How to implement theme switching and style theme management under Vue?
Vue is a modern JavaScript framework that allows front-end developers to build web applications in a componentized manner. Vue provides flexible APIs and tools for designing reusable and modular components, as well as the ability to handle dynamic data binding and responsive UI. In this article, we will discuss some basic Vue tips and methods to implement theme switching and style theme management.
- Implementing theme switching
The theme of a Vue application is the visual appearance of the application. An application's theme usually consists of colors, fonts, and other visual attributes. Vue allows switching between different themes on demand within the application. The following are some steps to achieve theme switching:
(1) Define theme style
First, we need to create a style for the theme. These styles can be defined in CSS files or used as style objects in Vue components. For example, here is the blue theme definition for the application:
.theme-blue { --primary-color: blue; }
This style gives the main color of the application. In this example, we set the --primary-color variable to blue. When we apply this style, all elements in the application that use this variable will turn blue.
(2) Switching themes in the application
In order to switch the theme in the application, we need to define a method that sets the style of the application according to the theme selected by the user. The following is a simple method:
changeTheme(theme) { // 获取所有使用主题的DOM元素 let elements = document.querySelectorAll('[data-theme]') // 更新样式 elements.forEach(element => { element.dataset.theme = theme }) }
In this method, we first use the querySelectorAll method to get all the elements using the theme. We can also see in the HTML examples below that they all use a "data-theme" attribute to identify the theme they use. We then loop through these elements and set their "data-theme" attribute to the user-selected theme.
(3) Using themes in Vue components
In order to use themes in Vue components, we need to use Vue's data binding function. Specifically, we can use the v-bind directive to bind the element's "data-theme" attribute to the Vue component's data. For example:
<template> <div v-bind:data-theme="theme" class="header">Header</div> <div v-bind:data-theme="theme" class="content">Content</div> <div v-bind:data-theme="theme" class="footer">Footer</div> </template> <script> export default { data() { return { theme: 'default' } } } </script>
In this Vue component, we use the v-bind directive to bind the element's "data-theme" attribute to the component's theme data. When we update the theme data, the elements bound to this data will automatically update the theme.
- Implementing style theme management
In addition to switching themes, we can also implement some other style theme management functions in the Vue application. For example, we can:
(1) Define multiple themes
We can define multiple themes and let users choose the style they like. For example, we can define a blue theme and a green theme. Users can choose their preferred theme within the app.
(2) Storing user selection
We can use the browser's local storage technology, such as localStorage, to store the theme selected by the user locally. This way, the next time the user visits the application, they will have the previously selected theme.
(3) Apply default theme
When a user accesses the application for the first time, we can apply a default theme to the application. This way, even if the user doesn't choose a theme, the application will have a default look and feel.
(4) Support multiple style attributes
In addition to color, we can also define other style attributes, such as font, border and shadow. These properties can be defined in different themes.
- Sample code
The following is a complete sample code that demonstrates the functionality of implementing various style theme management in a Vue application.
<template> <div v-bind:data-theme="theme" class="container"> <h1 id="Theme-Switcher">Theme Switcher</h1> <div> <label> <input type="radio" v-model="theme" value="default"> Default </label> <label> <input type="radio" v-model="theme" value="blue"> Blue </label> <label> <input type="radio" v-model="theme" value="green"> Green </label> </div> </div> </template> <script> export default { data() { return { theme: localStorage.getItem('theme') || 'default' } }, mounted() { // 应用默认主题 document.documentElement.setAttribute('data-theme', this.theme) }, methods: { changeTheme(theme) { // 获取所有使用主题的DOM元素 let elements = document.querySelectorAll('[data-theme]') // 更新样式 elements.forEach(element => { element.dataset.theme = theme }) // 存储用户选择 localStorage.setItem('theme', theme) } } } </script> <style> .container { --primary-color: black; --background-color: white; } [data-theme="default"] { --primary-color: black; --background-color: white; } [data-theme="blue"] { --primary-color: blue; --background-color: #f5f5f5; } [data-theme="green"] { --primary-color: green; --background-color: #f5f5f5; } h1 { color: var(--primary-color); } label { margin-right: 10px; } input:checked + span { color: var(--primary-color); font-weight: bold; } </style>
In this code example, we define a Vue component that contains a theme switcher. We use the component's data attribute theme to store the user-selected theme, and use the v-bind directive to bind the component's data attribute theme to all DOM elements that use the data attribute "data-theme".
The component's method changeTheme gets all elements from all DOM elements that use the data attribute "data-theme" and updates their data attribute "data-theme" when the user selects a theme. This method also uses localStorage to store the user-selected theme in the user's local browser storage.
Finally, we defined three different themes using CSS variables. Within these themes, we define two CSS variables that control the appearance of the application: --primary-color and --background-color. We also defined some basic CSS styles for the app’s title and theme switcher.
Conclusion
Implementing theme switching and style theme management in Vue applications is a useful feature that allows users to customize the appearance of the application according to their preferences. In this article, we discussed how to use Vue’s data binding capabilities and CSS variables to implement theme switching and style theme management. Of course, there are more methods and techniques to achieve these functions, but the methods given here are a good starting point.
The above is the detailed content of How to implement theme switching and style theme management under Vue?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
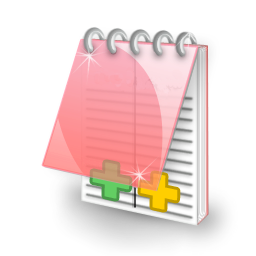
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool