The public keyword in PHP is an access modifier in object-oriented programming. It is used to indicate that members (properties and methods) are public and can be accessed from anywhere in the class. This article will introduce the usage and examples of the public keyword in PHP.
- Public modification of attributes
In PHP, we can use the public keyword to modify the attributes of a class so that it can be accessed from anywhere in the class. For example, we define a Person class and use the public keyword to modify its attribute $name:
class Person { public $name; public function __construct($name) { $this->name = $name; } public function greet() { echo "Hello, my name is " . $this->name; } } $person = new Person("John"); $person->name = "Mike"; // 修改属性值 echo $person->name; // 访问属性值
In the above example, we can directly access and modify the $name attribute of the $person object because it is public Keyword modification. By calling the $person->greet() method, we can output "Hello, my name is Mike".
- Public modification of methods
In addition to attributes, we can also use the public keyword to modify class methods. Methods modified by public can be directly called by external code of the class. For example, we continue to use the above Person class and add a public modified sayHello method:
class Person { public $name; // 构造方法 // 属性$name public function greet() { echo "Hello, my name is " . $this->name; } public function sayHello($to) { echo "Hello, " . $to; } } $person = new Person("John"); $person->sayHello("Mike"); // 调用public方法
In the above example, we can call the Person class through $person->sayHello("Mike") The public modified sayHello method in the method outputs "Hello, Mike".
- Inheritance of public modifiers
When a class inherits another class, the access modifiers of the inherited properties and methods follow the inheritance. If inherited properties or methods are public, they will also be public in subclasses. For example, we define a Student class that inherits from the Person class:
class Student extends Person { public $grade; public function study() { echo "I am studying in grade " . $this->grade; } } $student = new Student("Alice"); $student->name = "Bob"; // 从父类继承的public属性 echo $student->name; // 从父类继承的public属性 $student->study(); // 调用从父类继承的public方法
In the above example, the Student class inherits the Person class, and can directly access and modify the public attribute $name inherited from the parent class, and call it from The public method greet inherited from the parent class.
Summary:
In PHP, the public keyword is used to define the access permissions of the properties and methods of a class as public and can be accessed from anywhere in the class. Properties and methods modified by public can be directly called and modified in the internal and external code of the class. In addition, the inheritance mechanism of the public modifier allows subclasses to inherit and use the public properties and methods in the parent class. By rationally using the public keyword, we can better encapsulate the code and improve the maintainability and reusability of the code.
The above is the usage and examples of the public keyword in PHP. I hope it will be helpful to readers in understanding and applying the public keyword.
The above is the detailed content of Usage and examples of public keyword in PHP. For more information, please follow other related articles on the PHP Chinese website!
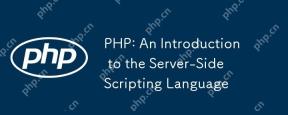
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
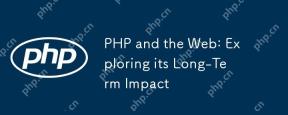
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
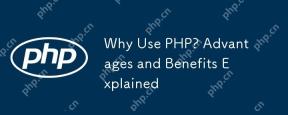
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
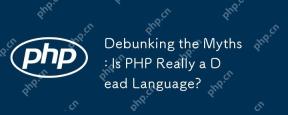
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
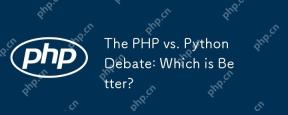
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
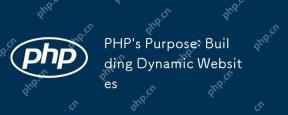
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
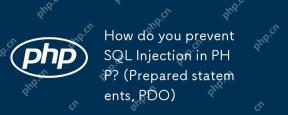
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
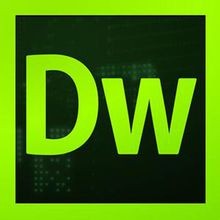
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
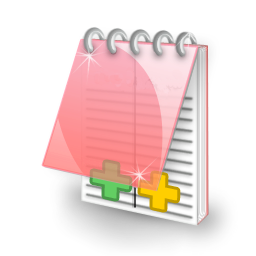
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.