Methods to improve cache efficiency in Go language
How to implement an efficient caching mechanism in Go language development
With the rapid development of the Internet, the challenges of high concurrency and large data volume have become problems that every developer must face. In scenarios where high concurrency and large amounts of data are processed, the caching mechanism has become one of the important means to improve system performance and response speed. As a popular programming language in Internet development today, Go language provides an efficient, concise, concurrent and safe programming method, and can also easily implement an efficient caching mechanism.
This article will introduce how to implement an efficient caching mechanism in Go language development, covering the following aspects:
- The principles and advantages of caching
- Using Go language Built-in Map implements caching
- Use third-party libraries to implement high-performance caching algorithms
- Cache update mechanism
- Cache expiration and invalidation handling
- Cache concurrency security
- Cache monitoring and statistics
1. Principles and advantages of caching
Cache is a way to temporarily store data, saving frequently used data in High-speed storage media for quick access and improved system performance. The main advantages of caching are as follows:
- Accelerate data access speed: The cache is usually located in the system's memory, and the read speed is much higher than disk or network requests, which can significantly improve the system response speed.
- Reduce database load: Caching can reduce frequent read requests to the database, thereby reducing the load on the database and improving the concurrency capability of the system.
- Improve system scalability: caching can reduce dependence on external resources, reduce system coupling, and facilitate system expansion and horizontal expansion.
2. Use the built-in Map of Go language to implement caching
In Go language, you can use the built-in Map type to implement a simple caching mechanism. By storing data in a Map, data reading and storage operations can be performed within O(1) time complexity. The following is a simple example:
package main import ( "fmt" "sync" ) type Cache struct { data map[string]interface{} lock sync.RWMutex expire int64 } func NewCache(expire int64) *Cache { return &Cache{ data: make(map[string]interface{}), expire: expire, } } func (c *Cache) Set(key string, value interface{}) { c.lock.Lock() defer c.lock.Unlock() c.data[key] = value } func (c *Cache) Get(key string) (interface{}, bool) { c.lock.RLock() defer c.lock.RUnlock() value, exist := c.data[key] return value, exist } func main() { cache := NewCache(3600) cache.Set("name", "Tom") value, exist := cache.Get("name") if exist { fmt.Println(value) } }
The above code uses Map as a storage container and ensures the concurrency security of data through read-write locks (sync.RWMutex). The cache expiration time can be set according to needs.
3. Use third-party libraries to implement high-performance caching algorithms
In addition to using the built-in Map to implement caching, you can also choose to use some third-party libraries to implement high-performance caching algorithms, such as Go The go-cache
library is widely used in the language. go-cache
Provides a rich cache operation interface and supports advanced functions such as expiration time and LRU mechanism. The following is an example of using the go-cache
library:
package main import ( "fmt" "github.com/patrickmn/go-cache" "time" ) func main() { c := cache.New(5*time.Minute, 10*time.Minute) c.Set("name", "Tom", cache.DefaultExpiration) value, exist := c.Get("name") if exist { fmt.Println(value) } }
The above code uses the go-cache
library to create a cache instance and set the survival time of cache items. and the time to clear expired items. You can choose an appropriate caching algorithm library based on specific needs.
4. Cache update mechanism
When implementing the cache mechanism, taking into account the real-time nature of the data, an effective cache update mechanism is required. Cache updates can be achieved in the following ways:
- Scheduled refresh: You can set the expiration time in the cache item. After the expiration time is reached, reload the data and refresh the cache. You can use the timer (
time.Ticker
) in thetime
package of the Go language to implement scheduled refresh. - Active update: When the user updates a certain data, the cache can be updated through active notification. You can use the publish-subscribe model (Pub-Sub) to implement the message notification mechanism.
- Delayed update: When the cache of a certain data expires, it is not updated immediately, but is delayed during the next access. Flag bits can be used to mark whether the cache needs to be updated.
