


How to implement real-time stream processing using PHP and Apache Kafka
Apache Kafka is a high-throughput, low-latency distributed publish/subscribe messaging system. It is widely used in the architecture of real-time stream processing systems to process high-frequency, large-capacity data streams. This article will introduce how to use PHP and Apache Kafka to implement real-time stream processing.
- Installing Apache Kafka
Before we start using Apache Kafka, we need to install it first. You can download and install Apache Kafka from the official website, or use some open source installation scripts. Here, we will use the binary version provided by Apache Kafka.
- Create a Kafka producer
Next, we will create a Kafka producer to push data to the Kafka cluster. In PHP, we can use the kafka-php extension to achieve this.
First, we need to download and compile the kafka-php extension. Detailed installation instructions can be found on kafka-php’s GitHub page. After the installation is complete, we can use the kafka-php extension in our PHP code.
The following is an example that demonstrates how to create a Kafka producer and send messages to a topic:
<?php require_once('KafkaProducer.php'); $producer = new KafkaProducer('localhost:9092'); $producer->send([ [ 'topic' => 'example-topic', 'value' => 'Hello, Kafka!', 'key' => 'key1' ] ]); ?>
In the above code, we first create a KafkaProducer object, specify The address of the Kafka cluster. Then, we sent a message to the topic (example-topic) through the send method.
The message sent is an array, which contains the subject, content and key of the message. Keys can be used to group messages so that the Kafka cluster can distribute messages with the same key into the same partition.
- Create a Kafka consumer
Next, we will create a Kafka consumer to consume data from the Kafka cluster. Similarly, in PHP, we can use kafka-php extension to achieve this.
<?php require_once('KafkaConsumer.php'); $consumer = new KafkaConsumer('localhost:9092', 'example-group', ['example-topic']); $consumer->consume(function($message) { echo $message->payload . " "; }); ?>
In the above code, we first create a KafkaConsumer object, specifying the address of the Kafka cluster, the name of the consumer group (group), and the topic to be consumed. Then, we start consuming data through the consume method.
Theconsume method accepts a callback function as a parameter for processing messages received from the Kafka cluster. In the callback function, we can access the content of the message (payload).
Note that we specified the name of the consumer group when creating the Kafka consumer. Consumer groups are a key concept in Kafka and are used to distribute messages into partitions. Consumers with the same consumer group name will consume the same topic together, and Kafka will automatically distribute messages among them. The purpose of the consumer group is to ensure that each message is consumed only once.
- Real-time stream processing
Now, we can combine the above two examples to achieve real-time stream processing. We can create a Kafka producer and send messages to the topic periodically. We can then create a Kafka consumer that processes the messages received from the topic in a callback function.
The following is an example demonstrating real-time stream processing:
<?php require_once('KafkaProducer.php'); require_once('KafkaConsumer.php'); $producer = new KafkaProducer('localhost:9092'); $consumer = new KafkaConsumer('localhost:9092', 'example-group', ['example-topic']); while (true) { $producer->send([ [ 'topic' => 'example-topic', 'value' => rand(0, 10), 'key' => 'key1' ] ]); $consumer->consume(function($message) { $value = $message->payload; echo "Received $value "; }); sleep(1); } ?>
In the above code, we first create a Kafka producer and a Kafka consumer. We then enter a loop that periodically sends a random number to the topic and consumes messages from the topic. In the consumer callback function, we print the received value to the console.
What is demonstrated here is a simple real-time stream processing process. In reality, real-time stream processing systems may be more complex, may have multiple producers and consumers, and may have multiple topics and partitions. But in any case, using PHP and Apache Kafka can easily build a real-time stream processing system and process high-frequency, large-volume data streams.
The above is the detailed content of How to implement real-time stream processing using PHP and Apache Kafka. For more information, please follow other related articles on the PHP Chinese website!
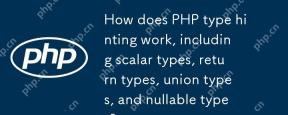
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
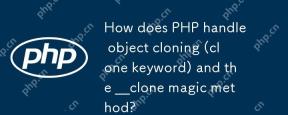
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
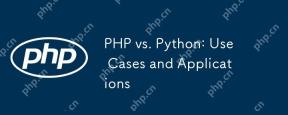
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
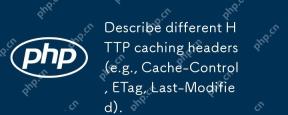
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
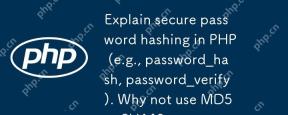
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
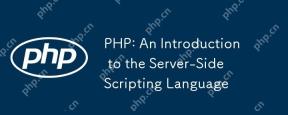
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
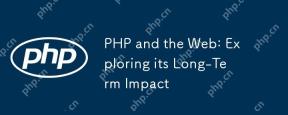
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
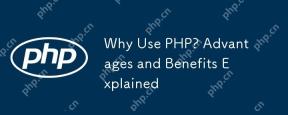
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
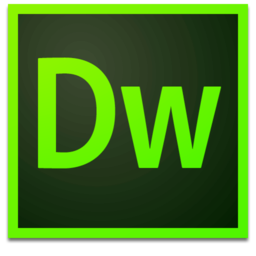
Dreamweaver Mac version
Visual web development tools
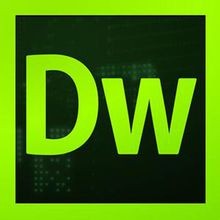
Dreamweaver CS6
Visual web development tools