


Using PHP to develop an enterprise resource planning (ERP) system that implements material requirements planning functions
Use PHP to develop an enterprise resource planning (ERP) system that implements material requirements planning functions
With the expansion of enterprise scale and the complexity of business, enterprises need to manage and control the procurement and use of materials more efficiently , to ensure the smooth progress of production. Material Requirements Planning (MRP) is an enterprise management tool used to determine and plan the materials required to meet production needs.
In order to automate the material requirements planning function, the PHP programming language can be used to develop an enterprise resource planning (ERP) system. This article will introduce how to use PHP to develop an ERP system that implements material requirements planning functions, and attach relevant code examples.
First, we need to establish a database to store the company's material information and demand planning data. You can use a MySQL database to create a database named "erp" and create the following two tables to store related data.
CREATE TABLE materials ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(100), stock INT, unit_price DECIMAL(10, 2) ); CREATE TABLE demands ( id INT AUTO_INCREMENT PRIMARY KEY, material_id INT, quantity INT, due_date DATE, FOREIGN KEY (material_id) REFERENCES materials(id) );
Next, we need to write PHP code to implement the core part of the material requirements planning function. We will use PHP's PDO library to connect to the database and write some functions to complete various operations.
The first is the function to query the material information in the material table.
function getMaterials() { $db = new PDO('mysql:host=localhost;dbname=erp', 'root', ''); $query = "SELECT * FROM materials"; $stmt = $db->prepare($query); $stmt->execute(); return $stmt->fetchAll(PDO::FETCH_ASSOC); }
The next step is the function to query the demand plan data in the demand plan table.
function getDemands() { $db = new PDO('mysql:host=localhost;dbname=erp', 'root', ''); $query = "SELECT * FROM demands"; $stmt = $db->prepare($query); $stmt->execute(); return $stmt->fetchAll(PDO::FETCH_ASSOC); }
Then there is a function that calculates the material requirements based on current inventory and demand planning data.
function calculateDemand($stock, $demand) { if ($stock >= $demand) { return 0; } else { return $demand - $stock; } }
Finally, it is a function that calculates the total amount of material requirements based on the material requirements and unit price.
function calculateTotalAmount($demand, $unitPrice) { return $demand * $unitPrice; }
The above are only part of the core functions we need to implement. According to actual needs and business processes, other functions can also be added, such as adding materials, editing demand plans, generating purchase orders, etc.
After writing the above code, we can create a page to display the material requirements planning data and calculation results.
$materials = getMaterials(); $demands = getDemands(); foreach ($materials as $material) { echo "物料名称: " . $material['name'] . "<br>"; echo "当前库存: " . $material['stock'] . "<br>"; foreach ($demands as $demand) { if ($demand['material_id'] == $material['id']) { $demandAmount = calculateDemand($material['stock'], $demand['quantity']); $totalAmount = calculateTotalAmount($demandAmount, $material['unit_price']); echo "需求计划: " . $demand['quantity'] . "<br>"; echo "物料需求量: " . $demandAmount . "<br>"; echo "物料需求总金额: " . $totalAmount . "<br>"; echo "<br>"; } } }
Through the above code example, we can implement a simple enterprise resource planning (ERP) system using the material requirements planning function developed in PHP. Enterprises can expand and improve according to actual needs, making the system more consistent with actual business processes and management needs.
To summarize, the material requirements planning function is very important for enterprises, and can help enterprises improve the efficiency of material management and the smooth progress of production. By using PHP to develop an ERP system that implements the material requirements planning function, automated material requirements planning can be implemented and relevant information such as material requirements and total amount can be calculated based on actual demand. This is very beneficial for enterprises to improve production efficiency and management effects.
The above is the detailed content of Using PHP to develop an enterprise resource planning (ERP) system that implements material requirements planning functions. For more information, please follow other related articles on the PHP Chinese website!
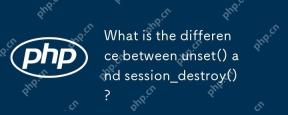
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
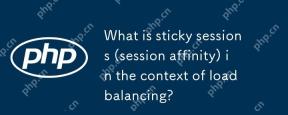
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
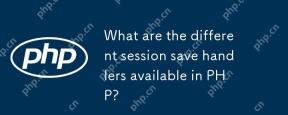
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
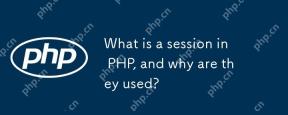
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
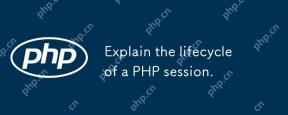
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
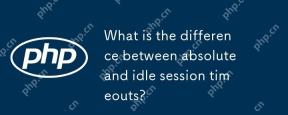
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
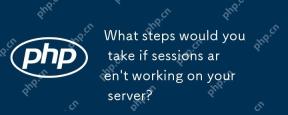
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
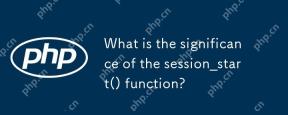
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
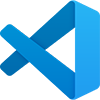
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