5. Cache expiration and invalidation handling
Cache expiration is an important issue that needs attention in the caching mechanism. If the expired cache continues to be used, it may cause data inaccuracy. In Go, the cache expiration and invalidation issues can be handled in the following ways:
- Set expiration time: Set the expiration time in the cache item. After the expiration time is reached, the cache item is automatically cleared, ensuring Accuracy of data.
- Active refresh: When the cache item expires, you can reload the data through the active refresh mechanism and update the expiration time of the cache item.
6. Cache concurrency security
In high concurrency scenarios, cache concurrency security is a very important part. The Go language provides mechanisms such as mutex locks (sync.Mutex
) and read-write locks (sync.RWMutex
) to ensure data concurrency security. When accessing and updating the cache, locks need to be used appropriately to protect shared resources.
7. Cache monitoring and statistics
In order to better understand the performance and usage of the cache, you can monitor and collect statistics on the cache. This can be achieved in the following ways:
- Monitor the cache hit rate: count the number of cache hits and total access times, and calculate the cache hit rate to measure cache usage.
- Monitor the cache size: regularly count the size of the cache. When the cache size exceeds a certain threshold, an alarm will be issued or the capacity will be expanded.
- Monitor cache performance: Regularly monitor cache read and write performance, including read and write time consuming, concurrency and other indicators, to detect whether there are performance problems.
Summarize:
Implementing an efficient caching mechanism in Go language development can significantly improve system performance and response speed. Through reasonable caching strategies, cache update mechanisms and monitoring statistics, the system's resource utilization and user experience can be optimized. I hope this article can help readers better understand and practice the caching mechanism in Go language.
The above is the detailed content of Methods to improve cache efficiency in Go language. For more information, please follow other related articles on the PHP Chinese website!
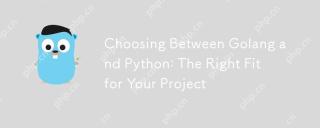
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
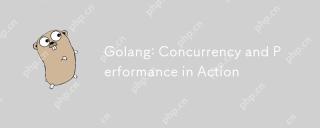
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
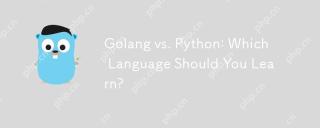
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
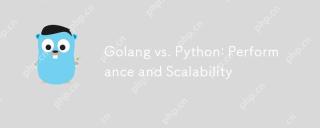
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
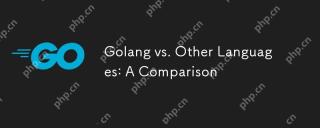
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
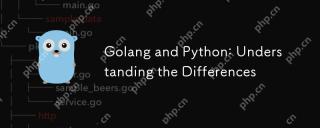
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
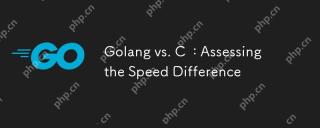
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
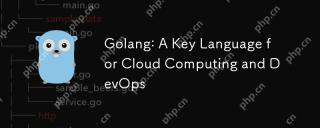
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
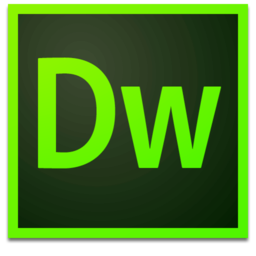
Dreamweaver Mac version
Visual web development tools
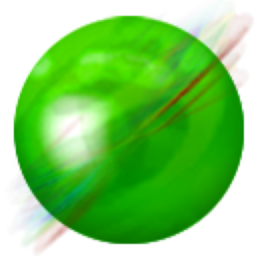
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
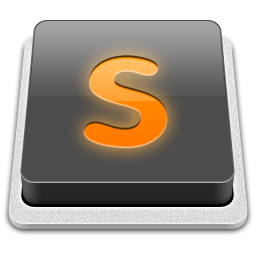
SublimeText3 Mac version
God-level code editing software (SublimeText3)