follow other related articles on the PHP Chinese website!
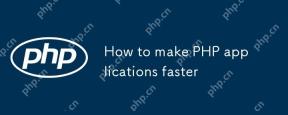
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
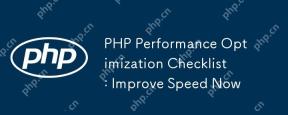
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
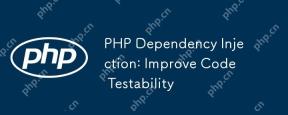
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
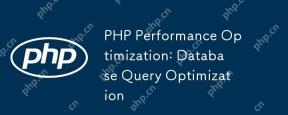
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
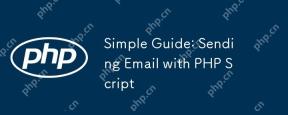
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
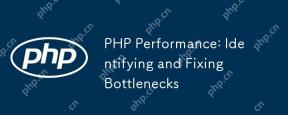
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
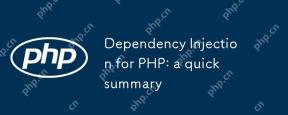
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
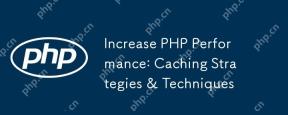
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
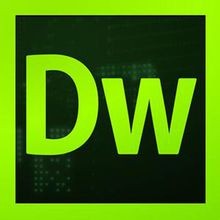
Dreamweaver CS6
Visual web development tools
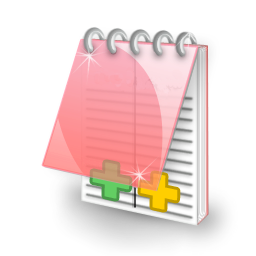
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
